Fundraiser Platform using MERN
Last Updated :
28 Dec, 2023
A fundraising application, often referred to as a “fundraiser application” or “crowdfunding platform,” is a software or web-based platform designed to facilitate the collection of funds for a specific cause, project, or campaign. These applications provide a digital space where individuals, organizations, or businesses can create fundraising campaigns and seek financial support from a wider audience, typically through online donations.
Preview of final output: Let us have a look at how the final application will look like.
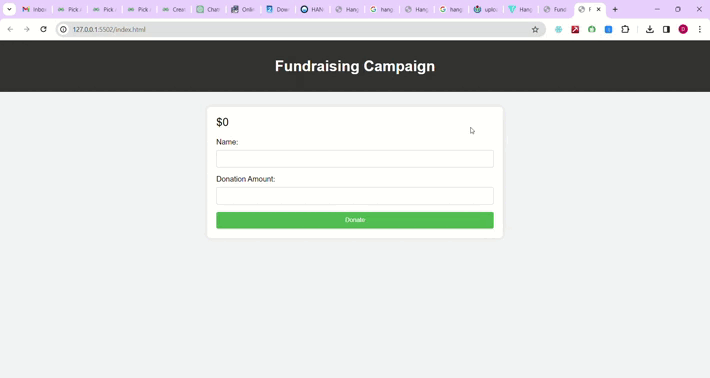
Output
Technologies Used:
Project Structure:
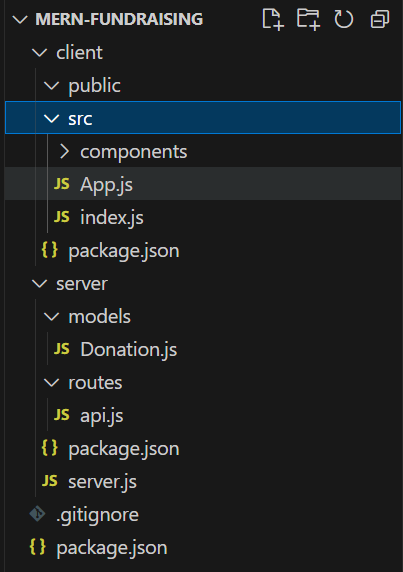
Project Structure
Approach:
- Project Initialization:
- Create a new folder for the project and navigate to it.
- Initialize a new Node.js project using npm init -y.
- Install necessary dependencies: express for the server and mongoose for MongoDB connection.
- Server Setup:
- Create a server folder.
- Inside server, create a server.js file.
- Set up an Express server in server.js.
- Connect to MongoDB using Mongoose.
- Set up middleware for parsing JSON.
- Create API routes to handle fundraising operations (create, list donations).
- Models:
- Inside server, create a models folder.
- Define a Donation model using Mongoose to represent donation data.
- API Routes:
- Inside server, create a routes folder.
- Create an api.js file within the routes folder to handle API routes.
- Implement routes for creating and fetching donations.
- Run the Backend:
- Start the Express server using node server/server.js.
Steps to Create the Backend Node App:
Step 1: Create a new React App
npx create-react-app <name of project>
Step 2: Navigate to the Project folder using:
cd <name of project>
Step 3: Installing Required Packages.
npm install express mongoose
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.17.1",
"mongoose": "^5.13.2"
},
Example: Below is the code for the backend server in nodejs
Javascript
const express = require( 'express' );
const mongoose = require( 'mongoose' );
const bodyParser = require( 'body-parser' );
const app = express();
const PORT = process.env.PORT || 5000;
useNewUrlParser: true ,
useUnifiedTopology: true ,
});
const Donation = mongoose.model( 'Donation' , {
amount: Number,
description: String,
});
app.use(bodyParser.json());
app.post( '/api/donations' , async (req, res) => {
try {
const { amount, description } = req.body;
const newDonation = new Donation({ amount, description });
await newDonation.save();
res.status(201).json(newDonation);
} catch (error) {
console.error(error);
res.status(500).send( 'Internal Server Error' );
}
});
app.get( '/api/donations' , async (req, res) => {
try {
const donations = await Donation.find();
res.json(donations);
} catch (error) {
console.error(error);
res.status(500).send( 'Internal Server Error' );
}
});
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
Start the Backend Server using the following command.
