Cryptographic Signing in Django
Last Updated :
12 Mar, 2024
Cryptographic Signing is a technique used to generate a digital signature for data, ensuring that it has not been tampered with and comes from a trusted source. In this article, we will see what is cryptographic signing in Django and how to implement it in our project.
What is Cryptographic Signing?
Cryptographic Signing is a technique used to generate a digital signature for data, ensuring that it has not been tampered with and comes from a trusted source. In Django, cryptographic signing is often employed to secure sensitive information such as user sessions, form data, and URL parameters. The framework provides a convenient way to sign and verify data using a secret key.
To use the cryptographic signing functionalities provided by Django, you need to import the signing
module. This is typically done at the beginning of your Python file or script.
from django.core import signing
Implementation of Cryptographic Signing in Django
Below is the guide to the Implementation of Cryptographic Signing in Django:
Starting the Project Folder
To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp home
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home",
]
File Structure
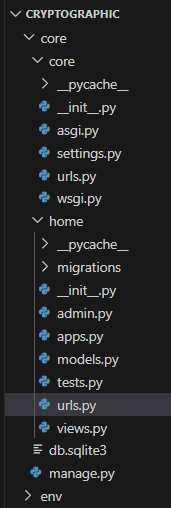
Setting Necessary Files
views.py : This Django code demonstrates a function called `sign_data` designed for web requests. It creates a dictionary of user information, defines a secret key, and utilizes Django’s `signing` module to sign the data. The resulting signed token is incorporated into an HTTP response, indicating the successful signing of the user data.
Python3
from django.http import HttpResponse
from django.core import signing
def sign_data(request):
# Data to be signed
data = {'user_id': 1, 'username': 'Alice_Charlie'}
# Secret key for signing
secret_key = 'Geeks_for_Geeks'
# Sign the data
signed_data = signing.dumps(data, key=secret_key)
return HttpResponse(f"Success!Your data has been signed successfully. Here's your signed token: {signed_data}")
project/urls.py : This Django code sets up two URL patterns: one for the admin interface at ‘/admin/’ and another for routing requests to the ‘home’ app, which is included through ‘home.urls’.
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('home.urls')),
]
app/urls.py : This Django code defines a single URL pattern that maps the root URL (‘/’) to the `sign_data` function in the current app’s `views` module, identified by the name ‘sign_data’.
Python3
from django.urls import path
from . import views
urlpatterns = [
path('sign/', views.sign_data, name='sign_data'),
]
Deployment of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
Usage of Cryptographic Signing Token
- User Authentication: Ensure secure user logins and authenticate subsequent requests using signed tokens.
- Data Integrity: Safeguard data during transmission by signing it, preventing tampering.
- Session Management: Securely manage user sessions by employing signed tokens to validate and track sessions.
- API Security: Enhance web API security by utilizing signed tokens for authenticating and validating requests.
- Authorization and Access Control: Convey authorization information through signed tokens to enforce access control policies effectively.
Conclusion
In conclusion , cryptographic signing is a mechanism employed to enhance the security of data by generating a signed token using a secret key. This process ensures the integrity and authenticity of information, particularly useful in user authentication, session management, and API security. By cryptographically signing data, Django applications can verify the source and detect any unauthorized modifications.
Share your thoughts in the comments
Please Login to comment...