Quiz Application using Django
Last Updated :
18 Mar, 2024
In this article, we will create the Django Quiz Application generally the Django Quiz App is a versatile and interactive web application designed to revolutionize learning and assessment. Created to address the need for engaging and adaptable online quizzes, it offers educators, businesses, and individuals a user-friendly platform to create, manage, and take quizzes on various subjects. This app is set to transform traditional learning and testing methods, making the process more enjoyable, efficient, and conducive to continuous learning.
Quiz Application using Django
To install Django follow these steps.
Starting the Project Folder
To start the project use this command
django-admin startproject quiz
cd quiz
To start the app use this command
python manage.py startapp home
Now add this app to the ‘settings.py’
File Structure
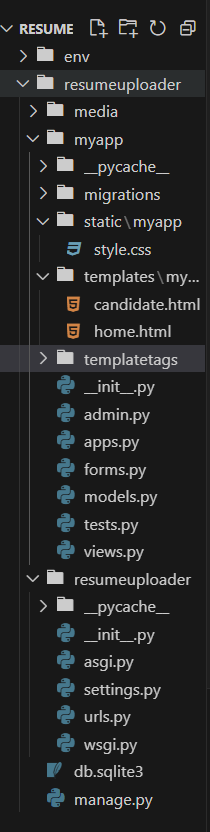
File Structure
then we register our app in settings.py file in the installed_apps sections like shown below
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home",
"django_extensions",
]
Setting up necessary Files
models.py
The code defines Django models for a quiz application. It includes models for categories, questions, and answers, along with a common base model for shared fields like timestamps. These models are used to organize and store quiz-related data in a Django project.
Python3
from django.db import models
import uuid
import random
class BaseModel(models.Model):
uid = models.UUIDField(primary_key=True, default=uuid.uuid4, editable = True)
created_at = models.DateField(auto_now_add = True)
updated_at = models.DateField(auto_now = True)
class Meta:
abstract = True
class Types(BaseModel):
gfg_name = models.CharField(max_length=100)
def __str__(self) -> str:
return self.gfg_name
class Question(BaseModel):
gfg = models.ForeignKey(Types, related_name='gfg',on_delete= models.CASCADE)
question = models.CharField(max_length=100)
marks = models.IntegerField(default = 5)
def __str__(self) -> str:
return self.question
def get_answers(self):
answer_objs = list(Answer.objects.filter(question= self))
data = []
random.shuffle(answer_objs)
for answer_obj in answer_objs:
data.append({
'answer' :answer_obj.answer,
'is_correct' : answer_obj.is_correct
})
return data
class Answer(BaseModel):
question = models.ForeignKey(Question,related_name='question_answer', on_delete =models.CASCADE)
answer = models.CharField(max_length=100)
is_correct = models.BooleanField(default = False)
def __str__(self) -> str:
return self.answer
views.py
The code is a part of a Django web application for quizzes:
home
Function: Displays a list of quiz categories. Redirects to the quiz page for a selected category.quiz
Function: Displays a quiz page for a specific category.get_quiz
Function: Retrieves random quiz questions, optionally filtered by category. Returns them as JSON. Handles exceptions with a “Something went wrong” response.
Python3
from django.shortcuts import render, redirect
from django.http import JsonResponse
from .models import *
import random
def home(request):
context = {'catgories': Types.objects.all()}
if request.GET.get('gfg'):
return redirect(f"/quiz/?gfg={request.GET.get('gfg')}")
return render(request, 'home.html', context)
def quiz(request):
context = {'gfg': request.GET.get('gfg')}
return render(request, 'quiz.html', context)
def get_quiz(request):
try:
question_objs = Question.objects.all()
if request.GET.get('gfg'):
question_objs = question_objs.filter(gfg__gfg_name__icontains = request.GET.get('gfg'))
question_objs = list(question_objs)
data = []
random.shuffle(question_objs)
for question_obj in question_objs:
data.append({
"uid" : question_obj.uid,
"gfg": question_obj.gfg.gfg_name,
"question": question_obj.question,
"marks": question_obj.marks,
"answer" : question_obj.get_answers(),
})
payload = {'status': True, 'data': data}
return JsonResponse(payload) # Return JsonResponse
except Exception as e:
print(e)
return HttpResponse("Something went wrong")
admin.py
Here we are registering the models.
Python3
from django.contrib import admin
from .models import *
# Register your models here.
class AnswerAdmin(admin.StackedInline):
model = Answer
class QuestionAdmin(admin.ModelAdmin):
inlines = [AnswerAdmin]
admin.site.register(Types)
admin.site.register(Question, QuestionAdmin)
admin.site.register(Answer)
Creating GUI
home.html
This HTML template is designed for a Django quiz app, providing a category selection form for users and using Bootstrap for styling and layout.
HTML
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.3.1/dist/css/bootstrap.min.css"
integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T"
crossorigin="anonymous">
<title>Django Quiz App</title>
</head>
<body>
<div class="container mt-5 pt-5">
<!-- Added the Geeksforgeeks heading here -->
<h1 style="color: green; text-align: center;">Geeksforgeeks</h1>
<div class="col-md-6 mx-auto">
<form action="">
<div class="form-group">
<label for="">Select Types</label>
<select name="gfg" id="" class="form-control" value="gfg">
<option value="Choose">Choose</option>
{% for type in catgories %}
<option value="{{type.gfg_name}}">{{type.gfg_name}}</option>
{% endfor %}
</select>
</div>
<button class="btn btn-danger mt-3">Submit</button>
</form>
</div>
</div>
</body>
</html>
quiz.html
This code integrates Vue.js into an HTML page to create a dynamic quiz interface where users can select answers, check correctness, and receive alerts. It fetches questions from a Django API based on the selected category.
