ReactJS In-Page Navigation
Last Updated :
30 Oct, 2023
React JS in-page navigation refers to navigating to the component present on the same page. These components are present on the same page so we simply have to scroll to that specific component for navigation.
Prerequisites
Approach
For in-page Navigation in React JS we will be using the React Anchor link Smooth Scroll library that takes the id of the component and on click scroll to that same component to implement the in-page navigation.
Syntax
<AnchorLink href=''/>
We mention the id of the component we want to go to the href like “#id”.
Features of React Anchor Link Smooth Scroll:
- Light weight module.
- Very easy to use just like anchor tag.
- Smooth scrolling feature better for user experience.
Creating React Application
Step 1: Create a React application using the following command:
npx create-react-app project
Step 2: After creating your project folder(i.e. project), move to it by using the following command:
cd project
Step 3: now install the dependency react-anchor-link-smooth-scroll by using the following command:
npm i react-anchor-link-smooth-scroll
Project Structure: It will look like this. We are adding a file WelcomePage.js where we will create a component WelcomePage containing two buttons.
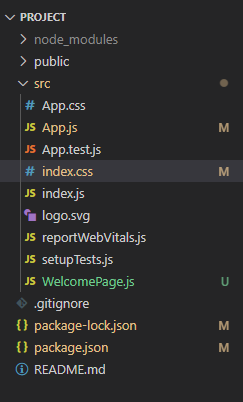
Dependencies list after installing packages
{
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-anchor-link-smooth-scroll": "^1.0.12",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
}
Example: This example demonstrates two components in AnchorLink tags using the id as the attribute to provide smooth in-page navigation.
Javascript
import AnchorLink from "react-anchor-link-smooth-scroll" ;
import WelcomePage from "./WelcomePage" ;
import "./App.css" ;
function App() {
return (
<div className= "App" >
<h1 align= "center" >Welcome To Geeksforgeeks</h1>
<h2 align= "center" >
<AnchorLink href= "#course" >
<button>Courses</button>
</AnchorLink>
<AnchorLink href= "#article" >
<button>Articles</button>
</AnchorLink>
</h2>
<WelcomePage />
</div>
);
}
export default App;
|
Javascript
import React from "react" ;
const Course = () => {
return (
<div id= "course" >Let 's Look at some courses</div>
);
};
const Article = () => {
return <div id="article">Let' s Read some Articles</div>;
};
const WelcomePage = () => {
return (
<div>
<Course />
<Article />
</div>
);
};
export default WelcomePage;
|
CSS
body {
margin : 0 ;
font-family : -apple-system, BlinkMacSystemFont,
"Segoe UI" , "Roboto" , "Oxygen" , "Ubuntu" ,
"Cantarell" , "Fira Sans" , "Droid Sans" ,
"Helvetica Neue" , sans-serif ;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
code {
font-family : source-code-pro, Menlo, Monaco, Consolas,
"Courier New" , monospace ;
}
#article {
margin-top : 1000px ;
font-size : 3 rem;
background-color : rgb ( 243 , 124 , 124 );
font-weight : 800 ;
text-align : center ;
padding : 80px ;
border : 2px solid red ;
margin-bottom : 500px ;
}
#course {
margin-top : 500px ;
border : 6px double green ;
font-size : 3 rem;
background-color : lightgreen;
font-weight : 800 ;
text-align : center ;
padding : 80px ;
}
button {
font-size : 1.5 rem;
padding : 10px 20px ;
margin-right : 10px ;
cursor : pointer ;
}
|
Step to Run Application: Run the application using the following command from the root directory of the project.
npm start
Output: In this example, when we are clicking on the buttons it smoothly scrolls down to its respective components.
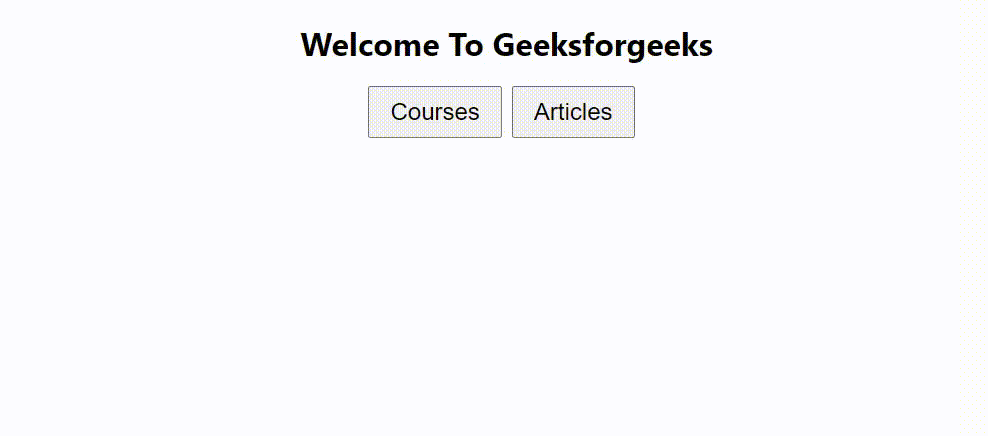
Share your thoughts in the comments
Please Login to comment...