Vue.js Props Declaration
Last Updated :
07 Nov, 2023
Vue.js Props Declaration facilitates the declaration of props explicitly for the Vue components. With this, Vue can identify how external props are passed to the component that should be treated as fall-through attributes. In other words, Props of Vue components is a configuration for the transfer of data between the components. Props are not defined by default we have to define them to use data that is passed to components.
Different ways to define Props
- Using defineProps() : We can declare the props using defineprops() function:
<script setup >
const student = defineProps([ 'name'] )
console.log(student.name )
</scirpt>
- Using props option: We can define props using the props option:
export default {
props: ['name']
}
We can also use objects in place of an array to declare props
// In script setup
defineProps({ name: String });
// In non scrpt setup
export default {
props: { name: String }
}
We can use TypeScript pure type annotations to declare props:
<script setup lang="ts">
defineProps<{
name ?: string
}>()
</script>
Syntax
defineProps({ prop1: 'datatype', props2: 'datatype' })
props: { prop1: 'datatype', props2: 'datatype' };
Properties
- prop1: It is an identifier that is used to reference the first data received by the component as prop.
- prop2: It is an identifier that is used to reference second data received by the component as prop.
- datatype: It is the respective datatype for props.
Example 1: In this example, we will declare the props as props option and print its value.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Home name = "Sam"
Age = 23
Batch = '7h'
Course = "Btech" />
</ template >
< script >
import Home from './components/Home.vue';
export default {
name: 'App',
components: {
Home,
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >
Props element are:< br />
</ h2 >
< h3 >Name : {{ name }}</ h3 >
< h3 >Course: {{ Course }}</ h3 >
< h3 >Age: {{ Age }}</ h3 >
< h3 >Batch: {{ Batch }}</ h3 >
</ template >
< script >
export default {
name: 'HomeDo',
props: ['name', 'Course', 'Age', 'Batch']
}
</ script >
|
Output:
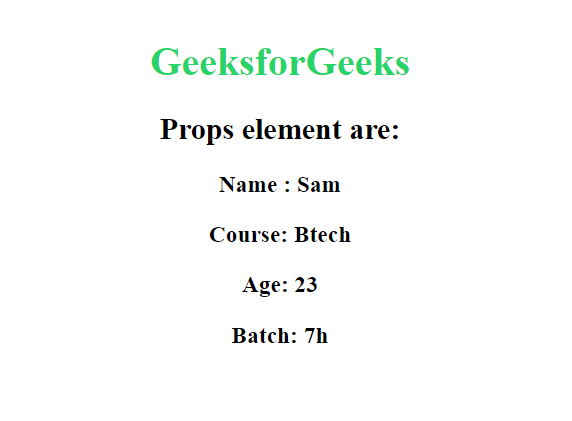
Example 2: In this example, we will declare the props using defineProps() method and print its value in DOM.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student name = "Sam"
Age = "23"
Course = "Btech"
Batch = "3rd" />
</ template >
< script >
import Student from './components/Student.vue';
export default {
name: 'App',
components: {
Student,
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >Chick on botton to view the props</ h2 >
< button v-on:click.left = "data = !data" >
Show
</ button >
< h2 v-if = "data" > Name : {{ name }} < br />
Course : {{ Course }} < br />
Age : {{ Age }}< br />
Batch : {{ Batch }}</ h2 >
</ template >
< script setup>
import { defineProps } from "vue";
defineProps({
name: String,
Course: String,
Age: String,
Batch: String
})
</ script >
< script >
export default {
name: 'StudentL',
data() {
return { data: 0 };
}
}
</ script >
|
Output:
Reference: https://vuejs.org/guide/components/props.html#props-declaration
Share your thoughts in the comments
Please Login to comment...