E-commerce Product Catalog using Django
Last Updated :
09 Oct, 2023
Django is an excellent choice for building a robust product catalog. Django, a high-level Python web framework, provides a solid foundation for creating feature-rich and scalable e-commerce websites. In this article, we will explore how to build an e-commerce product catalog using Django.
E-commerce Product Catalog using Django
To install Django follow these steps.
Starting the Project Folder
To start the project use this command
django-admin startproject ecommerce
cd ecommerce
To start the app use this command
python manage.py startapp catalog
Now add this app to the ‘settings.py’
Setting up the files
model.py: Here we have created a Product table with name, description, price, image, created_at, and updated_at field in the table.
Python3
from django.db import models
class Product(models.Model):
name = models.CharField(max_length = 255 )
description = models.TextField()
price = models.DecimalField(max_digits = 10 , decimal_places = 2 )
image = models.ImageField(upload_to = 'products/' )
created_at = models.DateTimeField(auto_now_add = True )
updated_at = models.DateTimeField(auto_now = True )
def __str__( self ):
return self .name
|
admin.py: Here we are registering our table in the admin.
Python3
from django.contrib import admin
from .models import Product
admin.site.register(Product)
|
views.py: It includes view functions for displaying product lists and details, with routing and HTML templates. The Product model is assumed to represent products. Additional features like user authentication and shopping cart functionality need to be added for a complete e-commerce solution
Python3
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects. all ()
return render(request, 'myapp/index.html' , { 'products' : products})
def product_detail(request, pk):
product = Product.objects.get(pk = pk)
return render(request, 'myapp/index2.html' , { 'product' : product})
from django.http import HttpResponse
def home(request):
return HttpResponse( 'Hello, World!' )
|
urls.py: Define the URL patterns in the urls.py file of the catalog app to map views to URLs.
Python3
from django.urls import path
from . import views
urlpatterns = [
path( '/home' , views.home, name = 'home' ),
path(' ', views.product_list, name=' product_list'),
path( '<int:pk>/' , views.product_detail, name = 'product_detail' ),
]
|
Creating GUI for Project
These HTML templates use Django template language ({% … %}) to render dynamic data from your Django models. In the product list template, it loops through the products queryset and displays each product’s name, description, price, and an image. The product detail template shows detailed information about a single product and provides a link to go back to the product list.
index.html: This HTML file is used to Display all the products on the page.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >Product Catalog</ title >
< style >
/* Add CSS styles for flex container and items */
.product-list {
display: flex;
flex-wrap: wrap; /* Allow items to wrap to the next row if necessary */
justify-content: space-between; /* Space items evenly along the main axis */
list-style: none; /* Remove list styles */
padding: 0;
}
.product-item {
flex: 1; /* Grow to fill available space evenly */
max-width: 30%; /* Limit item width to avoid overcrowding */
margin: 10px; /* Add spacing between items */
border: 1px solid #ccc; /* Add a border for visual separation */
padding: 10px;
text-align: center;
}
/* Style the "Buy Now" button */
.buy-now-button {
display: block;
margin-top: 10px;
background-color: #007bff;
color: #fff;
text-decoration: none;
padding: 5px 10px;
border-radius: 5px;
}
</ style >
</ head >
< body >
< h1 >Product Catalog</ h1 >
< ul class = "product-list" >
{% for product in products %}
< li class = "product-item" >
< a href = "{% url 'product_detail' product.pk %}" >
< img src = "{{ product.image.url }}" alt = "{{ product.name }}" width = "100" >
</ a >
< h2 >{{ product.name }}</ h2 >
< p >{{ product.description }}</ p >
< p >Price: ${{ product.price }}</ p >
< a href = "#" class = "buy-now-button" >Buy Now</ a >
</ li >
{% endfor %}
</ ul >
</ body >
</ html >
|
index2.html
This HTML file is used to view the particular product on the page.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >{{ product.name }} - Product Detail</ title >
</ head >
< body >
< h1 >{{ product.name }} - Product Detail</ h1 >
< div >
< img src = "{{ product.image.url }}" alt = "{{ product.name }}" width = "200" >
</ div >
< h2 >{{ product.name }}</ h2 >
< p >{{ product.description }}</ p >
< p >Price: ${{ product.price }}</ p >
< a href = "{% url 'product_list' %}" >Back to Product List</ a >
</ body >
</ html >
|
urls.py: Don’t forget to add the necessary URL patterns for serving media files in your project’s urls.py.
Python3
from django.contrib import admin
from django.urls import path, include
from django.conf.urls.static import static
from django.conf import settings
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' catalog.urls')),
] + static(settings.MEDIA_URL, document_root = settings.MEDIA_ROOT)
|
Setting Up Static and Media Files
Configure Django to serve static and media files during development. In your project’s settings.py file, add the following paths:
# Static files (CSS, JavaScript, images)
STATIC_URL = '/static/'
STATICFILES_DIRS = [BASE_DIR / "static"]
# Media files (uploaded user files)
MEDIA_URL = '/media/'
MEDIA_ROOT = BASE_DIR / "media"
# Default primary key field type
# https://docs.djangoproject.com/en/4.1/ref/settings/#default-auto-field
DEFAULT_AUTO_FIELD = 'django.db.models.BigAutoField'
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
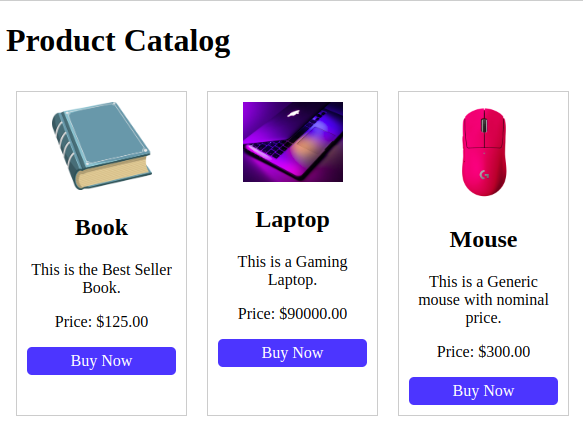
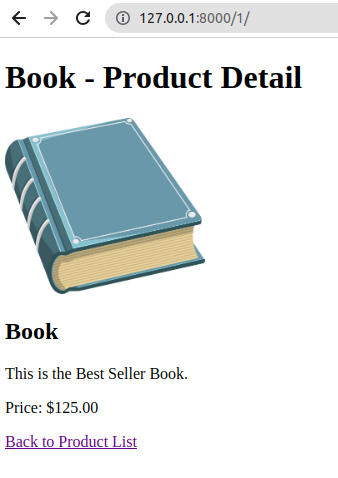
Share your thoughts in the comments
Please Login to comment...