Locating Strategies By ID Using Java
Last Updated :
16 Apr, 2024
Here we are going to discuss locating a particular web element using the value of its id
Steps for Locating Strategies By ID Using Java
Step1:Create a Java Project In Eclipse IDE
Launch Eclipse IDE and create a new project by navigating
File –>New –>Java Project
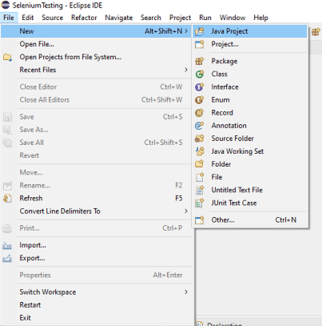
Creating new project
Step 2: Name The Java Project
A new window will be open to prompting to enter a Project Name. Provide a meaningful name
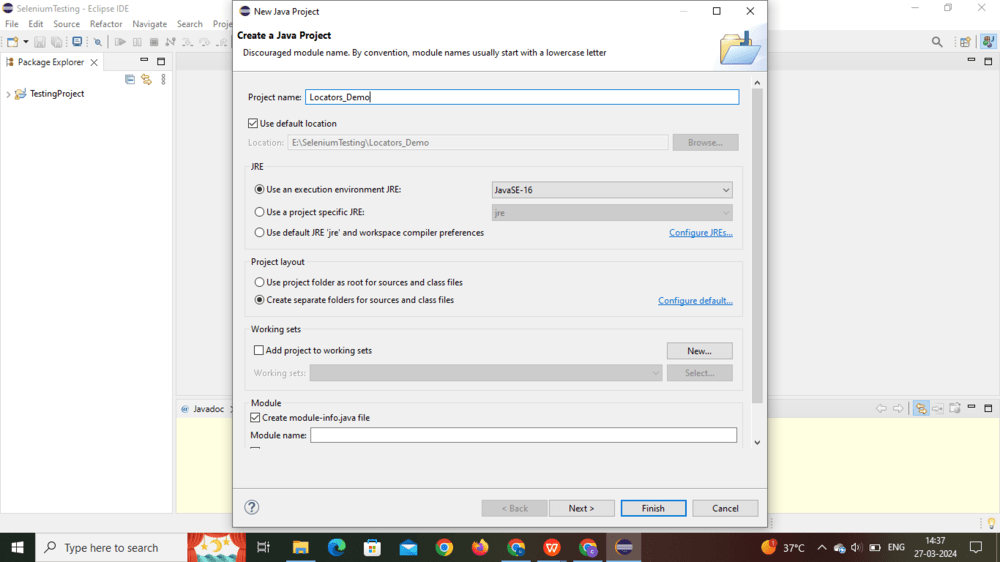
project name Locators_Demo
Step 3:Download Selenium and Configure Build Path
A new project is created with the name Locators_Demo. Then right-click on a project to configure selenium jar files with IDE.
before you should download Selenium WebDriver for Java.
