Queue for Competitive Programming
Last Updated :
18 Jan, 2024
In competitive programming, a queue is a data structure that is often used to solve problems that involve tasks that need to be completed in a specific order. This article explores the queue data structure and identifies its role as a critical tool for overcoming coding challenges in competitive programming.
A Queue is a linear data structure that follows a First-In, First-Out (FIFO) order. This means that the first element added to the queue is the first element to be removed.
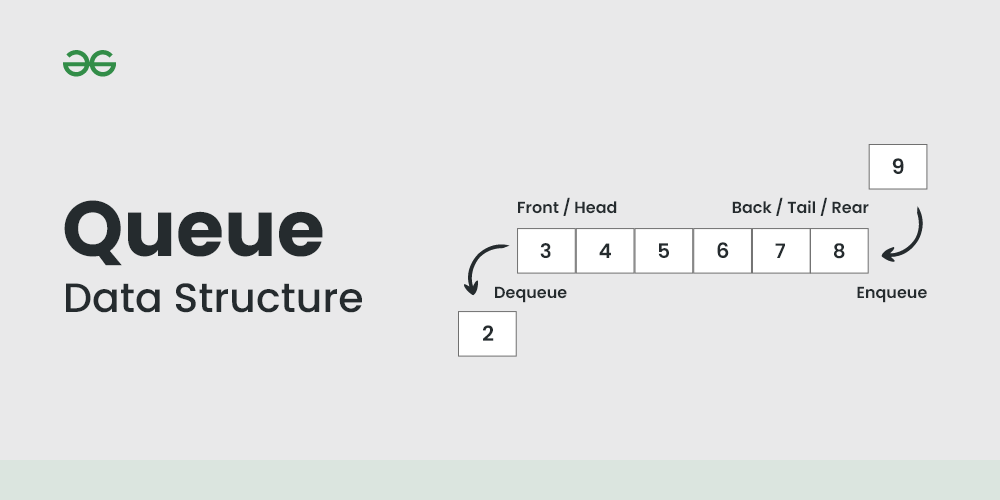
Queue Data Structure
Why to use Queue?
Queues are a useful data structure for several reasons:
- FIFO Order: Queues ensure that elements are processed in the order they were added, which is crucial for situations where order matters.
- Dynamic Nature: Queues can grow or shrink dynamically, which can be useful when solving problems related to finding maximum/minimum in subarrays.
- Breadth-first search algorithm:Â The breadth-first search algorithm uses a queue to explore nodes in a graph level-by-level. The algorithm starts at a given node, adds its neighbors to the queue, and then processes each neighbor in turn.
Idea behind a Queue
The idea behind a queue is to maintain a sequence of elements where new elements are added at one end and elements are removed from the other end. This is similar to how a line of people waiting for service operates. The person who has been waiting the longest is at the front of the line (first to be served), and new people join the line at the back (last to be served).
Use Cases of Queue in Competitive Programming
1. Min/Max Queue:
Min/max queue is a modified queue which supports finding the minimum and maximum elements in the queue efficiently. In addition to the standard enqueue and dequeue operations, a min/max queue provides operations to retrieve the current minimum or maximum element in the queue in constant time (O(1)).
Method 1: Using Dequeue and Queue
The idea is to use Doubly Ended Queue to store in increasing order if the structure is to return the minimum element and store in decreasing order if the structure is to return the maximum element.
