Real-Time Auction Platform using Node and Express.js
Last Updated :
04 Apr, 2024
The project is a Real-Time Auction Platform developed using Node.js Express.js and MongoDB database for storing details where users can browse different categories of products, view ongoing auctions, bid on items, and manage their accounts. The platform also allows sellers to list their products for auction.
Output Preview: Let us have a look at how the final output will look like.
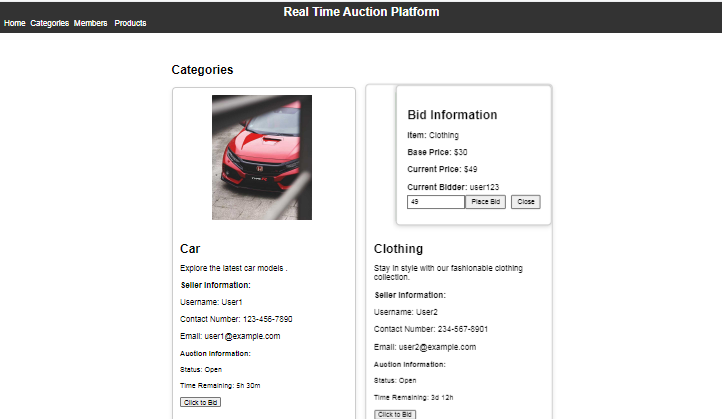
Prerequisites:
Approach to create Real-Time Auction Platform:
- Backend developed using Node.js and Express.js to handle HTTP requests and interact with the database.
- MongoDB used to store user information, product listings, and auction data.
- Frontend: Utilizes React.js to create a dynamic user interface for browsing categories, viewing item details, and placing bids.
- Sellers can list products for auction, specifying details like name, description, price, starting date, and ending date.
Steps to create the Real-Time Auction Platform:
Step 1: Create the project folder using the following command.
mkdir auction-platform
cd auction-platform
Step 2: Create a new project directory and navigate to your project directory.
mkdir server
cd server
Step 3: Run the following command to initialize a new NodeJS project.
npm init -y
Step 4: Install the required the packages in your server using the following command.
npm install express body-parser cors mongoose
Project Structure(backend):
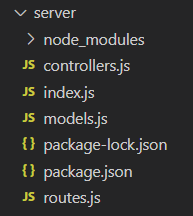
The updated Dependencies in package.json :
"dependencies": {
"axios": "^1.6.7",
"body-parser": "^1.20.2",
"cors": "^2.8.5",
"express": "^4.18.3",
"mongoose": "^8.2.1",
}
Code Example: Create the required files as shown in the folder structure and add the following codes.
Javascript
//index.js
const express = require('express');
const bodyParser = require('body-parser');
const mongoose = require('mongoose');
const routes = require('./routes');
const app = express();
const PORT = process.env.PORT || 3000;
mongoose.connect('Your MongoDB URI', {
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log('MongoDB connected'))
.catch(err => console.error('MongoDB connection error:', err));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
app.use('/', routes);
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
JavaScript
//models.js
const mongoose = require('mongoose');
const User = mongoose.model('User', new mongoose.Schema({
username: String,
contactNumber: String,
email: String,
}));
const ItemCategory = mongoose.model('ItemCategory', new mongoose.Schema({
name: String,
timeRemaining: String,
startingTime: Date,
state: String,
}));
const Product = mongoose.model('Product', new mongoose.Schema({
name: String,
description: String,
price: Number,
}));
module.exports = { User, ItemCategory, Product };
JavaScript
//routes.js
const express = require('express');
const router = express.Router();
const {
getAllUsers,
createUser,
getAllItemCategories,
createItemCategory,
getAllProducts,
createProduct,
} = require('./controllers');
router.get('/users', getAllUsers);
router.post('/users', createUser);
router.get('/itemCategories', getAllItemCategories);
router.post('/itemCategories', createItemCategory);
router.get('/products', getAllProducts);
router.post('/products', createProduct);
module.exports = router;
JavaScript
//controllers.js
const { User, ItemCategory, Product } = require('./models');
