How to prevent page from rendering before redirect in ReactJS ?
Last Updated :
07 May, 2023
ReactJS is a popular front-end framework that allows developers to create dynamic and responsive web applications. One common issue that developers face when working with ReactJS is preventing a page from rendering before a redirect is complete. This can lead to issues such as slow loading times, broken links, and a poor user experience. In this article, we will explore some best practices for preventing page rendering before a redirect in ReactJS.
Why is it important to prevent page rendering before a redirect?
The browser contacts the server to request the new page whenever a user clicks a link or submits a form on a website. The browser will begin loading the new page if the server responds with a redirect response. However, occasionally it might take a while for the new page to load, and during that time the old page might keep rendering. As a result, the user may encounter errors, broken links, or pages that have only partially loaded.
It is crucial to make sure that the browser waits until the redirect is finished before beginning to render the new page in order to avoid this from happening. This can be done using various techniques in ReactJS.
1. Use React Router– One way to prevent the page from rendering before the redirect in ReactJS is to use a React Router. A React Router allows you to manage your application’s routes and provides a way to navigate between them without causing a page reload.
To use a React Router, you will first need to install it by running the following command:
npm install react-router-dom
Once you have installed the React Router, you can use it to define your application’s routes. For example, you might define a route like this:
Javascript
import { BrowserRouter, Routes, Route }
from 'react-router-dom' ;
import Home from './pages/Home' ;
import About from "./pages/About" ;
function App() {
return (
<BrowserRouter>
<Routes>
<Route exact path= "/"
element={<Home />} />
<Route exact path= "about"
element={<About />} />
</Routes>
</BrowserRouter>
);
};
|
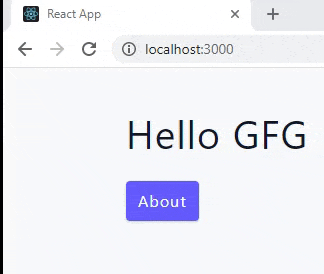
Output
The home route (“/”) in this example displays the Home component, while the about route (“/about”) does a redirect to the home route using the Redirect> component.
React Router will delay rendering the new page until the redirect is finished when a user navigates to the about route. This makes sure that no broken links or partially loaded pages are visible to the user.
2. Use the History API: Modern browsers come with a built-in capability called the History API that enables developers to control the browser history and switch between pages without having to refresh the whole thing. By replacing the existing state with the new state using the replaceState() method, developers can use the History API to stop page rendering before a redirect has finished.
Simply use the window.location.replace() method to redirect to the new URL after calling the replaceState() function on the History API with the new state and URL. This will delay rendering of the new page until the redirect is finished.
Javascript
import React from 'react' ;
const RedirectButton = () => {
const handleClick = () => {
const newState = { page: 'redirect' };
window.history.replaceState(newState,
'Redirect' , '/redirect' );
window.location.replace( '/redirect' );
};
return (
<button onClick={handleClick}>Redirect</button>
);
};
export default RedirectButton;
|
In this example, we have defined a button component called RedirectButton that, when clicked, will redirect to a new URL (“/redirect”) using the replaceState() method and the window.location.replace() method.
Conclusion: While creating web apps with ReactJS, it is crucial to prevent page rendering before a redirect is finished. Developers can make sure that the user experience is seamless and error-free by utilizing React Router or the History API method. This will prevent broken links and partially loaded pages. Developers may construct dynamic, responsive web applications that offer a fantastic user experience by adhering to these best practices.
Share your thoughts in the comments
Please Login to comment...