React-Bootstrap Carousel API
Last Updated :
06 Nov, 2023
React carousels, or sliders, are a popular UI (User Interface) component used in web development to showcase multiple items or images in a single, interactive, and space-efficient container. Carousels are commonly used for displaying product images, testimonials, news articles, or any other content that can be cycled through.
Syntax:
<Carousel>
</Carousel>
We will learn about the Carousel API with the following subtopic’s:
- Carousel
- Carousel Item
- Carousel Caption
Carousel:
Syntax:
<Carousel>
</Carousel>
Carousel has many attributes some are attributes are demonstrate below.
- activeIndex: The index of currently active Item.
- onSelect: A callback function when an item is selected.
- prevIcon: Previous element of carousel element
- nextIcon: Next element of carousel element
- keyboard: If this ‘true’ carousel item will change by keyboard otherwise not.
- pause: Specifies when to pause the automatic sliding. It can be “hover”, “focus”, or “false” to disable pausing.
- direction: The direction of the slide transition. It can be either “next” or “prev”.
Carousel Item:
Syntax:
<Carousel.Item>
</Carousel.Item>
Carousel has many attributes some are attributes are demonstrate below.
- active:This specifies whether the item is the currently active slide. When active is true, this item will be displayed as the active slide. It is string attribute.
- className: A CSS class name to be added to the Carousel.Item element, allowing you to apply custom styles.
- index: The index of the item in carousel.
CarouselCaption:
The carousel caption provide the caption for the carousel text.
Syntax:
<Carousel.Caption>
{Your Caption Here}
</Carousel.Caption>
Steps to create React Application And Installing Module:
Step 1: Create a React application using the following command.
npx create-react-app foldername
Step 2: After creating your project folder i.e. folder name, move to it using the following command.
cd folder_name
Step 3: After creating the ReactJS application, Install the required modules using the following command.
npm install react-bootstrap bootstrap
Step 3: Add the below line in index.js file.
import 'bootstrap/dist/css/bootstrap.css';
Project Structure:
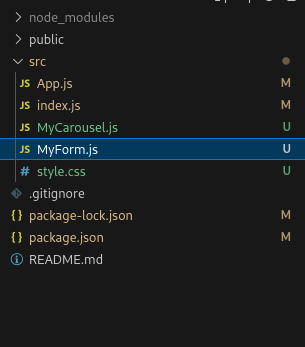
Example 1: The following program demonstrates the Carousel API.
Javascript
import React from 'react' ;
import MyCarousel from './MyCarousel' ;
function App() {
return (
<div className= 'App' >
<MyCarousel/>
</div>
)
}
export default App
|
Javascript
import React, { useState } from 'react' ;
import Carousel from 'react-bootstrap/Carousel' ;
function DynamicCarousel() {
const [index, setIndex] = useState(0);
const items = [
{
alt: 'First slide' ,
title: 'First Slide' ,
description: 'This is the first slide description.' ,
},
{
alt: 'Second slide' ,
title: 'Second Slide' ,
description: 'This is the second slide description.' ,
},
{
alt: 'Third slide' ,
title: 'Third Slide' ,
description: 'This is the third slide description.' ,
},
];
const handleSelect = (selectedIndex) => {
setIndex(selectedIndex);
};
return (
<Carousel activeIndex={index} onSelect={handleSelect}>
{items.map((item, i) => (
<Carousel.Item key={i}>
<img className= "d-block w-100" src={item.src} alt={item.alt} />
<Carousel.Caption>
<h3>{item.title}</h3>
<p>{item.description}</p>
</Carousel.Caption>
</Carousel.Item>
))}
</Carousel>
);
}
export default DynamicCarousel;
|
Steps to run the application: Run the application from the root directory of the project, using the following command.