link: Selenium WebDriver
Right-click on project–> Build Path –>Configure Build Path –>Class Path–>Add External Jars –>Select All Jars–>Apply And Close
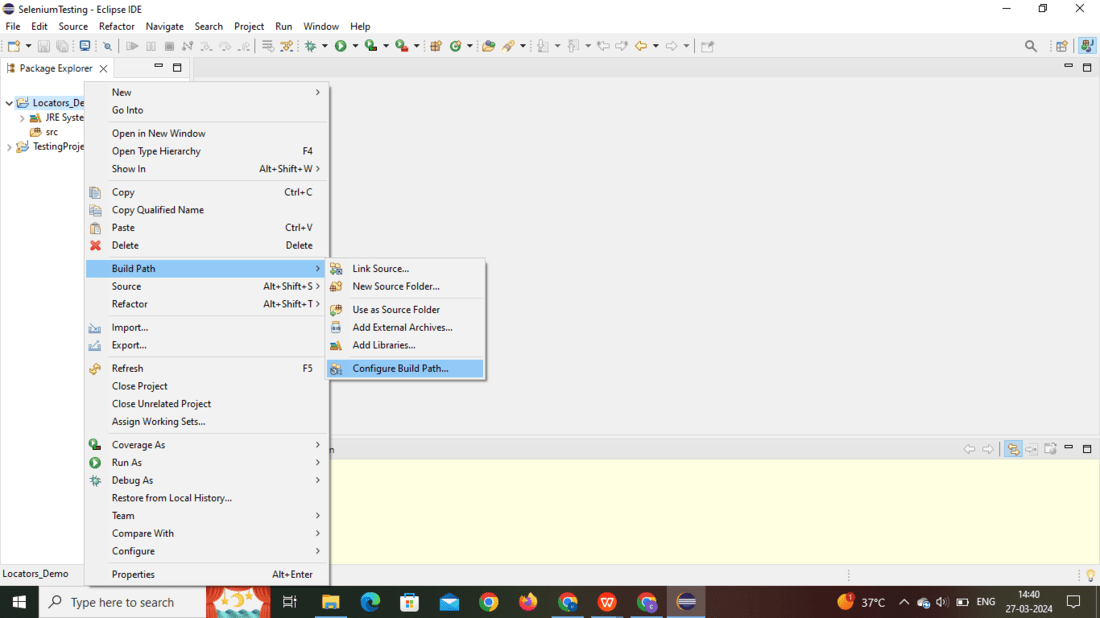
Configure Build Path
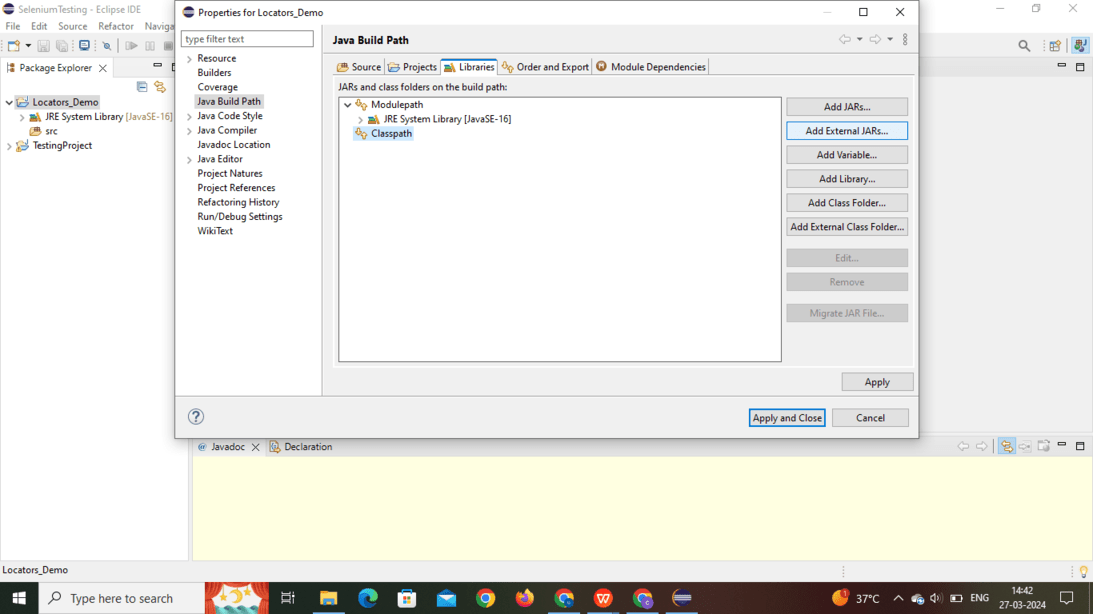
Add External Jars
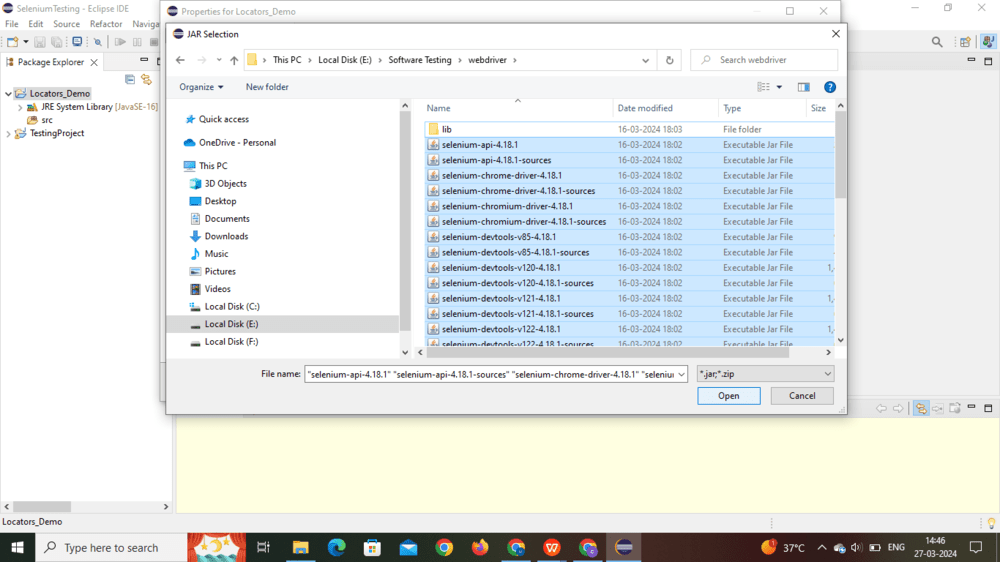
