Props vs Data in Vue.js
Last Updated :
30 Apr, 2024
Props in Vue.js
Props in Vue.js are custom attributes that allow data to be passed from a parent component to a child component. This enables the parent component to communicate with its child components, providing them with data or functionality.
In Vue.js, props are used to pass data from parent components to child components. This approach facilitates a unidirectional data flow, enhancing component reusability and maintainability.
Syntax:
To define props in a Vue component, use the props option within the component’s options object. Props are declared as an array of strings, each string representing a prop name.
Vue.component('child-component', {
props: ['propName'],
// Component definition...
});
Example: In this example, we have a parent component passing a message as a prop to a child component.
JavaScript
// App.vue
<script setup>
import { ref } from 'vue';
import ParentComponent from './ParentComponent.vue';
// Define a reactive variable for the count
const count = ref(0);
</script>
<template>
<div>
<!-- Display the count -->
<h1>Hello Vue 3</h1>
<button @click="count++">Count is:
{{ count }}</button>
<!-- Include the ParentComponent -->
<ParentComponent :message="count">
</ParentComponent>
</div>
</template>
<style scoped>
button {
font-weight: bold;
}
</style>
JavaScript
// ParentComponent.vue
<template>
<div>
<p>Message from parent: {{ message }}</p>
<child-component :message="message">
</child-component>
</div>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue';
import { defineProps } from 'vue';
// Define props
const props = defineProps({
message: Number,
});
</script>
JavaScript
// ChildComponent.vue
<template>
<div>
<p>Message from parent received by child: {{ message }}</p>
</div>
</template>
<script setup>
import { defineProps } from 'vue';
// Define props
const props = defineProps({
message: Number,
});
</script>
Output:
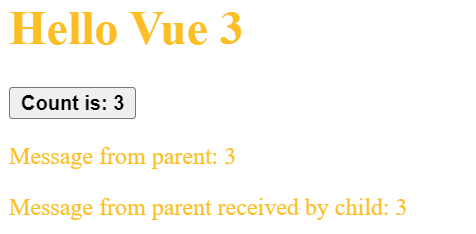
Data in Vue.js
Data in Vue.js refers to the internal state of a Vue component. It includes variables and properties that can be accessed and manipulated within the component. Data is used to manage the component’s state and reactivity. In contrast to props, data is used within a Vue component to manage its internal state. Data properties are accessible only within the component where they are defined, making them suitable for managing local component state.
Syntax:
To define data in a Vue component, use the data option within the component’s options object. Data is typically declared as a function that returns an object containing the component’s data properties.
// When you are using CDN link
Vue.component('my-component', {
data() {
return {
myData: 'Example Data',
};
},
// Component definition...
});
Example: In this example, we have a Vue component that manages a counter internally using data.
JavaScript
<!-- App.vue -->
<template>
<div>
<h1>Hello Vue 3</h1>
<counter></counter>
</div>
</template>
<script setup>
import Counter from './Counter.vue';
</script>
<style scoped>
h1 {
color: #333;
}
</style>
JavaScript
<!-- Counter.vue -->
<template>
<div>
<p>Counter: {{ counter }}</p>
<button @click="incrementCounter">Increment</button>
</div>
</template>
<script setup>
// Import required functions from Vue
import { ref } from 'vue';
// Define a reactive variable for the counter
const counter = ref(0);
// Define a method to increment the counter
function incrementCounter() {
counter.value++;
}
</script>
<style scoped>
button {
font-weight: bold;
}
</style>
Output:
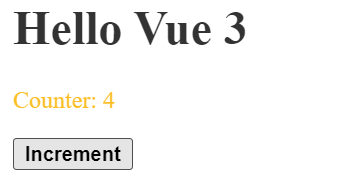
Difference between Props and Data
Feature
| Props
| Data
|
---|
Purpose
| Passing data from parent to child components
| Managing internal state of a component
|
---|
Scope
| Passed from parent component to child component
| Local to the component where it is defined
|
---|
Access Control
| Cannot be directly modified by the child component
| Can be directly modified within the component
|
---|
Reactivity
| Changes in props by parent trigger re-render in child
| Changes in data trigger reactivity within the component
|
---|
Communication Pattern
| Unidirectional: Parent to Child
| Internal to the component
|
---|
Share your thoughts in the comments
Please Login to comment...