exports.getAllUsers = async (req, res) => {
try {
const users = await User.find();
res.json(users);
} catch (err) {
res.status(500).json({ error: err.message });
}
};
exports.createUser = async (req, res) => {
try {
const user = await User.create(req.body);
res.status(201).json(user);
} catch (err) {
res.status(400).json({ error: err.message });
}
};
exports.getAllItemCategories = async (req, res) => {
try {
const itemCategories = await ItemCategory.find();
res.json(itemCategories);
} catch (err) {
res.status(500).json({ error: err.message });
}
};
exports.createItemCategory = async (req, res) => {
try {
const itemCategory = await ItemCategory.create(req.body);
res.status(201).json(itemCategory);
} catch (err) {
res.status(400).json({ error: err.message });
}
};
exports.getAllProducts = async (req, res) => {
try {
const products = await Product.find();
res.json(products);
} catch (err) {
res.status(500).json({ error: err.message });
}
};
exports.createProduct = async (req, res) => {
try {
const product = await Product.create(req.body);
res.status(201).json(product);
} catch (err) {
res.status(400).json({ error: err.message });
}
};
Step 5: Start the Server
In the terminal, navigate to the server folder and run the following command to start the server:
node index.js
Step 6: Go to root directory and create the React application using the following command.
npx create-react-app client
cd client
Step 7: Install the required packages in your application using the following command.
npm i react-router-dom axios styled-components
Project Structure(frontend):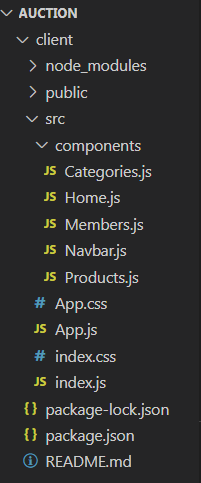
The updated Dependencies in package.json file will look like:
"dependencies": {
"axios": "^1.6.7",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.3",
"react-scripts": "5.0.1",
"styled-components": "^6.1.8",
"web-vitals": "^2.1.4"
}
Code Example: Create the following files as shown in folder structure and add the following codes.
CSS
/* src/App.css */
body {
font-family: 'Arial', sans-serif;
margin: 0;
padding: 0;
}
nav {
background-color: #333;
color: white;
padding: 10px;
}
nav h1 {
margin: 0;
}
nav a {
color: white;
margin-right: 10px;
text-decoration: none;
}
.container {
max-width: 800px;
margin: 20px auto;
}
ul {
list-style: none;
padding: 0;
}
li {
margin-bottom: 10px;
}
.members-container {
background-color: #f9f9f9;
padding: 20px;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.members-list {
padding-left: 20px;
}
.members-item {
font-size: 16px;
border-bottom: 1px solid #ddd;
padding: 10px 0;
}
.members-item:last-child {
border-bottom: none;
}
.categories-container {
max-width: 800px;
margin: auto;
padding: 20px;
}
.categories-list {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
.category-item {
width: 48%;
margin-bottom: 20px;
border: 1px solid #ccc;
border-radius: 8px;
overflow: hidden;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease-in-out;
}
.category-item:hover {
transform: scale(1.02);
}
.category-image img {
width: 100%;
height: 200px;
object-fit: cover;
}
.category-details {
padding: 15px;
}
h3 {
margin-bottom: 10px;
font-size: 1.5rem;
}
.category-description {
margin-bottom: 15px;
}
.category-seller-info {
margin-bottom: 15px;
}
.category-auction-info {
font-size: 0.9rem;
}
.bid-modal {
position: absolute;
top: 0;
right: 0;
background: #fff;
padding: 20px;
border: 1px solid #ccc;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
z-index: 100;
}
.bid-modal h3 {