Add External Jars
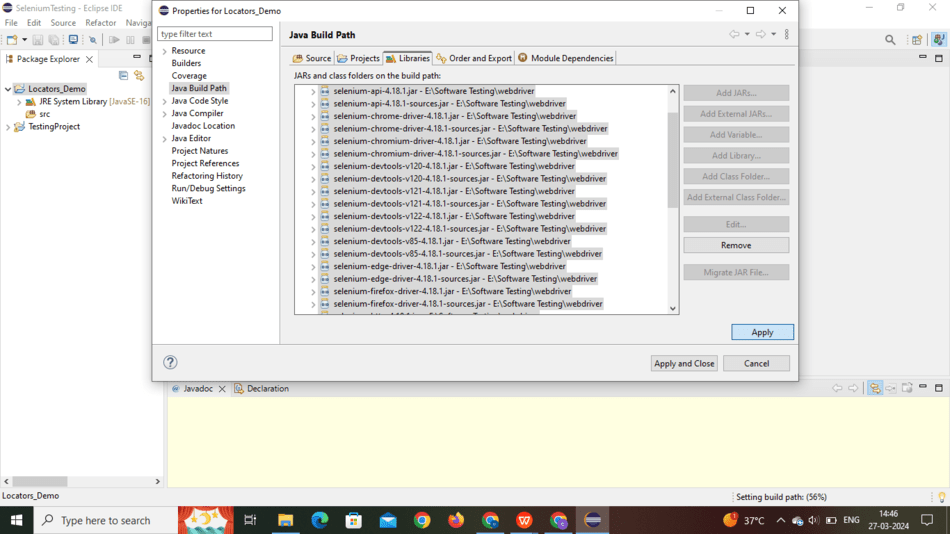
Add External Jars
Step 4: Download Chrome Driver
Right-click on Project to create a new folder to store the Chrome driver
Download a compatible version of Chrome driver for your Chrome browser then extract the files into the Chrome driver folder.
