Tip Calculator Project Using Django
Last Updated :
09 Feb, 2024
In this article, we will guide you through the process of creating a Tip Calculator Project using Django. This interactive project requires users to input the bill amount, the desired percentage for the tip, and the number of people splitting the bill. The application will then compute and display the Total Tip per person along with the Total Amount per person. This project is not only interesting but also straightforward, making it an excellent way to delve into Django development.
Tip Calculator Project Using Django
Below is the Implementation of the Tip Calculator Project Using Django.
To install Django follow these steps.
Starting the Project Folder
To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp home
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home",
]
File Structure:
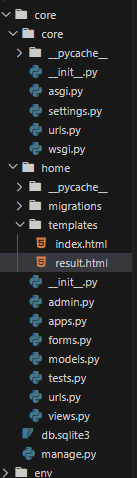
Setting Necessary Files
models.py : This Django model named `TipCalculation` has fields for bill amount, tip percentage, and number of people. It also includes optional fields for tip amount and total amount per person, allowing flexibility in data entry with blank and null values.
Python3
from django.db import models
class TipCalculation(models.Model):
bill_amount = models.DecimalField(max_digits = 10 , decimal_places = 2 )
tip_percentage = models.DecimalField(max_digits = 5 , decimal_places = 2 )
num_people = models.IntegerField()
tip_amount = models.DecimalField(max_digits = 10 , decimal_places = 2 , blank = True , null = True )
total_amount_per_person = models.DecimalField(max_digits = 10 , decimal_places = 2 , blank = True , null = True )
|
views.py : This Django code defines a tip calculator view (`tip_calculator`) that processes form input, calculates tip details, and saves them to the database. The result view (`tip_calculator_result`) fetches the latest calculation from the database and displays it using templates. The calculation logic involves a `TipCalculatorForm` and the `TipCalculation` model, with a redirect to the result page upon successful form submission.
Python3
from django.shortcuts import render, redirect
from .forms import TipCalculatorForm
from .models import TipCalculation
from decimal import Decimal
def tip_calculator(request):
if request.method = = 'POST' :
form = TipCalculatorForm(request.POST)
if form.is_valid():
instance = form.save(commit = False )
tip_percentage = Decimal(request.POST.get( 'tip_percentage' ))
instance.tip_percentage = tip_percentage
instance.tip_amount = instance.bill_amount * (tip_percentage / Decimal( 100 ))
instance.total_amount_per_person = (instance.bill_amount + instance.tip_amount) / instance.num_people
instance.save()
return redirect( 'tip_calculator_result' )
else :
form = TipCalculatorForm()
return render(request, 'index.html' , { 'form' : form})
def tip_calculator_result(request):
calculations = TipCalculation.objects.last()
return render(request, 'result.html' , { 'calculations' : calculations})
|
forms.py : This Django form (`TipCalculatorForm`) is designed for the `TipCalculation` model, with fields for bill amount and number of people. It includes a radio button selection for tip percentages (5%, 10%, 15%, 25%). The form is tailored to capture input for tip calculations in the associated model.
Python3
from django import forms
from .models import TipCalculation
class TipCalculatorForm(forms.ModelForm):
class Meta:
model = TipCalculation
fields = [ 'bill_amount' , 'num_people' ]
tip_percentage = forms.ChoiceField(choices = [( 5 , '5%' ), ( 10 , '10%' ), ( 15 , '15%' ), ( 25 , '25%' )], widget = forms.RadioSelect)
|
project/urls.py : This Django configuration sets up URL patterns. The admin interface is accessible at `/admin/`, and the root URL (`/`) includes the URL patterns defined in the `home.urls` module, facilitating modular URL handling.
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' home.urls')),
]
|
app/urls.py : This Django code sets up URL patterns for the ‘tip_calculator/’ and ‘tip_calculator_result/’ paths, linking them to the corresponding views. The names ‘tip_calculator’ and ‘tip_calculator_result’ can be used as references in the project.