margin-bottom: 10px;
}
.bid-modal p {
margin-bottom: 5px;
}
.bid-modal input {
margin-bottom: 10px;
padding: 5px;
width: 100px;
}
.bid-modal button {
padding: 5px 10px;
margin-right: 10px;
cursor: pointer;
}
.bid-modal button:last-child {
margin-right: 0;
}
Javascript
// src/App.js
import React from 'react';
import { BrowserRouter as Router, Route, NavLink, Routes } from 'react-router-dom';
import Home from './components/Home';
import Categories from './components/Categories';
import Members from './components/Members';
import Products from './components/Products';
import './App.css';
function App() {
return (
<Router>
<nav>
<h1><center>Real Time Auction Platform</center></h1>
<div>
<NavLink to="/" activeClassName="active">
Home
</NavLink>
<NavLink to="/categories" activeClassName="active">
Categories
</NavLink>
<NavLink to="/members" activeClassName="active">
Members
</NavLink>
<NavLink to="/products" activeClassName="active"> { }
Products
</NavLink>
</div>
</nav>
<div className="container">
<Routes>
<Route path="/" element={<Home />} />
<Route path="/categories" element={<Categories />} />
<Route path="/members" element={<Members />} />
<Route path="/products" element={<Products />} /> { }
</Routes>
</div>
</Router>
);
}
export default App;
Javascript
//components/Home.js
import React from 'react';
function Home() {
const userData = {
username: 'User 123',
};
const username = userData ? userData.username : 'Unknown';
return (
<div>
<h2>Welcome to the Real-Time Auction Platform</h2>
<p>Hello, {username}!</p>
</div>
);
}
export default Home;
Javascript
//components/Navbar.js
import React from 'react';
import { Link } from 'react-router-dom';
const Navbar = () => {
return (
<nav>
<Link to="/">Real-Time Auction Platform</Link>
<div>
<Link to="/">Home</Link>
<Link to="/categories">Categories</Link>
<Link to="/members">Members</Link>
<Link to="/products">Products</Link>
</div>
</nav>
);
};
export default Navbar;
Javascript
// src/components/Members.js
import React, { useState } from "react";
import "../App.css";
function Members() {
const hardcodedMembers = [
{
id: 1,
username: "User123",
contactNumber: "9898989898",
email: "user123@gmail.com",
},
{
id: 2,
username: "User2",
contactNumber: "234-567-8901",
email: "user2@example.com",
},
{
id: 3,
username: "User3",
contactNumber: "345-678-9012",
email: "user3@example.com",
},
{
id: 4,
username: "User4",
contactNumber: "456-789-0123",
email: "user4@example.com",
},
{
id: 5,
username: "User5",
contactNumber: "567-890-1234",
email: "user5@example.com",
},
{
id: 6,
username: "User6",
contactNumber: "678-901-2345",
email: "user6@example.com",
},
{
id: 7,
username: "User7",
contactNumber: "789-012-3456",
email: "user7@example.com",
},
{
id: 8,
username: "User8",
contactNumber: "890-123-4567",
email: "user8@example.com",
},
{
id: 9,
username: "User9",
contactNumber: "901-234-5678",
email: "user9@example.com",
},
{
id: 10,
username: "User10",
contactNumber: "012-345-6789",
email: "user10@example.com",
},
];
const [members, setMembers] = useState(hardcodedMembers);
return (
<div className="members-container">
<h2>Members</h2>
<ul className="members-list">
{members.map((member) => (
<li key={member.id} className="members-item">
<strong>{member.username}</strong> - {member.contactNumber} -{" "}
{member.email}
</li>
))}
</ul>
</div>
);
}
export default Members;
Javascript
//components/Categories.js
import React, { useState } from "react";
import "../App.css";