HTML
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.3.1/dist/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous">
<title>Django Quiz App</title>
</head>
<body>
<script src="https://unpkg.com/vue@3.0.0-rc.5/dist/vue.global.prod.js"></script>
<div id="app">
<ul>
<div class="container mt-5 pt-5">
<div class="col-md-6 mx-auto">
<h3>Give Quiz</h3>
<div v-for="question in questions">
<hr>
<p>[[question.question]]</p>
<div class="form-check" v-for="answer in question.answer">
<input @change="checkAnswer($event, question.uid)" :value="answer.answer" class="form-check-input" type="radio" name="flexRadioDefault" id="flexRadioDefault1">
<label class="form-check-label" for="flexRadioDefault1">
[[answer.answer]]
</label>
</div>
</div>
</div>
</div>
<!-- <li v-for="task in tasks">[[task]]</li> -->
</ul>
</div>
<script>
const app = Vue.createApp({
el: '#app',
delimiters: ['[[', ']]'],
data() {
return {
gfg: '{{gfg}}',
questions :[]
}
},
methods : {
getQuestions(){
var _this = this
fetch(`/api/get-quiz/?gfg=${this.gfg}`)
.then(response => response.json())
.then(result =>{
console.log(result)
_this.questions = result.data
})
},
checkAnswer(event, uid){
this.questions.map(question =>{
answer = question.answer
for(var i=0; i<answer.length; i++){
if(answer[i].answer==event.target.value){
if(answer[i].is_correct){
console.log('Your answer is correct!')
alert("Hurray ypur answer is correct !????")
}else{
console.log('Your answer is wrong!')
alert("Better luck next time !????")
}
}
}
})
console.log(event.target.value , uid)
}
},
created() {
this.getQuestions( )
},
});
app.mount('#app')
</script>
</body>
</html>
quiz/urls.py
This is the urls.py file of our project quiz in this file we just map the other urls.py file of our home app for performing the some operations
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('', include('home.urls')),
path("admin/", admin.site.urls),
]
home/urls.py
This is the urls.py file this is our app home urls.py
Python3
from django.contrib import admin
from django.urls import path
from .import views
urlpatterns = [
path('', views.home , name = 'home'),
path('quiz/', views.quiz, name= 'quiz'),
path('api/get-quiz/', views.get_quiz, name='get_quiz')
]
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
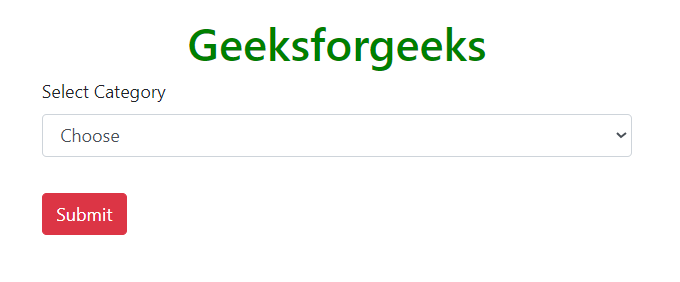
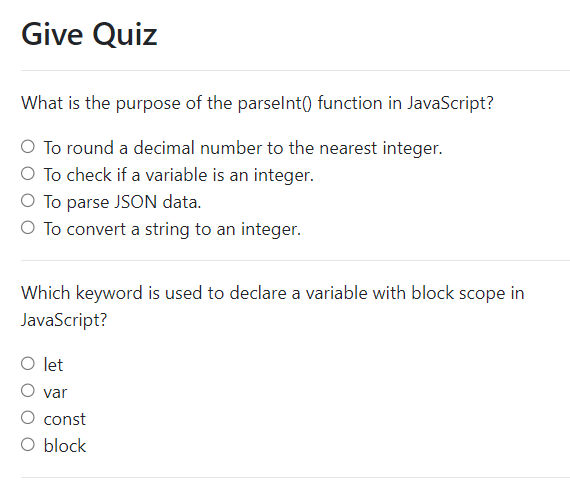
Conclusion
In conclusion, the Quiz Django app is a web application designed for creating and administering quizzes. It combines Django, a powerful Python web framework, with Vue.js, a JavaScript framework for building dynamic user interfaces. The app allows users to:
- Select a Category: Users can choose a quiz category from a dropdown menu.
- Answer Questions: The app presents users with a list of questions within the selected category. Users can select answers to these questions.
- Check Correctness: Upon selecting an answer, the app checks if it’s correct and provides immediate feedback through alerts.
- Dynamic UI: Vue.js is used to create a dynamic user interface that updates in real-time as users interact with the quiz.
- API Integration: The app fetches quiz questions from a Django API based on the selected category.
Overall, the Quiz Django app combines the power of Django for backend development and Vue.js for frontend interactivity to create an engaging and educational quiz-taking experience.
Share your thoughts in the comments
Please Login to comment...