How to get Access to a Child Method From the Parent in VueJS ?
Last Updated :
18 Jan, 2024
When working with Vue.js components, it’s common to encounter scenarios where you need to access a method inside the parent component that is defined in a child component. This can be useful for triggering specific actions or updating the child component’s state.
Below are the methods that can be used to access a child component method from a parent component in VueJS.
Using $refs
This approach involves using the $refs property provided by Vue.js to create a reference to the child component. The parent component can then directly call methods on the child component using this reference.
Example 1: The below code uses $refs to access the child component method from the parent component in VueJS.
Javascript
<!-- ParentComponent.vue -->
<template>
<div>
<h2>Parent Component</h2>
<button @click= "callChildMethod" >
Call Child Method
</button>
<child-component ref= "childRef" />
</div>
</template>
<script>
import ChildComponent from
'./ChildComponent.vue' ;
export default {
components: {
ChildComponent,
},
methods: {
callChildMethod() {
const childComponent =
this .$refs.childRef;
if (childComponent) {
childComponent.childMethod();
} else {
console.error
( 'Child component reference is undefined.' );
}
},
},
};
</script>
|
Javascript
<!-- ChildComponent.vue -->
<template>
<div>
<h3>Child Component</h3>
</div>
</template>
<script>
export default {
methods: {
childMethod() {
console.log
( 'Child method called!' );
},
},
};
</script>
|
Javascript
<!-- App.vue -->
<template>
<div id= "app" >
<parent-component />
</div>
</template>
<script>
import ParentComponent from
'./ParentComponent.vue' ;
export default {
components: {
ParentComponent,
},
};
</script>
<style>
#app {
font-family:
Avenir, Helvetica, Arial, sans-serif;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
|
Output:
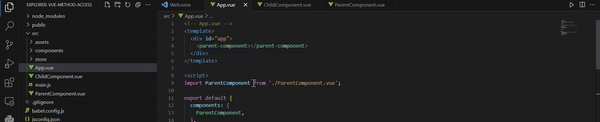
Example 2: The below example includes the advanced VueJS code to show some message on the user screen once the button is clicked and child method get accessed.
Javascript
<!-- ParentComponent.vue file -->
<template>
<div>
<h2>Parent Component</h2>
<button @click= "callChildMethod" >
Call Child Method
</button>
<!-- Display message with transition -->
<transition name= "fade" mode= "out-in" >
<div v- if = "message" class= "message" >
{{ message }}
</div>
</transition>
<child-component ref= "childRef" />
</div>
</template>
<script>
import ChildComponent from
'./ChildComponent.vue' ;
export default {
components: {
ChildComponent,
},
data() {
return {
message: '' ,
};
},
methods: {
callChildMethod() {
const childComponent = this .$refs.childRef;
if (childComponent) {
const result = childComponent.childMethod();
this .message = result;
} else {
console.error
( 'Child component reference is undefined.' );
}
},
},
};
</script>
<style>
.fade-enter-active,
.fade-leave-active {
transition: opacity 0.5s;
}
.fade-enter,
.fade-leave-to {
opacity: 0;
}
.message {
margin-top: 10px;
padding: 10px;
background-color: #4caf50;
color: #ffffff;
}
</style>
|
Javascript
<!-- ChildComponent.vue file -->
<template>
<div>
<h3>Child Component</h3>
</div>
</template>
<script>
export default {
methods: {
childMethod() {
console.log
( 'Child method called!' );
return
'Message from Child Component!' ;
},
},
};
</script>
|
Javascript
<!-- App.vue -->
<template>
<div id= "app" >
<parent-component />
</div>
</template>
<script>
import ParentComponent from
'./components/ParentComponent.vue' ;
export default {
components: {
ParentComponent
},
};
</script>
|
Output:
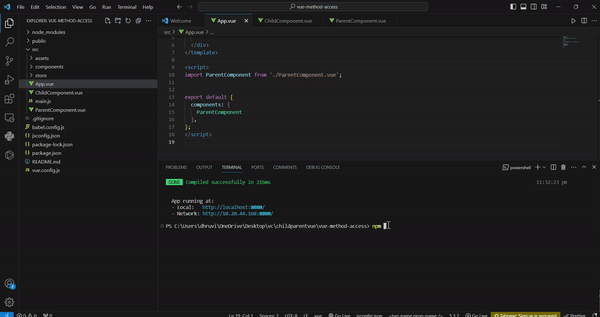
Using Custom Events
This approach involves using Vue.js custom events to establish communication between the parent and child components. The child component emits a custom event, and the parent component listens for that event, triggering the desired method.
Example: The below code uses custom events to access the child component method from the parent component in VueJS.
Javascript
<!-- ParentComponent.vue -->
<template>
<div>
<h2>Parent Component</h2>
<child-component
@child-method-triggered= "callChildMethod" />
</div>
</template>
<script>
import ChildComponent from
'./ChildComponent.vue' ;
export default {
components: {
ChildComponent,
},
methods: {
callChildMethod() {
console.log
( 'Child method called!' );
},
},
};
</script>
|
Javascript
<!-- ChildComponent.vue -->
<template>
<div>
<button @click= "triggerChildMethod" >
Call Child Method
</button>
<h3>Child Component</h3>
</div>
</template>
<script>
export default {
methods: {
triggerChildMethod() {
this .$emit
( 'child-method-triggered' );
},
},
};
</script>
|
Javascript
<!-- App.vue -->
<template>
<div id= "app" >
<parent-component/>
</div>
</template>
<script>
import ParentComponent from
'./components/ParentComponent.vue' ;
export default {
components: {
ParentComponent,
},
};
</script>
<script>
import { createApp } from 'vue' ;
import App from './App.vue' ;
createApp(App).mount( '#app' );
</script>
|
Output:
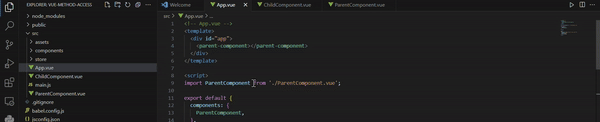
Share your thoughts in the comments
Please Login to comment...