How to Set URL Query Params in Vue with Vue-Router ?
Last Updated :
05 Jan, 2024
Vue Router is a navigation library designed specifically for Vue.js applications. In this article, we will learn about the different methods of setting the URL query params in Vue with Vue-Router. Below is the list of all possible methods.
Steps to Setup the Project Environment
Step 1: Create a VueJS application using the below command.
npm create vue@latest
Step 2: Navigate to the project that you have just created by this command.
cd your-project-name
Step 3: Run this command to install all packages
npm install
Step 4: Now, Set up vue router using below command.
npm install vue-router@4
Step 5: Create a folder inside src named views. Inside it, we will put all our components.
Step 6: Create components inside the views folder as listed below:
- AboutView.vue
- ContactView.vue
- HomeView.vue
Step 7: Create another file inside src named router.js. It will handle the routes of the application.
Step 8: Now Create a navbar inside the App.vue file to navigate easily around the webpage.
Step 9: Add the below code to main.js. It is the starting point of our application.
// main.js file
const { createApp } = require('vue');
import App from './App.vue';
import createRouter from './router';
createApp(App).use(createRouter()).mount('#app');
Project Structure:
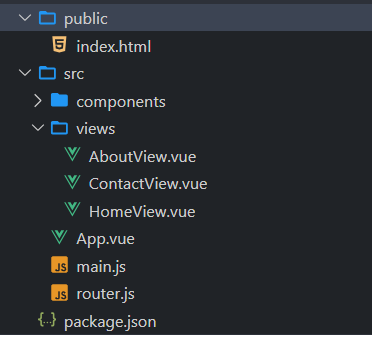
Using router-link
We use the router-link component to create links to routes with specified query parameters. The “to” prop accepts an object with the path and query properties, allowing you to include query parameters.
Syntax:
<RouterLink :to="{ path: '/yourPath', query: { //yourParams } }" ></RouterLink>
Example: The below JavaScript codes will help you implement the router-link method to set URL query params in Vue with Vue-Router.
Javascript
<!-- src/App.vue file -->
<script>
import {
RouterView,
RouterLink,
useRouter,
useRoute
}
from 'vue-router' ;
const router = useRouter();
const route = useRoute();
export default {
name: '#app' ,
};
</script>
<template>
<nav>
<RouterLink :to= "
{
path: '/',
query: { id: 1 }
}" >Home</RouterLink> |
<RouterLink :to= "
{
path: '/contact',
query: { id: 2 }
}" >Contact</RouterLink> |
<RouterLink :to= "
{
path: '/about',
query: { id: 3 }
}" >About</RouterLink>
</nav>
<RouterView />
</template>
<style>
nav {
width: 100%;
font-size: 24px;
}
nav a {
display: inline-block;
padding: 5px 10px;
margin: 10px;
}
nav a.router-link-exact-active {
background-color: green;
color: #fff;
text-decoration: none;
}
</style>
|
Javascript
import {
createRouter,
createWebHistory
}
from 'vue-router' ;
import HomeView
from './views/HomeView.vue' ;
import ContactView
from './views/ContactView.vue' ;
import AboutView
from './views/AboutView.vue' ;
const router = () =>
createRouter({
history: createWebHistory(),
routes: [
{
path: '/' ,
name: 'home' ,
component: HomeView,
},
{
path: '/about' ,
name: 'about' ,
component: AboutView,
},
{
path: '/contact' ,
name: 'contact' ,
component: ContactView,
},
],
});
export default router;
|
Javascript
const { createApp } = require( 'vue' );
import App from './App.vue' ;
import createRouter from './router' ;
createApp(App).use(createRouter()).mount( '#app' );
|
Javascript
<!-- views/HomeView.vue file -->
<template>
<h1>This is Home Page</h1>
</template>
<script>
export default {}
</script>
|
Javascript
<!-- views/ContactView.vue file -->
<template>
<h1>This is Contact Page</h1>
</template>
<script>
export default {}
</script>
|
Javascript
<!-- views/AboutView.vue file -->
<template>
<h1>This is About Page</h1>
</template>
<script>
export default {};
</script>
|
Output:
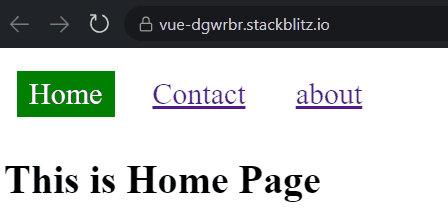
Using push() method
The push() method will help in setting the query params in the URL . You just need to replace your App.vue file with the below file in your project to implement this approach. It maintains a history stack while navigating
Syntax:
router.push({ path: '/yourPath', query: { yourparams } })
Example: The below JavaScript codes will help you implement the router-link method to set URL query params in Vue with router.push()
Javascript
<!-- App.vue file -->
<script>
import { RouterView,
RouterLink,
useRouter,
useRoute }
from 'vue-router' ;
export default {
name: '#app' ,
setup() {
const router =
useRouter();
const route =
useRoute();
function changeRoutes() {
if (router) {
router.push(
{
path: '/about' ,
query: { id: 1 }
});
}
}
return { router,
