POTD Solutions | 18 Nov’ 23 | Reverse a Doubly Linked List
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Linked Lists but will also help you build up problem-solving skills.
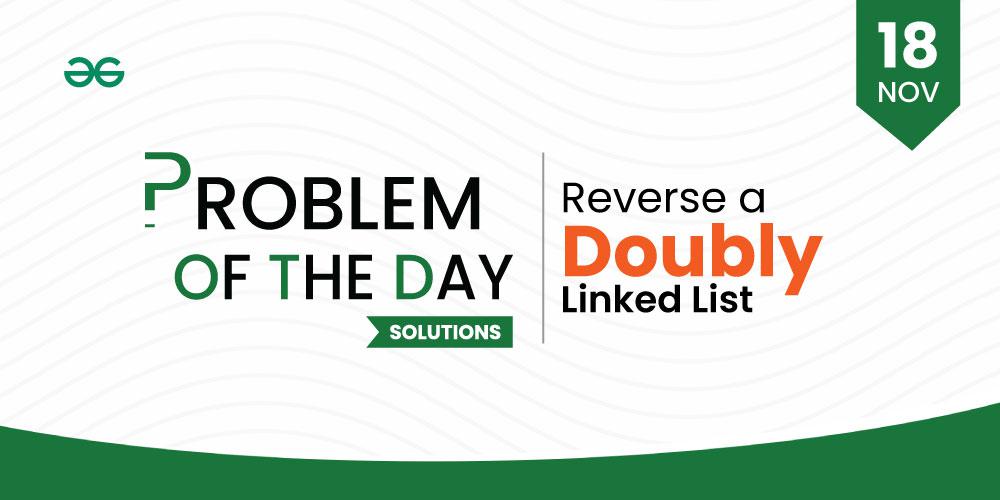
POTD Solution 18 November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 18 November: Reverse a Doubly Linked List:
Given a doubly linked list of n elements. Your task is to reverse the doubly linked list in-place.
Examples:
Input: LinkedList: 3 <–> 4 <–> 5
Output: 5 <–> 4 <–> 3
Input: LinkedList: 75 <–> 122 <–> 59 <–> 196
Output: 196 <–> 59 <–> 122 <–> 75
The idea is to use an iterative approach. We can modify the prev and next pointers of each node to reverse the direction of the links.
Step-by-step approach:
- Initialize two pointers, curr and prev. Initially, curr points to the head of the list, and prev is set to null.
- Enter a loop that iterates through the entire list. Inside the loop:
- Update the curr node’s prev pointer to its next pointer, effectively reversing the direction of the link.
- Update the curr node’s next pointer to prev, again reversing the direction of the link.
- Essentially, the prev and next pointers are swapped to reverse the node’s connections in the DLL.
- Once the loop is complete, the curr pointer will be pointing to the new head of the reversed list, and the prev pointer will be pointing to the node that was originally at the end of the list (now the new tail). Return prev to indicate the new head of the reversed DLL.
Below is the implementation of the above approach:
C++
class Solution
{
public :
Node* reverseDLL(Node * head)
{
if (head == NULL || head->next == NULL)
return head;
Node *prev = NULL, *curr = head;
while (curr != NULL){
prev = curr->prev;
curr->prev = curr->next;
curr->next = prev;
curr = curr->prev;
}
return prev->prev;
}
};
|
Java
public static Node reverseDLL(Node head)
{
if (head == null || head.next == null )
return head;
Node curr = head, prev = null ;
while (curr != null ){
prev = curr.prev;
curr.prev = curr.next;
curr.next = prev;
curr = curr.prev;
}
return prev.prev;
}
|
Python3
class Solution:
def reverseDLL( self , head):
temp = None
current = head
while current is not None :
temp = current.prev
current.prev = current. next
current. next = temp
current = current.prev
if temp is not None :
head = temp.prev
return head
|
Time Complexity : O(N), where N is the number of nodes in the doubly linked list.
Auxiliary Space: O(1), constant space
Share your thoughts in the comments
Please Login to comment...