React-Motion Introduction & Installation
Last Updated :
18 Mar, 2024
The react-motion package is a JavaScript animation library for the React applications which can be used for creating smooth and interactive animations. This uses the spring-based physics model, through which we can define animations with natural and dynamic movements. This library is mainly suited for complex UI animations and transitions.
In this article, we will explore this library with practical implementation in terms of an example.
Methods of React-Motion Package:
- Motion: The Motion component is the core of React-Motion and is used to animate React components. It takes an object with key-value pairs representing the styles to animate and transitions between them using physics-based animations. This method allows smooth animations with natural motion, making it best for creating interactive UI elements.
- spring: The spring function is used to define the spring physics configuration for animations within the Motion component. It allows us to specify parameters such as stiffness, damping, and precision, which determine the behavior of the animation. By adjusting these parameters, developers can control the speed, smoothness, and responsiveness of animations, ensuring they align with the desired user experience.
- preset: The preset method is used to define common spring configurations that encapsulate various combinations of stiffness, damping, and precision values. These presets provide convenient shortcuts for creating animations with predefined physics properties, making it easier for us to achieve specific animation effects without manually tweaking individual parameters.
Approach to use react-motion package:
Using the react-motion module in React JS includes the installation of the corresponding npm package in our React project. We will import the module in the component where we will apply the smooth and animative appearance and disappearance on the content block. The animation includes opacity, translation, scaling, and rotation effects, triggered by a button click.
Steps to Create React App & Installing React-Motion:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: After creating the ReactJS application, Install the react-motion modules using the following command:
npm i react-motion
The updated dependencies in package.json file after installing required modules.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-motion": "^0.5.2",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example of React-Motion
Code: This example adds the animation to the h1 and h3 Heading when the Button is clicked. Write the code in App.js file
Javascript
// App.js
import React,
{
useState
} from 'react';
import {
Motion,
spring,
presets
} from 'react-motion';
const App = () => {
const [isAnimating, setIsAnimating] = useState(false);
const btnFn = () => {
setIsAnimating(!isAnimating);
};
return (
<div style={
{
textAlign: 'center',
marginTop: '50px'
}}>
<Motion
defaultStyle={
{
opacity: 0,
translateY: -100,
scale: 0,
rotate: 0
}}
style={{
opacity: spring(isAnimating ? 1 : 0, { precision: 0.1 }),
translateY: spring(isAnimating ? 0 : -100, presets.gentle),
scale: spring(isAnimating ? 1 : 0, presets.wobbly),
rotate: spring(isAnimating ? 360 : 0),
}}>
{
(interpolatingStyle) => (
<div
style={{
opacity: interpolatingStyle.opacity,
transform: `translateY
(${interpolatingStyle.translateY}px)
scale(${interpolatingStyle.scale})
rotate(${
interpolatingStyle.rotate
}deg)`,
transition: 'opacity 0.5s, transform 0.5s',
background: 'lightblue',
padding: '20px',
borderRadius: '8px',
}}>
<h1 style={
{ color: 'green' }}>GeeksforGeeks</h1>
<h3>React Motion Package in ReactJS</h3>
</div>
)
}
</Motion>
<button
style={{
marginTop: '20px',
padding: '10px 20px',
fontSize: '16px',
cursor: 'pointer',
background: 'orange',
border: 'none',
borderRadius: '4px',
}}
onClick={btnFn}>
{isAnimating ? 'Hide' : 'Show'} Animation
</button>
</div>
);
};
export default App;
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
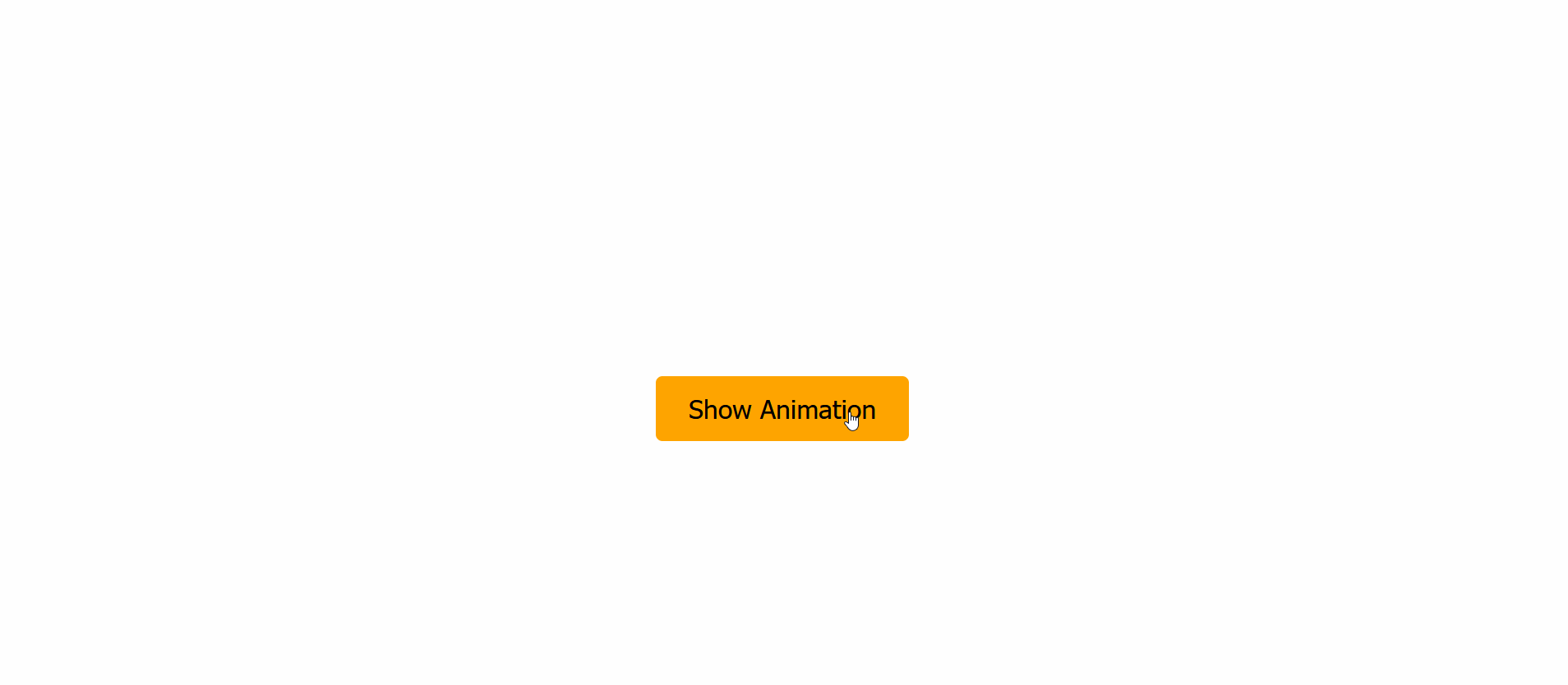
Share your thoughts in the comments
Please Login to comment...