How to Add Multiple Data Types for Props in VueJS ?
Last Updated :
22 Dec, 2023
Props are used to pass the data from the parent component to the child component. We can pass the multiple data types for props by using the type option or by using the Dynamic props. In this article, we will see the practical implementation of adding multiple data types for props in VueJS.
Approach 1: Using type Property
In this approach, we are using the type property to specify the two props like name and age. The name prop is of type String and the age prop has multiple data types like Number and String.
Syntax:
props: {
name: {
type: String,
required: true
},
age: {
type: [Number, String],
default: 0
}
Example: The below example explains the use of type property to pass multiple type props.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Vue.js Props Example 1
</ title >
< script src =
</ script >
</ head >
< body >
< center >
< div id = "app" >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 1: Using type Property
</ h3 >
< h4 > Props Values : </ h4 >
< child-component :name = "name"
:age = "age" >
</ child-component >
< form @ submit.prevent = "updateData" >
< label for = "nameProp" >
Enter Name:
</ label >
< input type = "text"
id = "nameProp"
v-model = "name" >
< label for = "ageProp" >
Enter Age:
</ label >
< input type = "text"
id = "ageProp"
v-model = "age" >
</ form >
</ div >
</ center >
< script >
Vue.component('child-component', {
props: {
name: {
type: String,
required: true
},
age: {
type: [Number, String],
default: 0
}
},
template: `
< div >
< p >
1. Name: < b >{{ name }}</ b >,
Type of Name is: < b >{{typeof name}}</ b >
</ p >
< p >
2. Age: < b >{{ age }}</ b >,
Type of Age is: < b >{{!isNaN(age) ?
typeof Number(age) : typeof age}}</ b >
</ p >
</ div >
`
});
new Vue({
el: '#app',
data: {
name: 'Hello Geek',
age: 42
},
});
</ script >
</ body >
</ html >
|
Output:
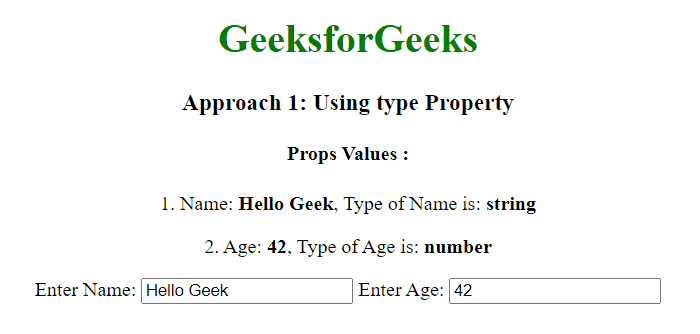
Approach 2: Using Dynamic Props Approach
In this approach, we are using Dynamic props like value and dataType. We have passed the multiple data types to the value props. The user can select the type from the selection box and based on the user input the value of that type will be shown.
Syntax:
props: {
value: [String, Number, Boolean], // Multiple data types for the 'value' prop
dataType: String, // Single data type for the 'dataType' prop
},
Example: The below example will illustrate the use of the dynamic props approach with practical implementation.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Vue.js Props Example
</ title >
< script src =
</ script >
</ head >
< body >
< div id = "app" >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 2: Using Dynamic Props Approach
</ h3 >
< label for = "dataType" >
Select Data Type:
</ label >
< select id = "dataType"
v-model = "selectedDataType"
@ change = "changeDataType" >
< option value = "String" >
String
</ option >
< option value = "Number" >
Number
</ option >
< option value = "Boolean" >
Boolean
</ option >
</ select >
< dynamic-component :value = "dynamicProp"
:dataType = "selectedDataType" >
</ dynamic-component >
</ div >
< script >
Vue.component('dynamic-component', {
props: {
value: [String, Number, Boolean],
dataType: String,
},
template: `
< div >
< p >Data Value: {{ value }}</ p >
</ div >`,
});
new Vue({
el: '#app',
data: {
dynamicProp: 'Hello, GFG!',
selectedDataType: 'String',
},
methods: {
changeDataType() {
switch (this.selectedDataType) {
case 'String':
this.dynamicProp = 'Hello, GFG!';
break;
case 'Number':
this.dynamicProp = 42;
break;
case 'Boolean':
this.dynamicProp = true;
break;
}
},
},
});
</ script >
</ body >
</ html >
|
Output:
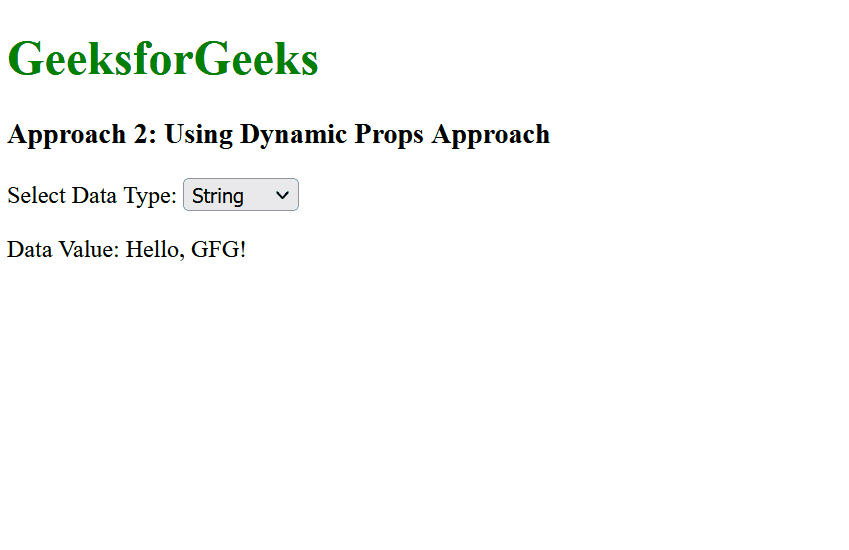
Share your thoughts in the comments
Please Login to comment...