function Categories() {
const [biddingItem, setBiddingItem] = useState(null);
const [bidAmount, setBidAmount] = useState(0);
const getUserByIndex = (index) => {
const users = [
{
username: "User1",
contactNumber: "123-456-7890",
email: "user1@example.com",
},
{
username: "User2",
contactNumber: "234-567-8901",
email: "user2@example.com",
},
{
username: "User3",
contactNumber: "345-678-9012",
email: "user3@example.com",
},
{
username: "User4",
contactNumber: "456-789-0123",
email: "user4@example.com",
},
{
username: "User5",
contactNumber: "567-890-1234",
email: "user5@example.com",
},
];
return users[index];
};
const categoriesData = [
{
id: 1,
name: "Car",
description: "Explore the latest car models .",
imageUrl:
"https://media.geeksforgeeks.org/wp-content/uploads/20240122184958/images2.jpg",
sellerInfo: getUserByIndex(0),
auctionInfo: {
status: "Open",
timeRemaining: "5h 30m",
basePrice: 50,
currentPrice: 0,
currentBidder: null,
},
},
{
id: 2,
name: "Clothing",
description: "Stay in style with our fashionable clothing collection.",
imageUrl:
"https://media.geeksforgeeks.org/wp-content/uploads/20230407153938/gfg-hoodie.jpg",
sellerInfo: getUserByIndex(1),
auctionInfo: {
status: "Open",
timeRemaining: "3d 12h",
basePrice: 30,
currentPrice: 0,
currentBidder: null,
},
},
{
id: 3,
name: "Books",
description:
"Immerse yourself in the world of literature with our diverse book collection.",
imageUrl:
"https://media.geeksforgeeks.org/wp-content/uploads/20240110011929/glasses-1052010_640.jpg",
sellerInfo: getUserByIndex(2),
auctionInfo: {
status: "Open",
timeRemaining: "1w 1d",
basePrice: 20,
currentPrice: 0,
currentBidder: null,
},
},
{
id: 4,
name: "Honda second hand vehicle",
description: "Best working condition .",
imageUrl:
"https://media.geeksforgeeks.org/wp-content/uploads/20240122182422/images1.jpg",
sellerInfo: getUserByIndex(3),
auctionInfo: {
status: "Open",
timeRemaining: "2d 8h",
basePrice: 80,
currentPrice: 0,
currentBidder: null,
},
},
{
id: 5,
name: "Sports wear",
description:
"Fuel your active lifestyle with our high-quality sports tshirt.",
imageUrl:
"https://media.geeksforgeeks.org/wp-content/uploads/20230407153931/gfg-tshirts.jpg",
sellerInfo: getUserByIndex(4),
auctionInfo: {
status: "Open",
timeRemaining: "6h 20m",
basePrice: 40,
currentPrice: 0,
currentBidder: null,
},
},
];
const handleBidClick = (item) => {
setBiddingItem(item);
setBidAmount(
item.auctionInfo.basePrice + Math.floor(Math.random() * 50) + 1
);
};
const handlePlaceBid = () => {
setBiddingItem((prevItem) => ({
...prevItem,
auctionInfo: {
...prevItem.auctionInfo,
currentPrice: bidAmount,
currentBidder: "user123",
},
}));
};
return (
<div className="categories-container">
<h2>Categories</h2>
<div className="categories-list">
{categoriesData.map((category) => (
<div key={category.id} className="category-item">
<div className="category-image">
<img src={category.imageUrl} alt={category.name} />
</div>
<div className="category-details">
<h3>{category.name}</h3>
<p className="category-description">{category.description}</p>
<div className="category-seller-info">
<p>
<strong>Seller Information:</strong>
</p>
<p>Username: {category.sellerInfo.username}</p>
<p>Contact Number: {category.sellerInfo.contactNumber}</p>
<p>Email: {category.sellerInfo.email}</p>
</div>
<div className="category-auction-info">
<p>
<strong>Auction Information:</strong>
</p>
<p>Status: {category.auctionInfo.status}</p>
<p>Time Remaining: {category.auctionInfo.timeRemaining}</p>
