POTD Solutions | 19 Nov’ 23 | Intersection of two sorted linked lists
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Linked List but will also help you build up problem-solving skills.
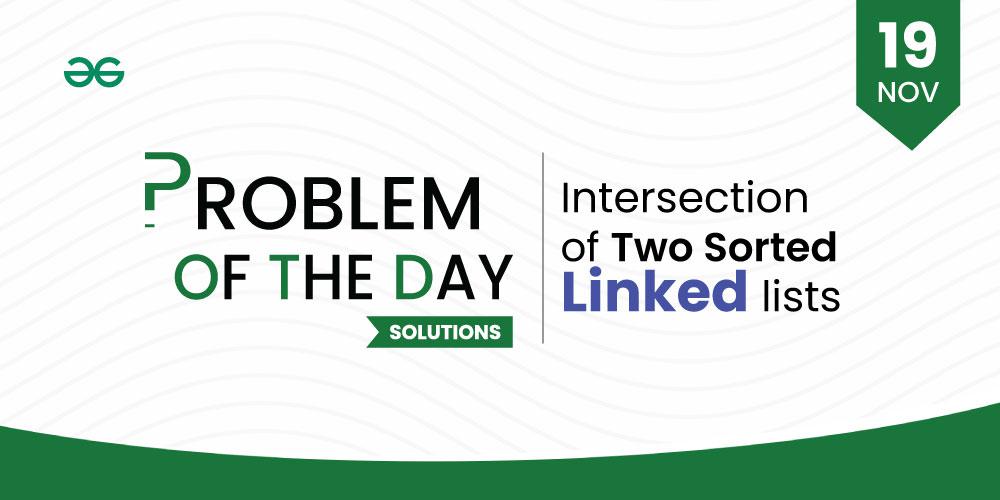
POTD Solution 19 November 2023
POTD 19 November: Intersection of two sorted linked lists:
Given two linked lists sorted in increasing order, create a new linked list representing the intersection of the two linked lists. The new linked list should be made with without changing the original lists.
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
Note: The elements of the linked list are not necessarily distinct.
Examples:
Input: LinkedList1 = 1->2->3->4->6, LinkedList2 = 2->4->6->8
Output: 2 4 6
Explanation: For the given two linked list, 2, 4 and 6 are the elements in the intersection.
Input: LinkedList1 = 10->20->40->50, LinkedList2 = 15->40
Output: 40
Explanation: For the given two linked list, 40 is the element in the intersection.
The idea is to use a temporary dummy node at the start of the result list. The pointer tail always points to the last node in the result list, so new nodes can be added easily. The dummy node initially gives the tail a memory space to point to. This dummy node is efficient, since it is only temporary, and it is allocated in the stack. The loop proceeds, removing one node from either ‘a’ or ‘b’ and adding it to the tail. When the given lists are traversed the result is in dummy. next, as the values are allocated from next node of the dummy. If both the elements are equal then remove both and insert the element to the tail. Else remove the smaller element among both the lists.
Below is the implementation of the above approach:
C++
class Solution {
public :
Node* findIntersection(Node* head1, Node* head2)
{
Node dummy(0);
Node* tail = &dummy;
dummy.next = NULL;
while (head1 != NULL && head2 != NULL) {
if (head1->data == head2->data) {
tail->next = new Node(head1->data);
tail = tail->next;
head1 = head1->next;
head2 = head2->next;
}
else if (head1->data < head2->data)
head1 = head1->next;
else
head2 = head2->next;
}
return (dummy.next);
}
};
|
Java
class Solution {
public static Node findIntersection(Node head1,
Node head2)
{
Node p = head1, q = head2;
Node tail = new Node( 0 );
Node dummy = tail;
while (p != null && q != null ) {
if (p.data == q.data) {
tail.next = new Node(p.data);
tail = tail.next;
p = p.next;
q = q.next;
}
else if (p.data < q.data)
p = p.next;
else
q = q.next;
}
return dummy.next;
}
}
|
Python3
class Solution:
def findIntersection( self , head1, head2):
dummy = Node( 0 )
tail = dummy
dummy. next = None
while (head1 ! = None and head2 ! = None ):
if (head1.data = = head2.data):
tail. next = Node(head1.data)
tail = tail. next
head1 = head1. next
head2 = head2. next
elif (head1.data < head2.data):
head1 = head1. next
else :
head2 = head2. next
return (dummy. next )
|
Time Complexity: O(m+n), where m and n are number of nodes in first and second linked lists respectively.
Auxiliary Space: O(min(m, n))
Share your thoughts in the comments
Please Login to comment...