Method 2: Using Dequeue of pairs
This method is used when we want to remove elements without knowing which element we have to remove. This is done by storing element along with the index and keeping track of how many elements we have added or removed.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
class MinQueue {
deque<pair< int , int >> q;
int added, removed;
public :
MinQueue() {
added = removed = 0;
}
int getMin() {
return q.front().first;
}
void enqueueElement( int ele) {
while (!q.empty() && q.back().first > ele)
q.pop_back();
q.push_back({ele, added++});
}
void dequeueElement() {
if (!q.empty() && q.front().second == removed)
q.pop_front();
removed++;
}
};
int main() {
MinQueue minQ;
minQ.enqueueElement(10);
minQ.enqueueElement(5);
cout << minQ.getMin() << "\n" ;
minQ.enqueueElement(3);
minQ.dequeueElement();
minQ.dequeueElement();
cout << minQ.getMin() << "\n" ;
return 0;
}
|
Java
import java.util.ArrayDeque;
import java.util.Deque;
class MinQueue {
private Deque<Pair<Integer, Integer>> q;
private int added, removed;
public MinQueue() {
q = new ArrayDeque<>();
added = removed = 0 ;
}
public int getMin() {
return q.getFirst().getKey();
}
public void enqueueElement( int ele) {
while (!q.isEmpty() && q.getLast().getKey() > ele) {
q.removeLast();
}
q.addLast( new Pair<>(ele, added++));
}
public void dequeueElement() {
if (!q.isEmpty() && q.getFirst().getValue() == removed) {
q.removeFirst();
}
removed++;
}
public static void main(String[] args) {
MinQueue minQ = new MinQueue();
minQ.enqueueElement( 10 );
minQ.enqueueElement( 5 );
System.out.println(minQ.getMin());
minQ.enqueueElement( 3 );
minQ.dequeueElement();
minQ.dequeueElement();
System.out.println(minQ.getMin());
}
}
class Pair<K, V> {
private K key;
private V value;
public Pair(K key, V value) {
this .key = key;
this .value = value;
}
public K getKey() {
return key;
}
public V getValue() {
return value;
}
}
|
Python3
from collections import deque
class MinQueue:
def __init__( self ):
self .q = deque()
self .added = 0
self .removed = 0
def get_min( self ):
return self .q[ 0 ][ 0 ]
def enqueue_element( self , ele):
while self .q and self .q[ - 1 ][ 0 ] > ele:
self .q.pop()
self .q.append((ele, self .added))
self .added + = 1
def dequeue_element( self ):
if self .q and self .q[ 0 ][ 1 ] = = self .removed:
self .q.popleft()
self .removed + = 1
if __name__ = = "__main__" :
min_q = MinQueue()
min_q.enqueue_element( 10 )
min_q.enqueue_element( 5 )
print (min_q.get_min())
min_q.enqueue_element( 3 )
min_q.dequeue_element()
min_q.dequeue_element()
print (min_q.get_min())
|
C#
using System;
using System.Collections.Generic;
public class MinQueue
{
private Queue<Tuple< int , int >> q = new Queue<Tuple< int , int >>();
private int added, removed;
public MinQueue()
{
added = removed = 0;
}
public int GetMin()
{
return q.Peek().Item1;
}
public void EnqueueElement( int ele)
{
while (q.Count > 0 && q.Peek().Item1 > ele)
q.Dequeue();
q.Enqueue(Tuple.Create(ele, added++));
}
public void DequeueElement()
{
if (q.Count > 0 && q.Peek().Item2 == removed)
q.Dequeue();
removed++;
}
}
class Program
{
static void Main( string [] args)
{
MinQueue minQ = new MinQueue();
minQ.EnqueueElement(10);
minQ.EnqueueElement(5);
Console.WriteLine(minQ.GetMin());
minQ.EnqueueElement(3);
minQ.DequeueElement();
minQ.DequeueElement();
Console.WriteLine(minQ.GetMin());
}
}
|
Javascript
class MinQueue {
constructor() {
this .q = [];
this .added = 0;
this .removed = 0;
}
getMin() {
return this .q[0][0];
}
enqueueElement(ele) {
while ( this .q.length > 0 && this .q[ this .q.length - 1][0] > ele) {
this .q.pop();
}
this .q.push([ele, this .added++]);
}
dequeueElement() {
if ( this .q.length > 0 && this .q[0][1] === this .removed) {
this .q.shift();
}
this .removed++;
}
}
const minQ = new MinQueue();
minQ.enqueueElement(10);
minQ.enqueueElement(5);
console.log(minQ.getMin());
minQ.enqueueElement(3);
minQ.dequeueElement();
minQ.dequeueElement();
console.log(minQ.getMin());
|
Time Complexity: Since each element is added once and removed once, so both enqueue and dequeue have O(1) time complexity and we can find the min element by accessing the front of the queue so getMin() operation has O(1) time complexity as well.
Auxiliary Space: O(N) to store all elements in the queue.
If we are given an array of N numbers and we need to find the minimum/maximum of all subarrays of size K, then we can easily solve this my maintaining a min/max queue.
3. Shortest subarray with sum at least K (array can have negative integers as well):
If we are given an array of N integers (positive and negative) and we need to find the smallest length of a subarray whose sum >= K, then we can use a variation of min queue to get the smallest length. Refer this article to know more about using a deque to find the shortest subarray with sum at least K.
Queues are used in multiple graph algorithms like Breadth First Search, Topological Sort, Shortest Path Algorithms like Dijkstra’s Algorithm, etc.
We can calculate the longest Subarray with maximum absolute difference using sliding window and two monotonic queues to keep track of the window max and window min.
Practice Problems on Queues for Competitive Programming:
Easy Level Problems on Queues:
Medium Level Problems on Queues:
Hard Level Problem on Queues:
Share your thoughts in the comments
Please Login to comment...