What is onMouseOutCapture in ReactJS ?
Last Updated :
12 Dec, 2023
The React `onMouseOutCapture` is like a watchman that notices when your mouse pointer leaves an element or its kids. It’s similar to `onMouseOut`, but the cool part is that it pays attention as soon as you start moving the mouse away, while `onMouseOut` waits a bit. Think of it as happening in two different phases of the mouse movement.
Prerequisite:
Approach to implement onMouseOutCapture Event:
- Event Handling Function: Begin by defining an event handling function,
onMouseOutCaptureHandler
, within the App
component. This function logs a message, indicating the occurrence of the onMouseOutCapture event.
- Component Structure: Utilize the React
div
element with the className “App” to structure the component. Include an <h1>
element displaying a greeting and a <p>
element where the onMouseOutCapture event is set to trigger the previously defined event handling function.
- Component Export: Conclude the component definition by exporting the
App
component as the default export. This sets up the application to display a greeting and respond to the onMouseOutCapture event on the specified paragraph element.
Syntax:
<element onMouseOutCapture={function}/>
Steps to Create React Application:
Step 1: Create a react project folder and install locally by npm i create-react-app.
npm create-react-app project
Step 2: After creating your project folder, move to it by using the following command.
cd project
Project Structure:
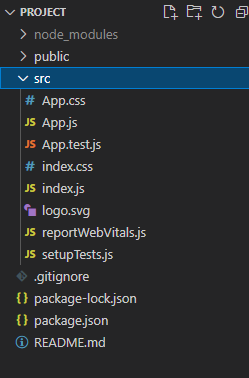
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: In this example, we are adding a text within the <p> tag i.e. ” Mouse over this line and leave!”. The onMouseOutCapture event will call onMouseOutCaptureHandler, a function that will show a text “onMouseOutCapture Event!” whenever we move the pointer of the mouse out of the element, in the console.
Javascript
function App() {
const onMouseOutCaptureHandler = () => {
console.log( "onMouseOutCapture Event!" );
};
return (
<div className= "App" >
<h1> Hey Geek!</h1>
<p onMouseOutCapture={onMouseOutCaptureHandler}>
Mouse over this line and leave!
</p>
</div>
);
}
export default App;
|
Step to Run the Application: Run the application using the following command from the root directory of the project.
npm start
Output:
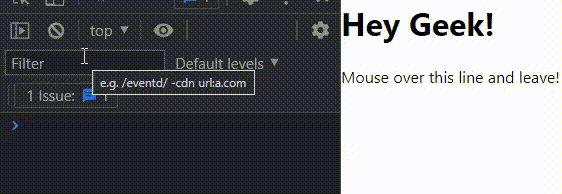
Output
Share your thoughts in the comments
Please Login to comment...