Build a Django Application to Perform CRUD Operations
Last Updated :
17 Apr, 2024
This project provides a comprehensive system for managing recipe data, including the ability to create, view, edit, and delete recipes. It demonstrates the fundamental operations of a CRUD application for recipe management using Django, typically used in web applications for organizing and maintaining recipe collections.
CRUD Operations In Django
the “Recipe Update” project is a part of a CRUD (Create, Read, Update, Delete) application for managing recipes. Here’s a summary of its key functionality:
- Create: The project enables users to create new recipes by providing a name, description, and an image upload.
- Read: Users can view a list of recipes with details, including names, descriptions, and images. They can also search for recipes using a search form.
- Update: Users can update existing recipes by editing their names, descriptions, or images. This functionality is provided through a form that populates with the recipe’s current details.
- Delete: The project allows users to delete recipes by clicking a “Delete” button associated with each recipe entry in the list.
Starting the Project Folder
To install Django follow these steps. To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp recipe
Setting up Necessary Files
setting.py: After creating the app we need to register it in settings.py in the installed_apps section like below
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"receipe",
]
models.py: The code defines a Django model named Recipe
that represents recipes. It includes fields for the user who created the recipe, the recipe’s name, description, image, and the number of times the recipe has been viewed. The user field is linked to the built-in User
model and can be null, allowing for recipes without a specific user.
Python3
from django.db import models
from django.contrib.auth.models import User
class Receipe(models.Model):
user = models.ForeignKey(User, on_delete=models.SET_NULL, null=True, blank=True)
receipe_name = models.CharField(max_length=100)
receipe_description = models.TextField()
receipe_image = models.ImageField(upload_to="receipe")
receipe_view_count = models.PositiveIntegerField(default=1)
views.py: The code is part of a Django web application for managing recipes. It includes functions for:
- Displaying and creating recipes, with the ability to filter recipes by name.
- Deleting a specific recipe.
- Updating an existing recipe, including the option to change the recipe’s image.
These functions are responsible for various recipe-related actions in the application.
Python3
from django.shortcuts import render, redirect
from .models import Receipe # Assuming "Receipe" is the correct model name
from django.http import HttpResponse
def receipes(request):
if request.method == 'POST':
data = request.POST
receipe_image = request.FILES.get('receipe_image')
receipe_name = data.get('receipe_name')
receipe_description = data.get('receipe_description')
Receipe.objects.create(
receipe_image=receipe_image,
receipe_name=receipe_name,
receipe_description=receipe_description,
)
return redirect('/')
queryset = Receipe.objects.all()
if request.GET.get('search'):
queryset = queryset.filter(
receipe_name__icontains=request.GET.get('search'))
context = {'receipes': queryset}
return render(request, 'receipes.html', context)
def delete_receipe(request, id):
queryset = Receipe.objects.get(id=id)
queryset.delete()
return redirect('/')
def update_receipe(request, id):
queryset = Receipe.objects.get(id=id)
if request.method == 'POST':
data = request.POST
receipe_image = request.FILES.get('receipe_image')
receipe_name = data.get('receipe_name')
receipe_description = data.get('receipe_description')
queryset.receipe_name = receipe_name
queryset.receipe_description = receipe_description
if receipe_image:
queryset.receipe_image = receipe_image
queryset.save()
return redirect('/')
context = {'receipe': queryset}
return render(request, 'update_receipe.html', context)
admin.py: We register the models in admin.py file
Python
from django.contrib import admin
from .models import *
from django.db.models import Sum
admin.site.register(Receipe)
Creating GUI for app
receipes.html: First we created the receipes.html file this Django template code is a part of a web page for adding, searching, displaying, updating, and deleting recipes. Here’s a simplified explanation.
HTML
{% extends "base.html" %}
{% block start %}
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
<style>
.text{
color: green;
font-weight: bold;
}
</style>
<div class="container mt-5">
<form class="col-6 mx-auto card p-3 shadow-lg" method="post" enctype="multipart/form-data">
{% csrf_token %}
<h2 class="text text-center"> GeeksforGeeks </h2>
<br>
<h3>Add Receipe</h3>
<hr>
<div class="form-group">
<label for="exampleInputEmail1">Receipe name</label>
<input name="receipe_name" type="text" class="form-control" required>
</div>
<div class="form-group">
<label for="exampleInputPassword1" >Receipe description</label>
<textarea name="receipe_description" class="form-control" required ></textarea>
</div>
<div class="form-group">
<label for="exampleInputPassword1">Receipe Image</label>
<input name="receipe_image" type="file" class="form-control" >
</div>
<button type="submit" class="btn btn-success">Add Receipe</button>
</form>
<hr>
<div class="class mt-5">
<form action="">
<div class="max-auto col-6">
<div class="form-group">
<label for="exampleInputEmail1">Search Food</label>
<input name="search" type="text" class="form-control">
</div>
<button type="submit" class="btn btn-primary "> Search</button>
</form>
</div>
</div>
<table class="table mt-5">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Receipe name</th>
<th scope="col">Receipe Desc</th>
<th scope="col">Image</th>
<th scope="col">Actions</th>
</tr>
</thead>
<tbody>
{% for receipe in receipes %}
<tr>
<th scope="row">{{forloop.counter}}</th>
<td>{{receipe.receipe_name}}</td>
<td>{{receipe.receipe_description}}</td>
<td>
<img src="/media/{{receipe.receipe_image}}" style="height: 100px;"> </td>
<td>
<a href="/delete_receipe/{{receipe.id }}" class="btn btn-danger m-2">Delete</a>
<a href="/update_receipe/{{receipe.id }}" class="btn btn-success">Update</a>
</td>
</tr>
{% endfor %}
</tbody>
</table>
</div>
{% endblock %}
update_receipe.html: This template provides a user-friendly interface for editing the details of a recipe, including its name, description, and image. When a user submits the form, it likely sends the updated data to a Django view for processing and updating the database record.