Chrome Driver Download Link: Chrome Driver
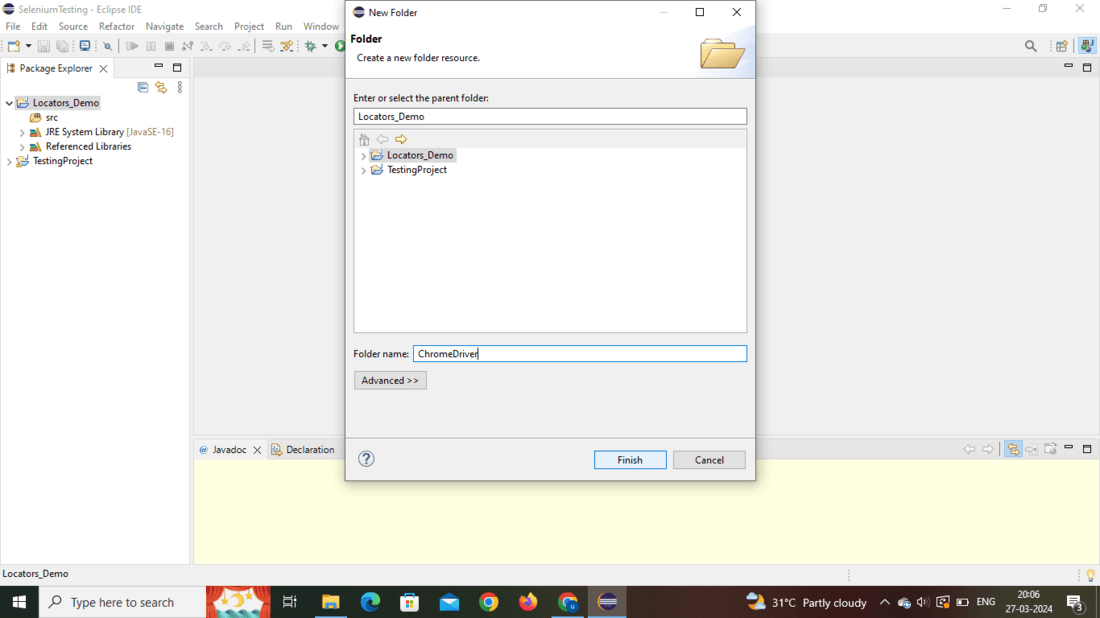
Create a folder
Step 5 Create a New Class File
Right-click on the src folder then create a package name as com. selenium. Test under this package create one class name as ByIdDemo the click on finish. Then the project folder structure is shown as the below pictures.
.png)
Create Class
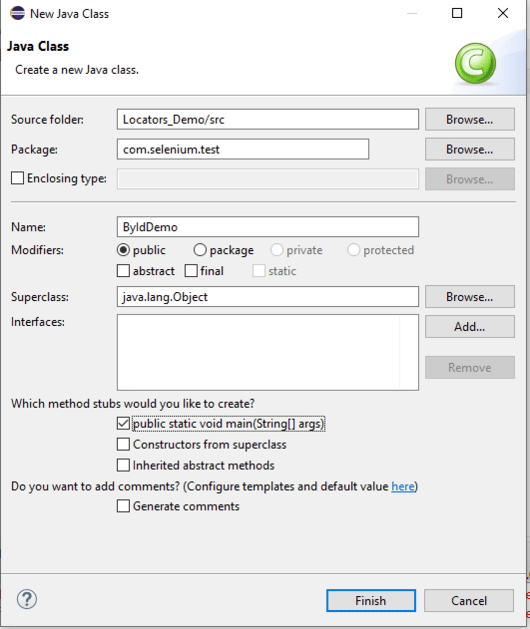
ByIdDemo.java
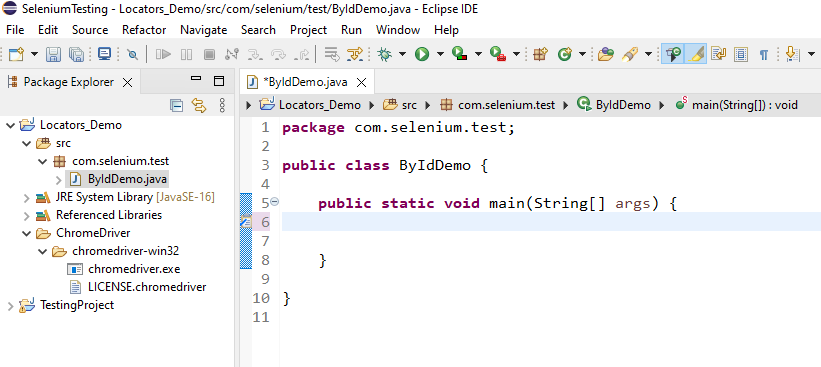
Step 6: Selenium WebDriver Coding
Java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
public class ByIdDemo {
public static void main(String[] args) {
//System property for chrome driver
System.setProperty("webdriver.chrome.driver","./ChromeDriver/chromedriver-win32/chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.facebook.com/");
driver.findElement(By.id("email")).sendKeys("abc@gmail.com");
driver.findElement(By.id("pass")).sendKeys("12345");
driver.findElement(By.name("login")).click();
}
}
.png)
Final test case
Step 7: Run The Java Project
Right-click on the class then select Run as Java Application
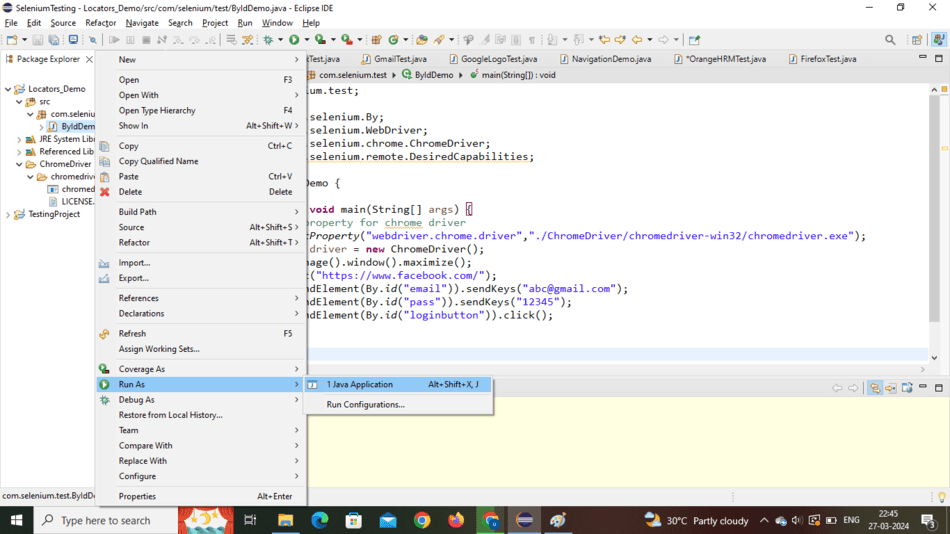
Run As Java Application
Output :
Face Facebook login page will automated by the opening typing email and password and then clicking on the login button.
.png)
Conclusion
In conclusion, Id is a locator which is present under the By class. Id locator which is unique for every element in the DOM page that’s why an ID can uniquely identify an element.
Frequently Asked Questions based on Locating Strategies By ID Using Java:
What is Selenium?
Ans: Selenium is a free and open-source web application automation tool. Also, we can call it a Functional Testing web application automation tool. Not used for window-based applications.
- It will support multiple browsers
- It will support different operating systems
- and also supports multiple languages
What are The Locators?
Ans: Locators are used to identify the web elements. In Selenium if we want to perform any action like typing in a textbox, or clicking buttons before these actions we should find the element using locators. In Selenium there are eight types of locators. All of them are static methods in By class. All the methods take a string as an argument and it returns an object of type By. The By object is used as an input argument for the findElement() method. The return type of the findElement() method is WebElement (I).
List Of Locators:
By.tagName
- By.id
- By.name
- By.className
- By.linkText
- By.partialTextLink
- By.cssSelector
- By.xpath
If an ID is not there for a particular web element what is the solution?
Ans: Id, name, and class name are available as attributes of an element to handle the single element we use findElement(). return type of findElement() is WebElement. In findElement(), if the specified locator does not match with any element it will throw NoSuchElementException. Or if the specified locator is matching with multiple elements it will return the address of the first matching element. If we can not identify the element by using any of the above locators, then we can identify that element by using cssSelector.
Share your thoughts in the comments
Please Login to comment...