Vue.js Props
Last Updated :
07 Nov, 2023
Vue.js Props is an attribute to the configuration of the component. It is used to pass the data to the component to work dynamically. The props configuration defines the type of data it can receive from different components of Vue.
Props Declaration
- Using defineProps(): We can declare the props using defineprops() function
<script setup >
const student = defineProps([ 'name'] )
console.log(student.name )
</scirpt>
- Using props option: We can define props using the props option:
export default {
props: [ 'name']
}
- We can also use objects in place of an array to declare props
// In script setup
defineProps({ name: String });
// In non scrpt setup
export default {
props: { name: String }
}
- We can use TypeScript pure type annotations to declare props:
<script setup lang="ts">
defineProps<{
name ?: string
}>()
</script>
Refer to the Vue.js Props Declaration article for detailed description.
Prop Passing Details
This facilitates the various components for the Props, such as Name Casing, Static vs. Dynamic Props, Passing Different Value Types, and Binding Multiple Properties Using an Object, which helps to manage & organize the Props in a structured manner in the Components.
One-Way Data Flow
In Vue.js, all the props are bonded between the child and properties are one-way-down. When we update the prop in the parent component it is updated to all the child components but the reverse does not happen and vue.js warns the use.
Cases When child component tries to change Props:
- When the Child component gets props from the parent and wants to use it as a local data property. In such a case, we can use a local variable of the child component that is initialized with props value.
props = defineProps(['props1']);
// Variable intialize with props value
temp = props1;
- When we need some props that are not in the final data, it is raw data for final data. In such a case, we can use computed property with the prop’s value.
props = defineProps(['firstName', 'lastName']);
// Applying computed property on props
fullName = computed(() => firstName + lastName);
Mutating Object / Array Props
Vue.js does not prevent the array and object mutation because the array and object are passed as a reference to the child and prevention is expensive as compared to others.
Prop Validation
Components can specify the criteria for their props, including their types. If these criteria are not met, Vue will issue a warning in the JavaScript console of the browser. This is typically valuable while generating the component meant for consumption by other developers.
Boolean Casting
Props with boolean type mimic the behavior of native boolean attributes.
// This is equal to Enable = true
<Component Enable />
// This is equal to Enable false
<Component />
When we allow multiple props Boolean casting is also applied.
// True is casted
defineProps({
Enable: [Boolean, Number]
})
// True is casted
defineProps({
Enable: [Boolean, String]
})
// True is casted
defineProps({
Enable: [Number, Boolean]
})
// Absence of Enable will casted as empty string Enable=""
defineProps({
Enable: [String, Boolean]
})
Syntax
// Using script setup
<script setup>
const props = defineProps(['data1', 'data2', ...]);
</script>
// Using non script setup
<script>
export default{
props: [ 'data1', 'data2'... ]
}
Properties
- data1: It is the variable name that is given to data1 of type define.
Example 1: In this example, we will pass name, course, age, and batch as props data and print it. Vue.js project files are:
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Home name = "Sam"
Course = "Btech"
Age = 22
Batch = "7th" />
</ template >
< script >
import Home from './components/Home.vue';
export default {
name: 'App',
components: {
Home,
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >
Props element are:< br />
</ h2 >
< h3 >Name : {{ name }}</ h3 >
< h3 >Course: {{ Course }}</ h3 >
< h3 >Age: {{ Age }}</ h3 >
< h3 >Batch: {{ Batch }}</ h3 >
</ template >
< script >
export default {
name: 'HomeDo',
props: {
name: String,
Course: String,
Age: Number,
Batch: Number,
}
}
</ script >
|
Output:
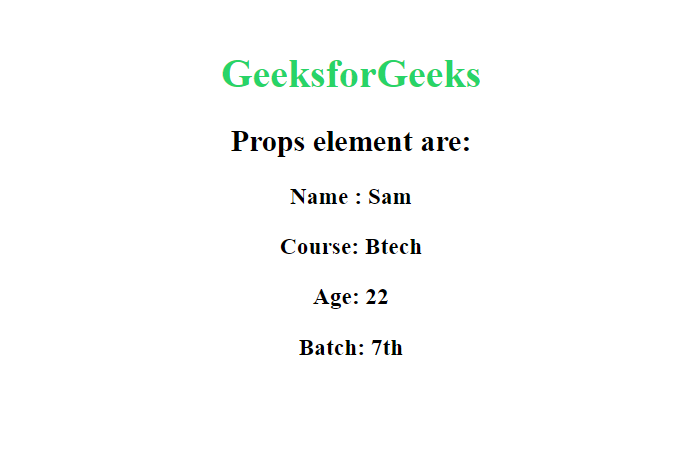
props
Example 2: In this example, we will define props with script setup. We will pass props data to the component and show it on the mouse click event.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student name = "Sam" />
</ template >
< script >
import Student from './components/Student.vue';
export default {
name: 'App',
components: {
Student,
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >Chick on botton to view the props</ h2 >
< button v-on:click.left = "data = !data" >
Show
</ button >
< h2 v-if = "data" >Geeks name is: {{ props.name }}</ h2 >
</ template >
< script setup>
import { defineProps } from "vue";
const props = defineProps(['name'])
</ script >
< script >
export default {
name: 'StudentL',
data() {
return { data: false }
}
}
</ script >
|
Output:
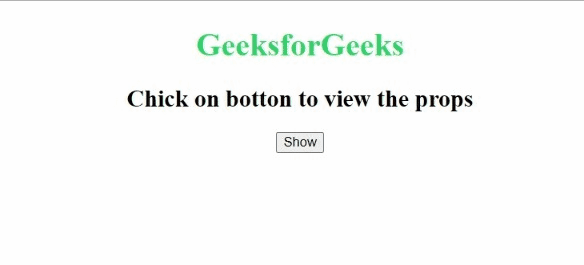
pros
Reference: https://vuejs.org/guide/components/props.html
Share your thoughts in the comments
Please Login to comment...