HTML
{% extends "base.html" %}
{% block start %}
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
<style>
.text{
color: green;
font-weight: bold;
}
</style>
<div class="container mt-5">
<form class="col-6 mx-auto card p-3 shadow-lg" method="post" enctype="multipart/form-data">
{% csrf_token %}
<h2 class="text text-center"> GeeksforGeeks </h2>
<h>Update Receipe</h>
<hr>
<div class="form-group">
<label for="exampleInputEmail1">Receipe name</label>
<input name="receipe_name" value="{{receipe.receipe_name}}" type="text" class="form-control" required>
</div>
<div class="form-group">
<label for="exampleInputPassword1">Receipe description</label>
<textarea name="receipe_description" value="" class="form-control"
required>{{receipe.receipe_description}}</textarea>
</div>
<div class="form-group">
<label for="exampleInputPassword1">Receipe Image</label>
<input name="receipe_image" type="file" class="form-control">
</div>
<button type="submit" class="btn btn-success">Update Receipe</button>
</form>
</div>
{% endblock %}
base.html: It is the base HTML file which is extended by all other HTML files.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{{page}}</title>
<style>
table {
width: 80%;
margin: 20px auto;
border-collapse: collapse;
}
th,
td {
padding: 10px;
text-align: left;
border: 1px solid #ccc;
}
th {
background-color: #f2f2f2;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
tr:hover {
background-color: #ddd;
}
</style>
</head>
<body>
{% block start %}
{% endblock %}
<script>
console.log('Hey Django')
</script>
</body>
</html>
urls.py: Setting up all the paths for our function.
Python3
from django.contrib import admin
from django.urls import path
from receipe import views
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.receipes),
path('update_receipe/<id>', views.update_receipe, name='update_receipe'),
path('delete_receipe/<id>', views.delete_receipe, name='delete_receipe'),
]
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
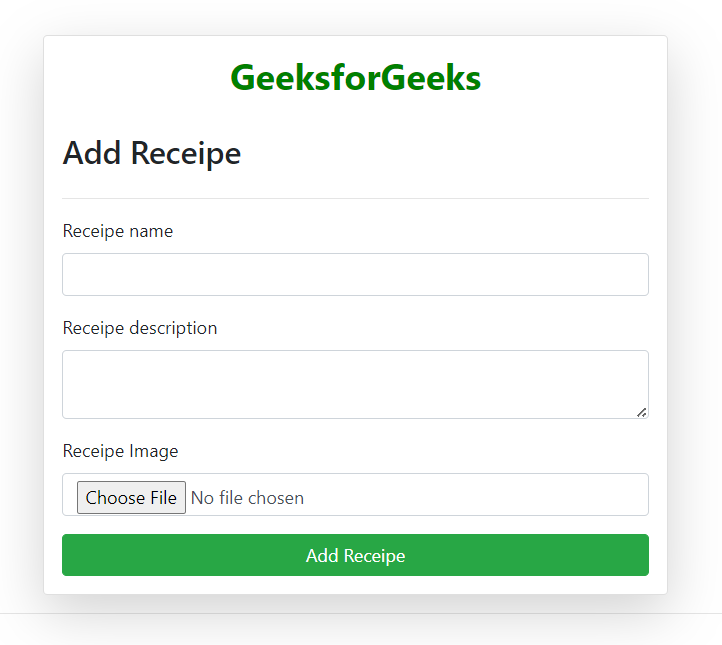
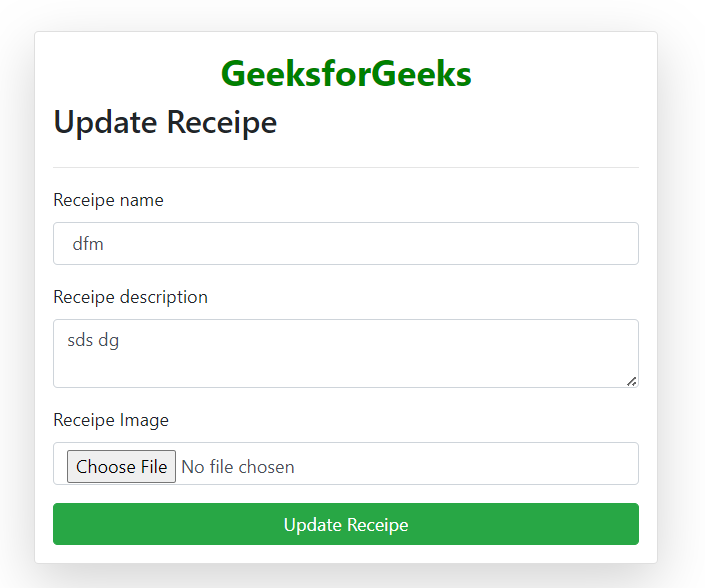
Share your thoughts in the comments
Please Login to comment...