Sealed Class in Java
Last Updated :
09 Jan, 2024
In Java, we have the concept of abstract classes. It is mandatory to inherit from these classes since objects of these classes cannot be instantiated. On the other hand, there is a concept of a final class in Java, which cannot be inherited or extended by any other class. What if we want to restrict the number of classes that can inherit from a particular class? The answer is sealed class. So, a sealed class is a technique that limits the number of classes that can inherit the given class. This means that only the classes designated by the programmer can inherit from that particular class, thereby restricting access to it. when a class is declared sealed, the programmer must specify the list of classes that can inherit it. The concept of sealed classes in Java was introduced in Java 15.
Steps to Create a Sealed Class
- Define the class that you want to make a seal.
- Add the “sealed” keyword to the class and specify which classes are permitted to inherit it by using the “permits” keyword.
Example
sealed class Human permits Manish, Vartika, Anjali
{
public void printName()
{
System.out.println("Default");
}
}
non-sealed class Manish extends Human
{
public void printName()
{
System.out.println("Manish Sharma");
}
}
sealed class Vartika extends Human
{
public void printName()
{
System.out.println("Vartika Dadheech");
}
}
final class Anjali extends Human
{
public void printName()
{
System.out.println("Anjali Sharma");
}
}
Explanation of the above Example:
- Human is the parent class of Manish, Vartika, and Anjali. It is a sealed class so; other classes cannot inherit it.
- Manish, Vartika, and Anjali are child classes of the Human class, and it is necessary to make them either sealed, non-sealed, or final. Child classes of a sealed class must be sealed, non-sealed, or final.
- If any class other than Manish, Vartika, and Anjali tries to inherit from the Human class, it will cause a compiler error.
Code Implementation
Java
import java.lang.*;
sealed class Human permits Manish, Vartika, Anjali
{
public void printName()
{
System.out.println( "Default" );
}
}
non-sealed class Manish extends Human
{
public void printName()
{
System.out.println( "Manish Sharma" );
}
}
sealed class Vartika extends Human
{
public void printName()
{
System.out.println( "Vartika Dadheech" );
}
}
final class Anjali extends Human
{
public void printName()
{
System.out.println( "Anjali Sharma" );
}
}
public class Main
{
public static void main(String[] args)
{
Human h1 = new Anjali();
Human h2 = new Vartika();
Human h3 = new Manish();
h1.printName();
h2.printName();
h3.printName();
}
}
|
Output:Â
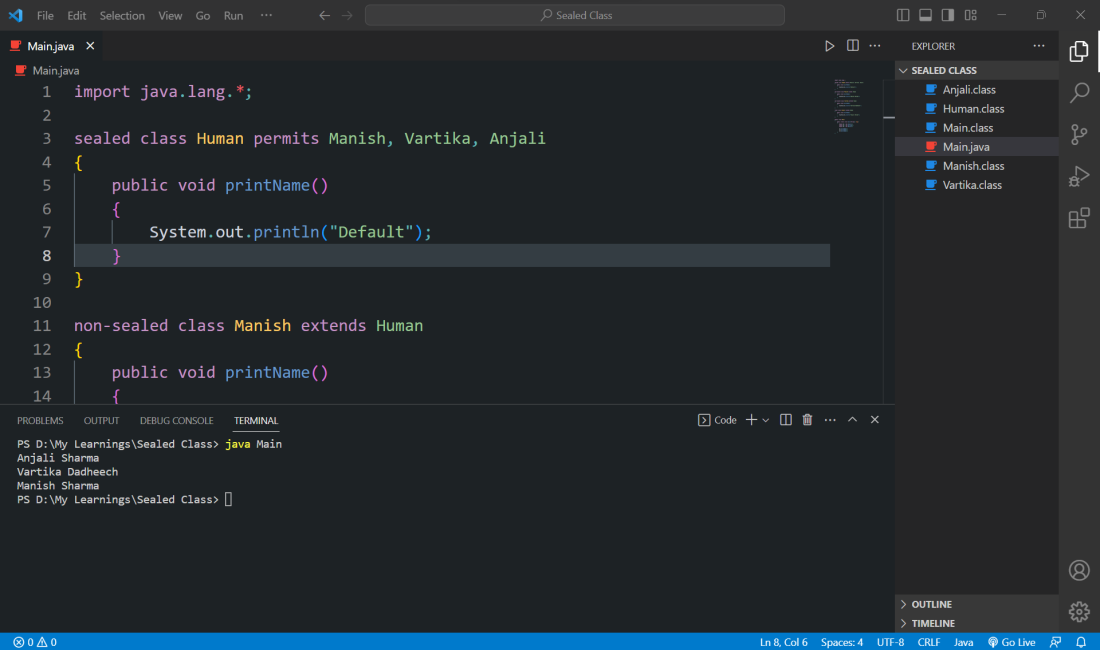
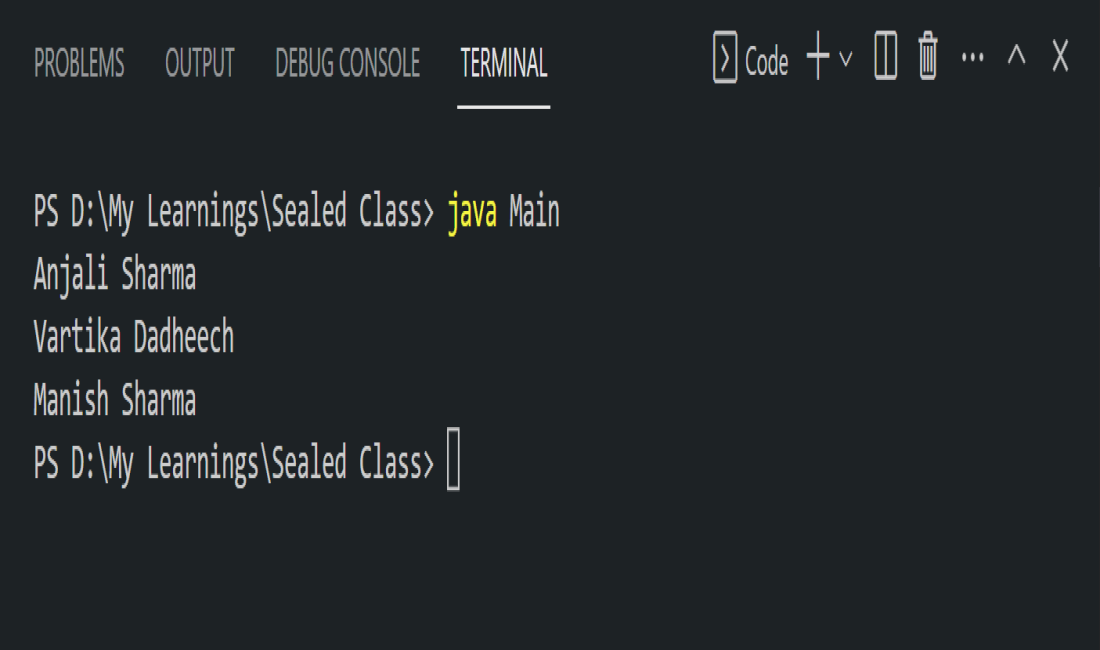
Share your thoughts in the comments
Please Login to comment...