Python3
from django.urls import path
from .views import tip_calculator, tip_calculator_result
urlpatterns = [
path( 'tip_calculator/' , tip_calculator, name = 'tip_calculator' ),
path( 'tip_calculator_result/' , tip_calculator_result, name = 'tip_calculator_result' ),
]
|
Creating GUI
index.html : This HTML code defines a simple web page for a Tip Calculator. It includes a form with fields for Bill Amount, Tip Percentage, and Number of People. The styling uses flexbox for layout, and the form submission is directed to the ‘tip_calculator’ URL. Tip Percentage options are presented as radio buttons. The styling emphasizes clarity and a clean design for user input.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Tip Calculator</ title >
< style >
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 0;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
h2 {
text-align: center;
color: #333;
margin-bottom: 20px;
}
form {
background-color: #fff;
border-radius: 10px;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
display: flex;
flex-direction: column;
align-items: center;
}
label {
display: block;
font-weight: bold;
margin-bottom: 5px;
}
input, select {
width: 100%;
padding: 8px;
margin-bottom: 10px;
box-sizing: border-box;
border: 1px solid #ccc;
border-radius: 4px;
}
.tip-options {
display: flex;
margin-bottom: 10px;
}
.tip-option {
margin-right: 10px;
}
button {
background-color: #4caf50;
color: #fff;
padding: 10px;
border: none;
border-radius: 4px;
cursor: pointer;
width: 100%;
}
button:hover {
background-color: #45a049;
}
</ style >
</ head >
< body >
< form method = "post" action = "{% url 'tip_calculator' %}" >
< h2 >Tip Calculator</ h2 >
< br >
{% csrf_token %}
< label for = "{{ form.bill_amount.id_for_label }}" >Bill Amount</ label >
{{ form.bill_amount }}
< br >
< label for = "{{ form.tip_percentage.id_for_label }}" >Tip Percentage</ label >
< div class = "tip-options" >
{% for choice in form.tip_percentage %}
< div class = "tip-option" >
{{ choice.tag }} {{ choice.choice_label }}
</ div >
{% endfor %}
</ div >
< br >
< label for = "{{ form.num_people.id_for_label }}" >Number of People</ label >
{{ form.num_people }}
< br >
< button type = "submit" >Calculate</ button >
</ form >
</ body >
</ html >
|
result.html: This HTML code creates a result page for the Tip Calculator, presenting calculated tip and total amounts per person. The design features a centered layout with clear typography and a link to calculate another tip. Amounts are displayed with the currency symbol “₹” for Indian Rupees.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Tip Calculator Result</ title >
< style >
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 0;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
h2 {
text-align: center;
color: #333;
}
.result-container {
background-color: #fff;
border-radius: 10px;
padding: 20px;
width: 250px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
text-align: center;
}
.result-text {
font-size: 18px;
margin-bottom: 15px;
font-family: 'Times New Roman', Times, serif;
}
.result-value {
font-size: 20px;
font-weight: bold;
color: #4caf50;
}
.calculate-another-link {
display: block;
margin-top: 20px;
text-align: center;
text-decoration: none;
color: #333;
}
.calculate-another-link:hover {
text-decoration: underline;
}
</ style >
</ head >
< body >
< div class = "result-container" >
< h2 >Result</ h2 >
< p class = "result-text" >Tip / Person: < span class = "result-value" >₹ {{ calculations.tip_amount }}</ span ></ p >
< p class = "result-text" >Total / Person: < span class = "result-value" >₹ {{ calculations.total_amount_per_person }}</ span ></ p >
< a class = "calculate-another-link" href = "{% url 'tip_calculator' %}" >Calculate Another Tip</ a >
</ div >
</ body >
</ html >
|
Deployment of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
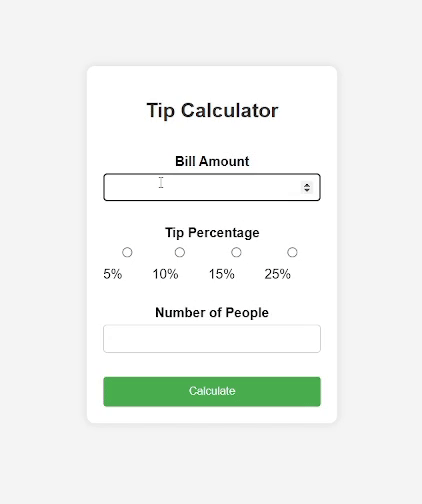
Share your thoughts in the comments
Please Login to comment...