{category.auctionInfo.status === "Open" && (
<>
<button onClick={() => handleBidClick(category)}>
Click to Bid
</button>
</>
)}
</div>
{biddingItem && biddingItem.id === category.id && (
<div className="bid-modal">
<h3>Bid Information</h3>
<p>
<strong>Item:</strong> {biddingItem.name}
</p>
<p>
<strong>Base Price:</strong> $
{biddingItem.auctionInfo.basePrice}
</p>
<p>
<strong>Current Price:</strong> $
{biddingItem.auctionInfo.currentPrice}
</p>
<p>
<strong>Current Bidder:</strong>{" "}
{biddingItem.auctionInfo.currentBidder || "None"}
</p>
<input
type="number"
value={bidAmount}
onChange={(e) => setBidAmount(e.target.value)}
min={biddingItem.auctionInfo.basePrice + 1}
/>
<button onClick={handlePlaceBid}>Place Bid</button>
<button onClick={() => setBiddingItem(null)}>Close</button>
</div>
)}
</div>
</div>
))}
</div>
</div>
);
}
export default Categories;
Javascript
//components/Products.js
import React, { useState } from "react";
function Products() {
const defaultSellerInfo = {
username: "User 123",
contactNumber: "9898989898",
email: "user123@gmail.com",
};
const defaultProduct = {
name: "",
description: "",
price: 0,
sellerInfo: defaultSellerInfo,
startingDate: "",
endingDate: "",
image: null,
};
const [products, setProducts] = useState([]);
const [newProduct, setNewProduct] = useState(defaultProduct);
const [editProduct, setEditProduct] = useState(null);
const [editName, setEditName] = useState("");
const [editDescription, setEditDescription] = useState("");
const [editPrice, setEditPrice] = useState("");
const [editStartingDate, setEditStartingDate] = useState("");
const [editEndingDate, setEditEndingDate] = useState("");
const handleInputChange = (e) => {
const { name, value } = e.target;
switch (name) {
case "name":
setEditName(value);
break;
case "description":
setEditDescription(value);
break;
case "price":
setEditPrice(value);
break;
case "startingDate":
setEditStartingDate(value);
break;
case "endingDate":
setEditEndingDate(value);
break;
default:
break;
}
};
const handleImageChange = (e) => {
setEditProduct({
...editProduct,
image: e.target.files[0],
});
};
const addProduct = () => {
setProducts([...products, newProduct]);
setNewProduct(defaultProduct);
};
const deleteProduct = (index) => {
setProducts(products.filter((_, i) => i !== index));
};
const updateProduct = (index) => {
const updatedProducts = [...products];
const updatedProduct = {
...editProduct,
name: editName,
description: editDescription,
price: editPrice,
startingDate: editStartingDate,
endingDate: editEndingDate,
};
updatedProducts[index] = updatedProduct;
setProducts(updatedProducts);
setEditProduct(null);
// Clear edit fields
setEditName("");
setEditDescription("");
setEditPrice("");
setEditStartingDate("");
setEditEndingDate("");
};
return (
<div
style={{
fontFamily: "Arial, sans-serif",
maxWidth: "800px",
margin: "0 auto",
}}
>
<h2 style={{ textAlign: "center", marginBottom: "20px" }}>Products</h2>
<div>
<h3>Add New Product</h3>
<div style={{ marginBottom: "10px" }}>
<input
type="text"
name="name"
placeholder="Name"
value={newProduct.name}
onChange={(e) =>
setNewProduct({ ...newProduct, name: e.target.value })
}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="text"
name="description"
placeholder="Description"
value={newProduct.description}
onChange={(e) =>
setNewProduct({ ...newProduct, description: e.target.value })
}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="number"
name="price"
