Object Level Lock vs Class Level Lock in Java
Last Updated :
19 Apr, 2022
Synchronized is the modifier applicable only for methods and blocks but not for classes and variables. If multiple threads are trying to operate simultaneously on the same java object then there may be a chance of data inconsistency problem to overcome this problem we should go for a ‘synchronized‘ keyword. If a method or block declares as synchronized then at a time only one thread is allowed to execute that method or block on the given object so that the data inconsistency problem will be resolved. Internally synchronization concept is implemented by using the lock. Every object in java has a unique lock. Whenever we are using a ‘synchronized‘ keyword then only the lock concept will come into the picture.
If a thread want’s to execute a synchronized method on the given object first it has to get a lock of that object once the thread got the lock then it is allowed to execute any synchronized method on that object. Acquiring and releasing lock internally takes care of by JVM and the programmer is not responsible for this activity. While a thread executing a synchronized method on the given object the remaining threads are not allowed to execute any synchronized method simultaneously on the same object. But remaining threads are allowed to execute non-synchronized methods simultaneously.
Object Level Lock is a mechanism where every object in java has a unique lock which is nothing but an object–level lock. If a thread wants to execute a synchronized method on the given object then the thread first required the object level lock once the thread gets object level lock then it is allowed to execute any synchronized method on the given object and once the method execution completed automatically thread releases the lock of that object.
Example:
Java
import java.io.*;
import java.util.*;
class Display {
public void wish(String name)
{
synchronized ( this )
{
for ( int i = 1 ; i <= 10 ; i++) {
System.out.print( "Good Morning: " );
try {
Thread.sleep( 1000 );
}
catch (InterruptedException e) {
System.out.println(e);
}
System.out.println(name);
}
}
}
}
class myThread extends Thread {
Display d;
String name;
myThread(Display d, String name)
{
this .d = d;
this .name = name;
}
public void run()
{
d.wish(name);
}
}
class GFG {
public static void main(String[] args)
{
Display d = new Display();
myThread t1 = new myThread(d, "Dhoni" );
myThread t2 = new myThread(d, "Yuvraj" );
t1.start();
t2.start();
}
}
|
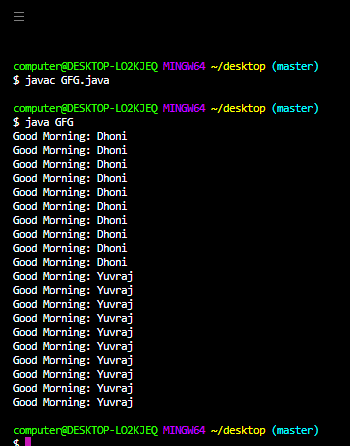
Class Level Lock is a mechanism where every class in java has a unique lock which is nothing but a class level lock. If a thread wants to execute a static synchronized method then the thread requires a class level lock once the thread gets a class level lock then it is allowed to execute any static synchronized method of that class. Once method execution completes automatically thread releases the lock. While a thread executing a static synchronized method the remaining thread is not allowed to execute any static synchronized method of that class simultaneously.
Example:
Java
import java.io.*;
import java.util.*;
class Display {
public static void wish(String name)
{
synchronized (Display. class )
{
for ( int i = 1 ; i <= 10 ; i++) {
System.out.print( "Good Morning: " );
try {
Thread.sleep( 1000 );
}
catch (InterruptedException e) {
System.out.println(e);
}
System.out.println(name);
}
}
}
}
class myThread extends Thread {
Display d;
String name;
myThread(Display d, String name)
{
this .d = d;
this .name = name;
}
public void run()
{
d.wish(name);
}
}
class GFG {
public static void main(String[] args)
{
Display d = new Display();
myThread t1 = new myThread(d, "Dhoni" );
myThread t2 = new myThread(d, "Yuvraj" );
t1.start();
t2.start();
}
}
|
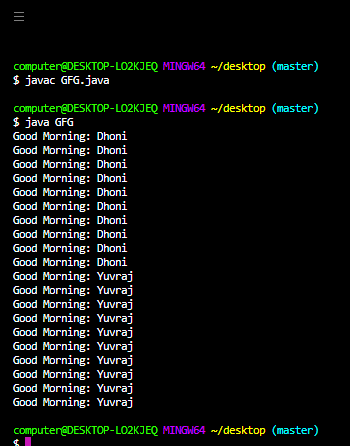
Class Level Lock
|
Object Level Lock
|
This lock can be used when we want to prevent multiple threads to enter the synchronized block of available instances on runtime. |
This lock is used when we want a non-static method or non-static block of our code should be accessed by only one thread at a time. |
This lock is used to make static data thread-safe. |
This lock is used to make non-static data thread-safe. |
Multiple objects of a particular class may exist but there is always one class’s class object lock available. |
Every object the class have their own lock. |
We can get a class level lock as follows:
public class GFG {
public void m1( ) {
synchronized (GFG.class) {
// some line of code
}
}
|
We can get object level lock as follows:
public class GFG {
public void m1( ) {
synchronized (this) {
// some line of code
}
}
}
|
Share your thoughts in the comments
Please Login to comment...