POTD Solutions | 10 Nov’ 23 | Number following a pattern
Last Updated :
11 Nov, 2023
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Stack and Two Pointer Algorithm but will also help you build up problem-solving skills.
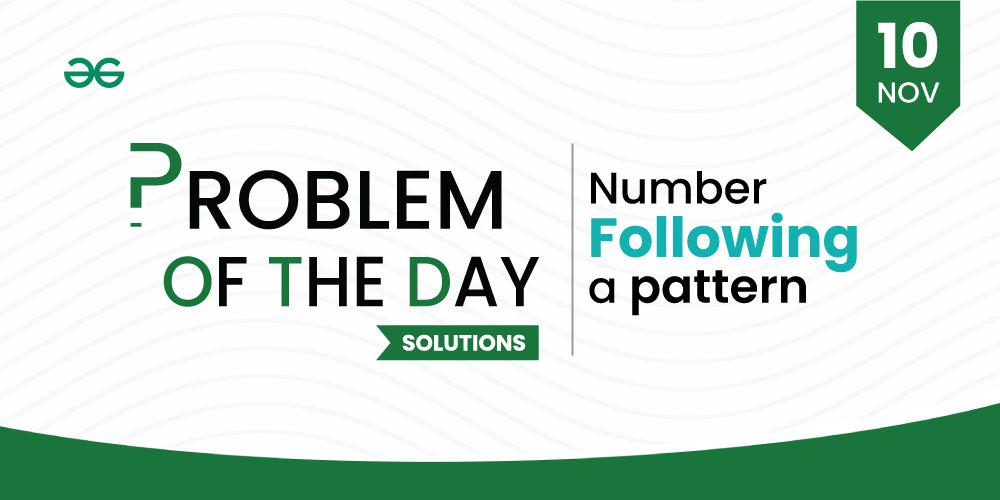
POTD Solutions 10 November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 10 November: Number following a pattern
Given a pattern containing only I‘s and D‘s. I for increasing and D for decreasing. Devise an algorithm to print the minimum number following that pattern. Digits from 1-9 and digits can’t repeat.
Examples:
Input: D
Output: 21
Input: I
Output: 12
Input: DD
Output: 321
We can that when we encounter I, we got numbers in increasing order but if we encounter ‘D’, we want to have numbers in decreasing order. Length of the output string is always one more than the input string. So the loop is from 0 to the length of the string. We have to take numbers from 1-9 so we always push (i+1) to our stack. Then we check what is the resulting character at the specified index.So, there will be two cases which are as follows:
Case 1: If we have encountered I or we are at the last character of input string, then pop from the stack and add it to the end of the output string until the stack gets empty.
Case 2: If we have encountered D, then we want the numbers in decreasing order. so we just push (i+1) to our stack.
Below is the implmentation of the above approach:
C++
class Solution{
public :
string printMinNumberForPattern(string seq){
string result;
stack< int > stk;
for ( int i = 0; i <= seq.length(); i++)
{
stk.push(i + 1);
if (i == seq.length() || seq[i] == 'I' )
{
while (!stk.empty())
{
result += to_string(stk.top());
stk.pop();
}
}
}
return result ;
}
};
|
Java
class Solution{
static String printMinNumberForPattern(String seq){
StringBuilder result = new StringBuilder();
Stack<Integer> stk = new Stack<>();
for ( int i = 0 ; i <= seq.length(); i++) {
stk.push(i + 1 );
if (i == seq.length() || seq.charAt(i) == 'I' ) {
while (!stk.isEmpty()) {
result.append(stk.pop());
}
}
}
return result.toString();
}
}
|
Python3
class Solution:
def printMinNumberForPattern(ob,seq):
result = []
stk = []
for i in range ( len (seq) + 1 ):
stk.append(i + 1 )
if i = = len (seq) or seq[i] = = 'I' :
while stk:
result.append( str (stk.pop()))
return ''.join(result)
|
Time Complexity: O(n)
Auxiliary Space: O(n), since n extra space has been taken.
- Since we have to find a minimum number without repeating digits, maximum length of output can be 9 (using each 1-9 digits once)
- Length of the output will be exactly one greater than input length.
- The idea is to iterate over the string and do the following if current character is ‘I’ or string is ended.
- Assign count in increasing order to each element from current-1 to the next left index of ‘I’ (or starting index is reached).
- Increase the count by 1.
Below is the implmentation of the above approach:
C++
class Solution{
public :
string printMinNumberForPattern(string seq){
int n = seq.length();
if (n >= 9)
return "-1" ;
string result(n+1, ' ' );
int count = 1;
for ( int i = 0; i <= n; i++)
{
if (i == n || seq[i] == 'I' )
{
for ( int j = i - 1 ; j >= -1 ; j--)
{
result[j + 1] = '0' + count++;
if (j >= 0 && seq[j] == 'I' )
break ;
}
}
}
return result;
}
};
|
Java
class Solution{
static String printMinNumberForPattern(String seq){
int n = seq.length();
if (n >= 9 ) {
return "-1" ;
}
char [] result = new char [n + 1 ];
int count = 1 ;
for ( int i = 0 ; i <= n; i++) {
if (i == n || seq.charAt(i) == 'I' ) {
for ( int j = i - 1 ; j >= - 1 ; j--) {
result[j + 1 ] = ( char ) ( '0' + count++);
if (j >= 0 && seq.charAt(j) == 'I' ) {
break ;
}
}
}
}
return new String(result);
}
}
|
Python3
class Solution:
def printMinNumberForPattern(ob,seq):
n = len (seq)
if n > = 9 :
return "-1"
result = [ ' ' ] * (n + 1 )
count = 1
for i in range (n + 1 ):
if i = = n or seq[i] = = 'I' :
j = i - 1
while j > = - 1 :
result[j + 1 ] = str (count)
count + = 1
if j > = 0 and seq[j] = = 'I' :
break
j - = 1
return ''.join(result)
|
Time Complexity: O(N)
Auxiliary Space: O(N), since N extra space has been taken.
Share your thoughts in the comments
Please Login to comment...