POTD Solutions | 11 Nov’ 23 | Isomorphic Strings
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of String but will also help you build up problem-solving skills.
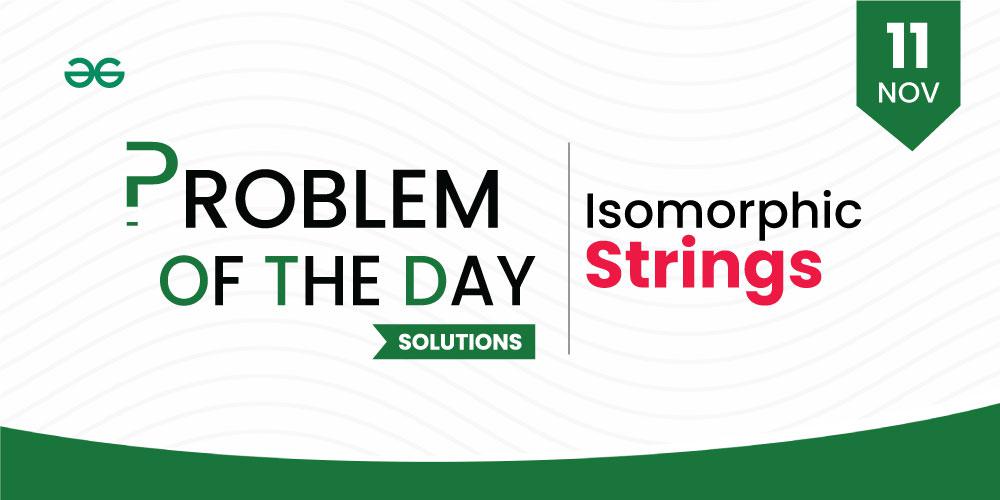
POTD Solution 11 November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 11 November: Isomorphic Strings
Given two strings ‘str1‘ and ‘str2‘, check if these two strings are isomorphic to each other.
If the characters in str1 can be changed to get str2, then two strings, str1 and str2, are isomorphic. A character must be completely swapped out for another character while maintaining the order of the characters. A character may map to itself, but no two characters may map to the same character.
Example:
Input: str1 = “aab”, str2 = “xxy”
Output: True
Explanation: ‘a’ is mapped to ‘x’ and ‘b’ is mapped to ‘y’.
Input: str1 = “aab”, str2 = “xyz”
Output: False
Explanation: One occurrence of ‘a’ in str1 has ‘x’ in str2 and other occurrence of ‘a’ has ‘y’.
The idea is to store map the character and check whether the mapping is correct or not.
Step-by-step approach:
- Create a hashmap of (char, char) to store the mapping of str1 and str2.
- Now traverse on the string and check whether the current character is present in the Hashmap.
- If it is present then the character that is mapped is there at the ith index or not.
- Else check if str2[i] is not present in the key then add the new mapping.
- Else return false.
Below is the implementation of the above approach:
C++
class Solution
{
public :
bool areIsomorphic(string str1, string str2)
{
int m = str1.length(), n = str2.length();
if (m != n)
return false ;
bool marked[MAX_CHARS] = { false };
int map[MAX_CHARS];
memset (map, -1, sizeof (map));
for ( int i = 0; i < n; i++)
{
if (map[str1[i]] == -1)
{
if (marked[str2[i]] == true )
return false ;
marked[str2[i]] = true ;
map[str1[i]] = str2[i];
}
else if (map[str1[i]] != str2[i])
return false ;
}
return true ;
}
};
|
Java
class Solution
{
public static boolean areIsomorphic(String str1,String str2)
{
int m = str1.length();
int n = str2.length();
if (m != n) {
return false ;
}
boolean [] marked = new boolean [ 256 ];
Map<Character, Character> map = new HashMap<>();
for ( int i = 0 ; i < n; i++) {
if (!map.containsKey(str1.charAt(i))) {
if (marked[str2.charAt(i)]) {
return false ;
}
marked[str2.charAt(i)] = true ;
map.put(str1.charAt(i), str2.charAt(i));
}
else if (map.get(str1.charAt(i)) != str2.charAt(i)) {
return false ;
}
}
return true ;
}
}
|
Python3
class Solution:
def areIsomorphic( self ,str1,str2):
m = len (str1)
n = len (str2)
if m ! = n:
return False
marked = set ()
mapping = {}
for i in range (n):
if str1[i] not in mapping:
if str2[i] in marked:
return False
marked.add(str2[i])
mapping[str1[i]] = str2[i]
elif mapping[str1[i]] ! = str2[i]:
return False
return True
|
Time Complexity: O(N) i.e. O(|N| + |N|), Traversing over the string of size N, where N is the length of the string.
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...