route,
changeRoutes };
},
};
</script>
<template>
<nav>
<RouterLink to= "/" >
Home
</RouterLink> |
<RouterLink to= "/contact" >
Contact
</RouterLink> |
<RouterLink to= "/about" >
About
</RouterLink><br />
<br />
<button @click= "changeRoutes" >
Set Query param using push()
</button>
</nav>
<RouterView />
</template>
<style>
nav {
width: 100%;
font-size: 24px;
}
nav a {
display: inline-block;
padding: 5px 10px;
}
nav a.router-link-exact-active {
background-color: green;
color: #fff;
text-decoration: none;
}
</style>
|
Javascript
import { createRouter,
createWebHistory }
from 'vue-router' ;
import HomeView
from './views/HomeView.vue' ;
import ContactView
from './views/ContactView.vue' ;
import AboutView
from './views/AboutView.vue' ;
const router = () =>
createRouter({
history: createWebHistory(),
routes: [
{
path: '/' ,
name: 'home' ,
component: HomeView,
},
{
path: '/about' ,
name: 'about' ,
component: AboutView,
},
{
path: '/contact' ,
name: 'contact' ,
component: ContactView,
},
],
});
export default router;
|
Javascript
const { createApp } = require( 'vue' );
import App from './App.vue' ;
import createRouter from './router' ;
createApp(App).use(createRouter()).mount( '#app' );
|
Javascript
<!-- views/AboutView.vue file -->
<template>
<h1>This is About Page</h1>
</template>
<script>
export default {};
</script>
|
Javascript
<!-- views/ContactView.vue file -->
<template>
<h1>This is Contact Page</h1>
</template>
<script>
export default {}
</script>
|
Javascript
<!-- views/HomeView.vue file -->
<template>
<h1>This is Home Page</h1>
</template>
<script>
export default {}
</script>
|
Output:
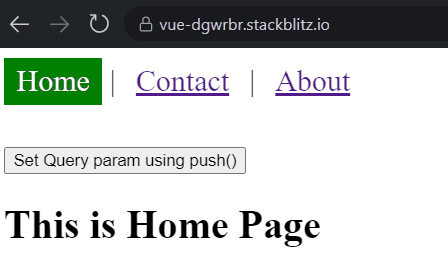
Using replace() method
This method is helps in setting the query params in the URL .You just need to replace your App.vue file with the below file to implement this approach.It do not maintain a history stack while navigating
Syntax:
router.replace({ path: '/yourPath', query: { yourParams } })
Example: The below JavaScript codes will help you implement the router-link method to set URL query params in Vue with router.replace()
Javascript
<!-- App.vue file -->
<script>
import { RouterView,
RouterLink,
useRouter,
useRoute }
from 'vue-router' ;
export default {
name: '#app' ,
setup() {
const router = useRouter();
const route = useRoute();
function changeRoutes() {
if (router) {
router.replace(
{
path: '/contact' ,
query: { id: 1 }
});
}
}
return { router, route,
changeRoutes };
},
};
</script>
<template>
<nav>
<RouterLink to= "/" >
Home
</RouterLink> |
<RouterLink to= "/contact" >
Contact
</RouterLink> |
<RouterLink to= "/about" >
About
</RouterLink> <br /><br />
<button @click= "changeRoutes" >
Change route using replace()
</button>
</nav>
<RouterView />
</template>
<style>
nav {
width: 100%;
font-size: 24px;
}
nav a {
display: inline-block;
padding: 5px 10px;
margin: 10px;
}
nav a.router-link-exact-active {
background-color: green;
color: #fff;
text-decoration: none;
}
</style>
|
Javascript
import {
createRouter,
createWebHistory
}
from 'vue-router' ;
import HomeView
from './views/HomeView.vue' ;
import ContactView
from './views/ContactView.vue' ;
import AboutView
from './views/AboutView.vue' ;
const router = () =>
createRouter({
history: createWebHistory(),
routes: [
{
path: '/' ,
name: 'home' ,
component: HomeView,
},
{
path: '/about' ,
name: 'about' ,
component: AboutView,
},
{
path: '/contact' ,
name: 'contact' ,
component: ContactView,
},
],
});
export default router;
|
Javascript
const { createApp } = require( 'vue' );
import App from './App.vue' ;
import createRouter from './router' ;
createApp(App).use(createRouter()).mount( '#app' );
|
Javascript
<!-- views/HomeView.vue file -->
<template>
<h1>This is Home Page</h1>
</template>
<script>
export default {}
</script>
|
Javascript
<!-- views/ContactView.vue file -->
<template>
<h1>This is Contact Page</h1>
</template>
<script>
export default {}
</script>
|
Javascript
<!-- views/AboutView.vue file -->
<template>
<h1>This is About Page</h1>
</template>
<script>
export default {};
</script>
|
Output:
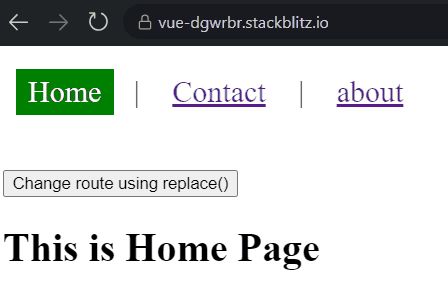
Conclusion
In Vue.js managing URL query parameters with Vue Router is a powerful feature that allows you to create dynamic and interactive web applications. Vue Router helps to work with query parameters, enabling developers to update the URL and component state based on user interactions.
Share your thoughts in the comments
Please Login to comment...