POTD Solutions | 13 Nov’ 23 | Shortest Common Supersequence
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Dynamic Programming but will also help you build up problem-solving skills.
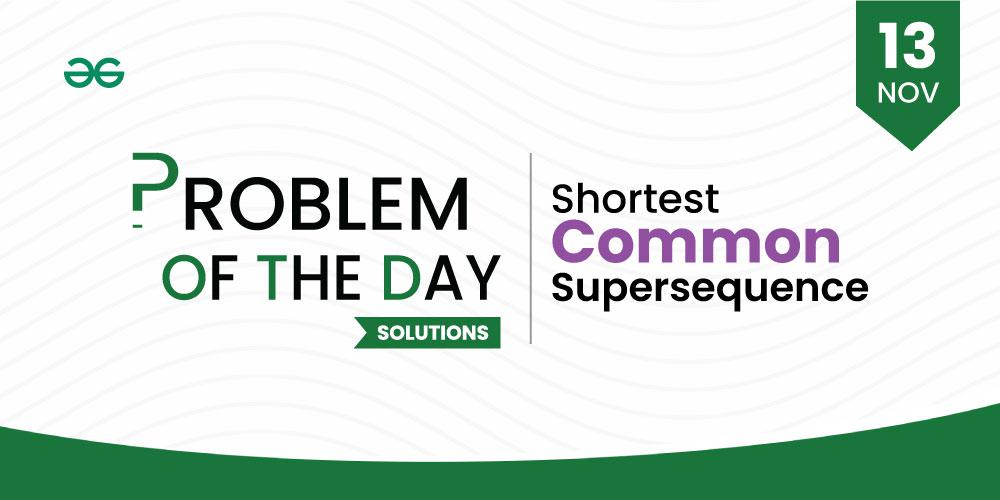
POTD Solution 13 November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 13 November: Shortest Common Supersequence
Given two strings X and Y of lengths m and n respectively, find the length of the smallest string that has both, X and Y as its sub-sequences.
Note: X and Y can have both uppercase and lowercase letters.
Examples:
Input: X = “abcd”, Y = “xycd”
Output: 6
Explanation: Shortest Common Supersequence would be abxycd which is of length 6 and has both the strings as its subsequences.
Input: X = “efgh”, Y = “jghi”
Output: 6
Explanation: Shortest Common Supersequence would be ejfghi which is of length 6 and has both the strings as its subsequences.
We need to find a string that has both strings as subsequences and is the shortest such string. If both strings have all characters different, then result is sum of lengths of two given strings. If there are common characters, then we don’t want them multiple times as the task is to minimize length. Therefore, we first find the longest common subsequence, take one occurrence of this subsequence and add extra characters.
Length of the shortest supersequence = (Sum of lengths of given two strings) – (Length of LCS of two given strings)
Below is the implementation of the above approach:
C++
class Solution {
public :
int lcs(string& X, string& Y, int m, int n)
{
int L[m + 1][n + 1];
int i, j;
for (i = 0; i <= m; i++) {
for (j = 0; j <= n; j++) {
if (i == 0 || j == 0)
L[i][j] = 0;
else if (X[i - 1] == Y[j - 1])
L[i][j] = L[i - 1][j - 1] + 1;
else
L[i][j] = max(L[i - 1][j], L[i][j - 1]);
}
}
return L[m][n];
}
int shortestCommonSupersequence(string X, string Y,
int m, int n)
{
int l = lcs(X, Y, m, n);
return (m + n - l);
}
};
|
Java
class Solution {
static int lcs(String X, String Y, int m, int n)
{
int [][] L = new int [m + 1 ][n + 1 ];
int i, j;
for (i = 0 ; i <= m; i++) {
for (j = 0 ; j <= n; j++) {
if (i == 0 || j == 0 )
L[i][j] = 0 ;
else if (X.charAt(i - 1 ) == Y.charAt(j - 1 ))
L[i][j] = L[i - 1 ][j - 1 ] + 1 ;
else
L[i][j] = Math.max(L[i - 1 ][j],
L[i][j - 1 ]);
}
}
return L[m][n];
}
public static int shortestCommonSupersequence(String X,
String Y,
int m,
int n)
{
int l = lcs(X, Y, m, n);
return (m + n - l);
}
}
|
Python3
def lcs(X, Y, m, n):
L = [[ 0 ] * (n + 2 ) for i in
range (m + 2 )]
for i in range (m + 1 ):
for j in range (n + 1 ):
if (i = = 0 or j = = 0 ):
L[i][j] = 0
elif (X[i - 1 ] = = Y[j - 1 ]):
L[i][j] = L[i - 1 ][j - 1 ] + 1
else :
L[i][j] = max (L[i - 1 ][j],
L[i][j - 1 ])
return L[m][n]
|
C#
class Solution {
static int lcs(String X, String Y, int m, int n)
{
int [, ] L = new int [m + 1, n + 1];
int i, j;
for (i = 0; i <= m; i++) {
for (j = 0; j <= n; j++) {
if (i == 0 || j == 0)
L[i, j] = 0;
else if (X[i - 1] == Y[j - 1])
L[i, j] = L[i - 1, j - 1] + 1;
else
L[i, j] = Math.Max(L[i - 1, j],
L[i, j - 1]);
}
}
return L[m, n];
}
public int shortestCommonSupersequence( string x,
string y, int m,
int n)
{
int l = lcs(x, y, m, n);
return (m + n - l);
}
}
|
Javascript
class Solution
{
shortestCommonSupersequence(X, Y, m, n)
{
let dp = new Array(m + 1).fill(0).map(() => new Array(n + 1).fill(0));
for (let i = 0; i <= m; i++) {
for (let j = 0; j <= n; j++) {
if (i === 0)
dp[i][j] = j;
else if (j === 0)
dp[i][j] = i;
else if (X[i - 1] === Y[j - 1])
dp[i][j] = 1 + dp[i - 1][j - 1];
else
dp[i][j] = 1 + Math.min(dp[i - 1][j], dp[i][j - 1]);
}
}
return dp[m][n];
}
}
|
Time Complexity: O(|X| * |Y|), where |X| is the length of string X and |Y| is the length of string Y.
Auxiliary Space: O(|X| * |Y|)
Share your thoughts in the comments
Please Login to comment...