placeholder="Price"
value={newProduct.price}
onChange={(e) =>
setNewProduct({ ...newProduct, price: e.target.value })
}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="text"
name="startingDate"
placeholder="Starting Date"
value={newProduct.startingDate}
onChange={(e) =>
setNewProduct({ ...newProduct, startingDate: e.target.value })
}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="text"
name="endingDate"
placeholder="Ending Date"
value={newProduct.endingDate}
onChange={(e) =>
setNewProduct({ ...newProduct, endingDate: e.target.value })
}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="file"
name="image"
onChange={(e) =>
setNewProduct({ ...newProduct, image: e.target.files[0] })
}
style={{ padding: "5px" }}
/>
<button
onClick={addProduct}
style={{ padding: "5px 10px", marginLeft: "10px" }}
>
Add Product
</button>
</div>
</div>
<div>
<h3>Products List</h3>
<ul style={{ listStyleType: "none", padding: 0 }}>
{products.map((product, index) => (
<li
key={index}
style={{
marginBottom: "20px",
border: "1px solid #ccc",
padding: "10px",
borderRadius: "5px",
boxShadow: "0 0 5px rgba(0, 0, 0, 0.1)",
}}
>
<div
style={{
display: "flex",
alignItems: "center",
marginBottom: "10px",
}}
>
{product.image && (
<img
src={URL.createObjectURL(product.image)}
alt="Product"
style={{
marginRight: "10px",
width: "100px",
height: "auto",
borderRadius: "5px",
}}
/>
)}
<div>
<strong>Name:</strong> {product.name}
<br />
<strong>Description:</strong> {product.description}
<br />
<strong>Price:</strong> ${product.price}
<br />
<strong>Starting Date:</strong> {product.startingDate}
<br />
<strong>Ending Date:</strong> {product.endingDate}
<br />
<strong>Seller Info:</strong> <br />
Username: {product.sellerInfo.username}
<br />
Contact Number: {product.sellerInfo.contactNumber}
<br />
Email: {product.sellerInfo.email}
</div>
</div>
<div>
{editProduct === product ? (
<>
<input
type="text"
name="name"
value={editName}
onChange={handleInputChange}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="text"
name="description"
value={editDescription}
onChange={handleInputChange}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="number"
name="price"
value={editPrice}
onChange={handleInputChange}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="text"
name="startingDate"
value={editStartingDate}
onChange={handleInputChange}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="text"
name="endingDate"
value={editEndingDate}
onChange={handleInputChange}
style={{ marginRight: "10px", padding: "5px" }}
/>
<input
type="file"
name="image"
onChange={handleImageChange}
style={{ padding: "5px" }}
/>
<button
onClick={() => updateProduct(index)}
style={{ padding: "5px 10px", marginLeft: "10px" }}
>
Update
</button>
<button
onClick={() => setEditProduct(null)}
style={{ padding: "5px 10px", marginLeft: "10px" }}
>
Cancel
</button>
</>
) : (
<>
<button
onClick={() => {
setEditProduct(product);
setEditName(product.name);
setEditDescription(product.description);
setEditPrice(product.price);
setEditStartingDate(product.startingDate);
setEditEndingDate(product.endingDate);
}}
>
Edit
</button>
<button
onClick={() => deleteProduct(index)}
style={{ marginLeft: "10px" }}
>
Delete
</button>
</>
)}
</div>
</li>
))}
</ul>
</div>
</div>
);
}
export default Products;
Start your application using the following command.
npm start
Output:
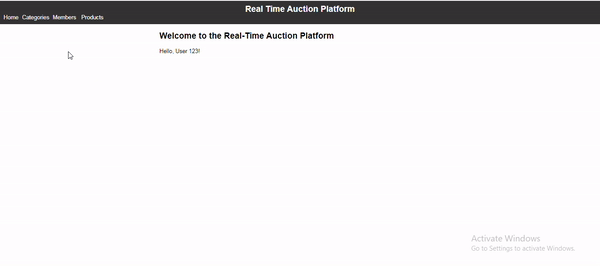
Share your thoughts in the comments
Please Login to comment...