node server.js
Steps to Create the Frontend React App:
Step 1: Create a new React App
npx create-react-app <name of project>
Step 2: Navigate to the Project folder using:
cd <name of project>
The updated dependencies in package.json file of frontend will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example: Below is the code for the frontend client in reactjs
Javascript
import React, {useState,useEffect} from 'react' ;
import './App.css' ;
function App() {
const [donations, setDonations] = useState([]);
const [amount, setAmount] = useState( '' );
const [description, setDescription] = useState( '' );
useEffect(() => {
fetchDonations();
}, []);
const fetchDonations = async () => {
try {
const response =
await fetch('http:
const data = await response.json();
setDonations(data);
} catch (error) {
console.error('Error fetching donations: ', error);
}
};
const handleDonate = async () => {
try {
// Make a POST request to your server' s API endpoint
const response =
method: 'POST' ,
headers: {
'Content-Type' : 'application/json' ,
},
body: JSON.stringify(
{
amount: parseFloat(amount),
description
}),
});
if (response.ok) {
setDonations(
[...donations,
await response.json()]);
setAmount( '' );
setDescription( '' );
} else {
console.error( 'Error donating:' , response.statusText);
}
} catch (error) {
console.error( 'Error donating:' , error);
}
};
return (
<div className= "App" >
<h1>MERN Fundraising App</h1>
<div>
<label>
Amount:
<input type= "number"
value={amount}
onChange={
(e) =>
setAmount(e.target.value)
} />
</label>
<br />
<label>
Description:
<input type= "text" value={description}
onChange={
(e) =>
setDescription(e.target.value)
} />
</label>
<br />
<button onClick={handleDonate}>Donate</button>
</div>
<div>
<h2>Donations:</h2>
<ul>
{donations.map((donation) => (
<li key={donation._id}>
Amount: {donation.amount},
Description: {donation.description}
</li>
))}
</ul>
</div>
</div>
);
}
export default App;
|
CSS
body {
font-family : Arial , sans-serif ;
margin : 0 ;
padding : 0 ;
background-color : #f4f4f4 ;
}
header {
background-color : #333 ;
color : #fff ;
text-align : center ;
padding : 1em 0 ;
}
section {
max-width : 600px ;
margin : 2em auto ;
background-color : #fff ;
padding : 20px ;
border-radius: 8px ;
box-shadow: 0 0 10px rgba( 0 , 0 , 0 , 0.1 );
}
#totalAmount {
font-size : 24px ;
margin-bottom : 20px ;
}
form {
display : flex;
flex- direction : column;
}
label {
margin-bottom : 8px ;
}
input {
padding : 10px ;
margin-bottom : 16px ;
border : 1px solid #ccc ;
border-radius: 4px ;
}
button {
background-color : #4caf50 ;
color : #fff ;
padding : 10px ;
border : none ;
border-radius: 4px ;
cursor : pointer ;
}
|
Starting the React App.
npm start
Output of data saved in the Database:
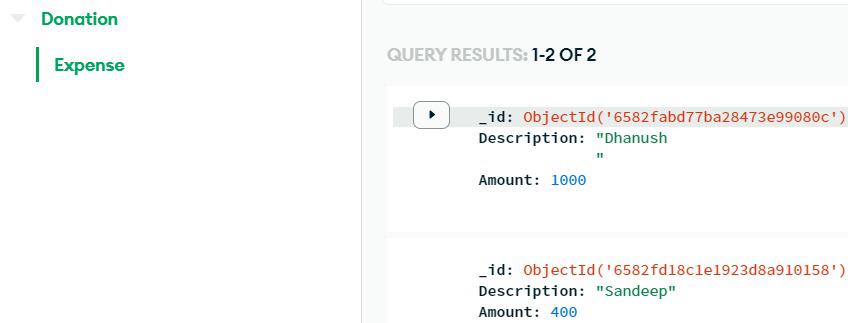
Output: Visit http://localhost:3000 in your browser to see the MERN fundraising app in action.
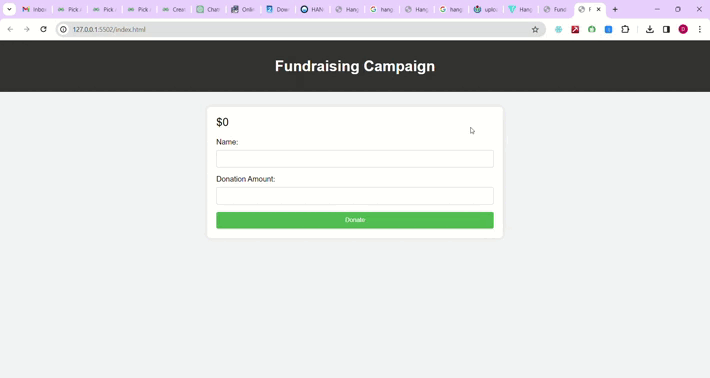
Output
Share your thoughts in the comments
Please Login to comment...