npm start
Output:
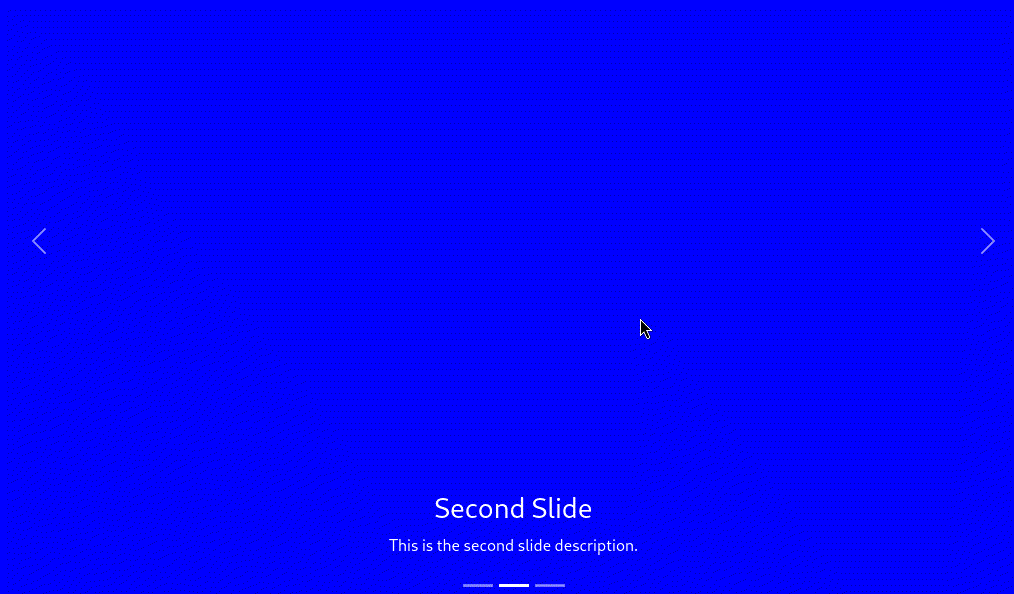
Example 2: The following program demonstrates the Carousel API with Carousel.Item using interval prop.
Javascript
import React from 'react' ;
import MyCarousel from './MyCarousel' ;
function App() {
return (
<div className= 'App' >
<MyCarousel/>
</div>
)
}
export default App
|
Javascript
import React from 'react' ;
import { Carousel } from 'react-bootstrap' ;
function MyCarousel() {
return (
<div className= 'bg-black text-center' style={{height: "100vh" }}>
<Carousel>
<Carousel.Item interval={1000}>
<img
alt= "First slide"
className= 'h'
style={{height: "450px" ,marginTop: "30px" }}
/>
<Carousel.Caption>
<h3>First Slide</h3>
<p>Some description for the first slide.</p>
</Carousel.Caption>
</Carousel.Item>
<Carousel.Item interval={500}>
<img
alt= "Second slide"
style={{height: "450px" ,marginTop: "30px" }}
/>
<Carousel.Caption>
<h3>Second Slide</h3>
<p>Some description for the second slide.</p>
</Carousel.Caption>
</Carousel.Item>
<Carousel.Item interval={200}>
<img
alt= "Third slide"
style={{height: "450px" ,marginTop: "30px" }}
/>
<Carousel.Caption>
<h3>Third Slide</h3>
<p>Some description for the third slide.</p>
</Carousel.Caption>
</Carousel.Item>
</Carousel>
</div>
);
}
export default MyCarousel;
|
Steps to run the application: Run the application from the root directory of the project, using the following command.
npm start
Output:
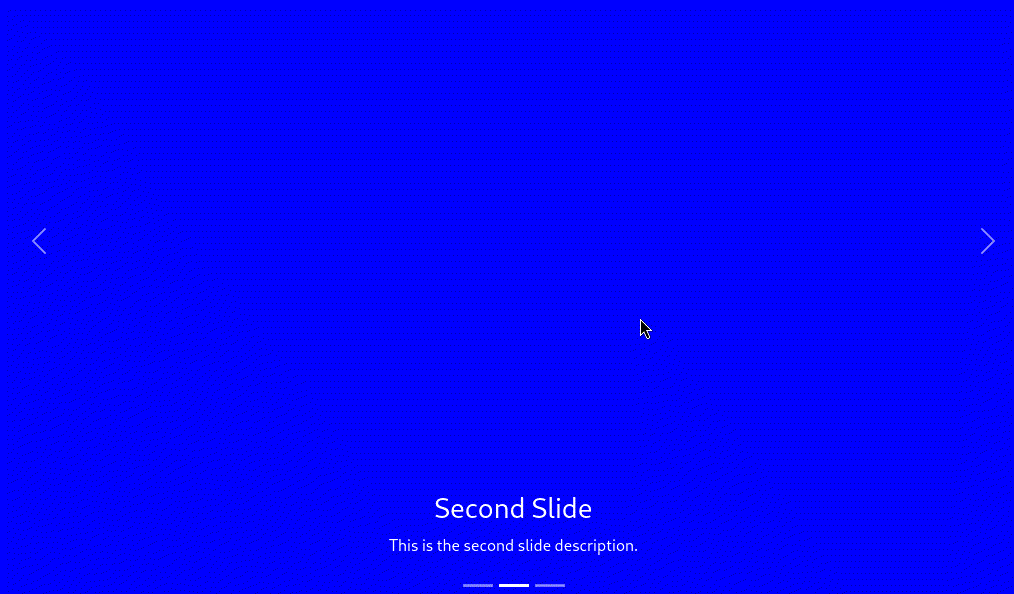
Share your thoughts in the comments
Please Login to comment...