Selenium is one of the most powerful and widely used tools for automated web applications. Whether you’re a software developer or a QA tester selenium is an important tool to have in your toolkit. One of the most important tasks in order to automate the web applications is interacting with the elements on a web page that’s where Selenium Locating strategies come into play. Selenium Locating Strategies are a set of methods used to precisely locate and interact with web elements on a web page. These web elements can be anything from buttons, checkboxes, and input fields to complex elements like tables and iframes. Locating the element accurately is a crucial step in automating user interactions effectively such as filling out forms, clicking buttons, etc.
What are Locating Strategies in Selenium?
Selenium Locating strategies are a set of methods that we can use to precisely locate the target web element on a webpage. Locating the target web element is a crucial step in automating user interactions on a web page. Selenium offers 7 locating strategies that we can use to locate the target web element precisely on a web page.
Here are the 7 locating strategies:
- Locating By ID.
- Locating By Class Name.
- Locating By Name.
- Locating By Tag Name
- Locating By CSS Selector.
- Locating By XPath.
- Locating By Link Text And Partial Link Text.
1. Locating By ID
The ID attribute of an HTML element is a unique identifies , the id attribute is assigned to a specific element and should be unique on a web page and this makes it an ideal choice for locating element because it guarantees that you’re targeting the exact element you intend to interact with.
- Searching for an element using its ID is generally faster than other methods such as XPATH or CSS Selector because it leverages native browser functions optimized for this purpose.
- To locate an element by ID we use By.ID locator provided by the By class.
- By is a class that provides a set of locating strategies to locate web elements on a web page.
Syntax:
element=driver.find_element(By.ID, “element_id”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.ID: This specifies that you’re locating the element by its ID.
element_id: Replace this with the actual id of the element you want to locate.
Below is the Python program to implement Locating by ID:
Python
# Python program to implement Locating by ID
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace this with your actual url
url = "https://www.geeksforgeeks.org/data-structures/?ref=shm"
driver.get(url)
# Replace ID_NAME with the Id of your element
try:
# Replace this with the id of the element
element = driver.find_element(By.ID, "nav_tab_courses")
except NoSuchElementException:
print("Not Found")
else:
print("Found")
Output:
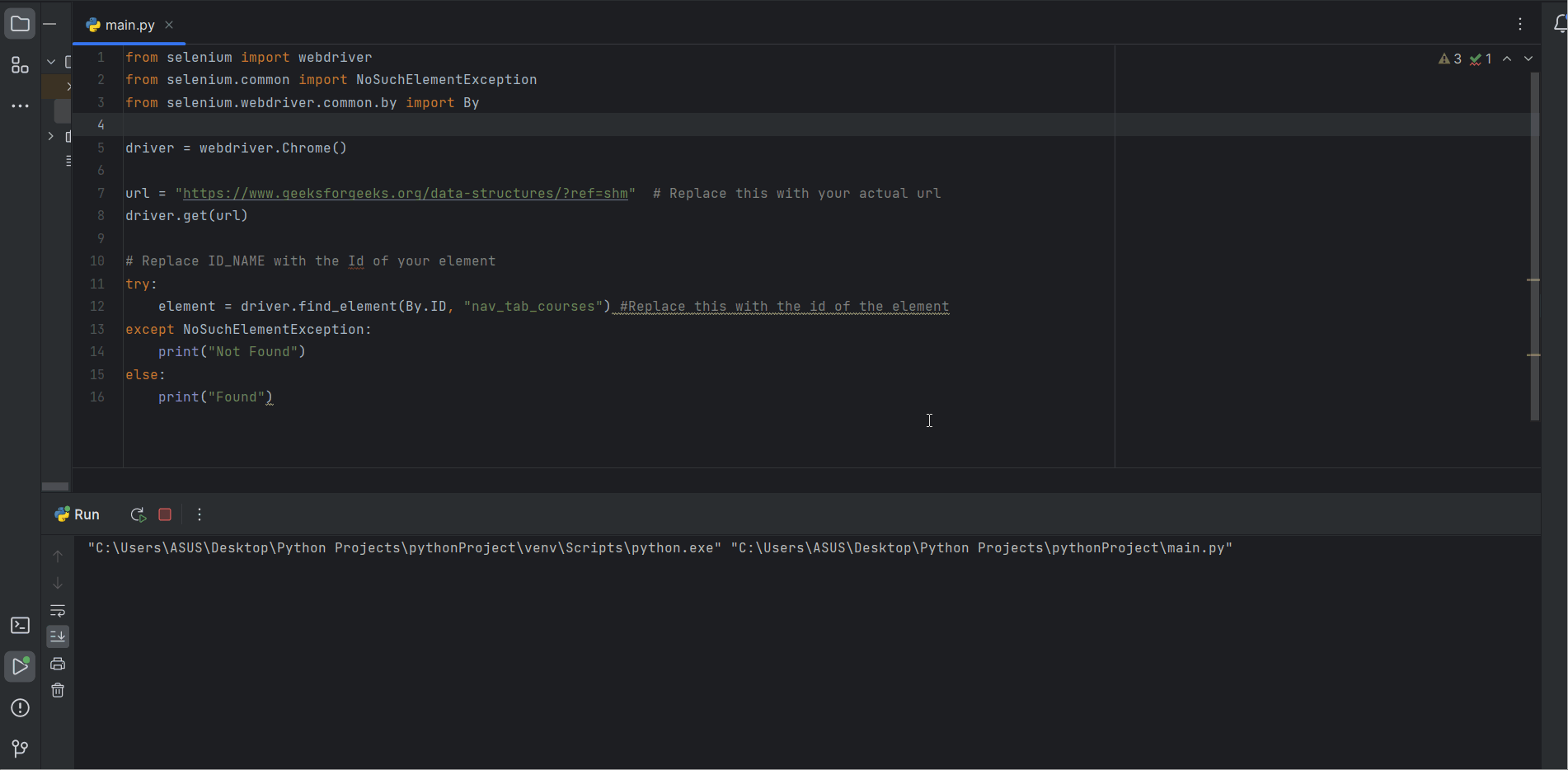
Selenium Locating Strategies
2. Locating By Class Name
The class attribute is one of the most commonly used in attribute in HTML .The class attribute allows multiple elements on a webpage to share a common styling. While classes can be shares with other elements , each element class value should be unique within its scope. This uniqueness makes it a right choice for locating elements on a web page.
- To locate an element by CLASS we use By.CLASS_NAME locator provided by the By class.
- By is a class that provides a set of locating strategies to locate web elements on a web page.
Syntax:
element=driver.find_element(By.CLASS_NAME, “element_class_name”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.CLASS_NAME: This specifies that you’re locating the element by its class.
element_class_name: Replace this with the actual class of the element you want to locate.
Below is the Python program to implement Locating by Class Name:
Python
# Python program to implement Locating by Class Name
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace this with your actual url
url = "https://www.geeksforgeeks.org/data-structures/?ref=shm"
driver.get(url)
# Replace ID_NAME with the Id of your element
try:
# Replace this with the id of the element
element = driver.find_element(By.CLASS_NAME, "entry-title")
except NoSuchElementException:
print("Not Found")
else:
print("Found")
Output:
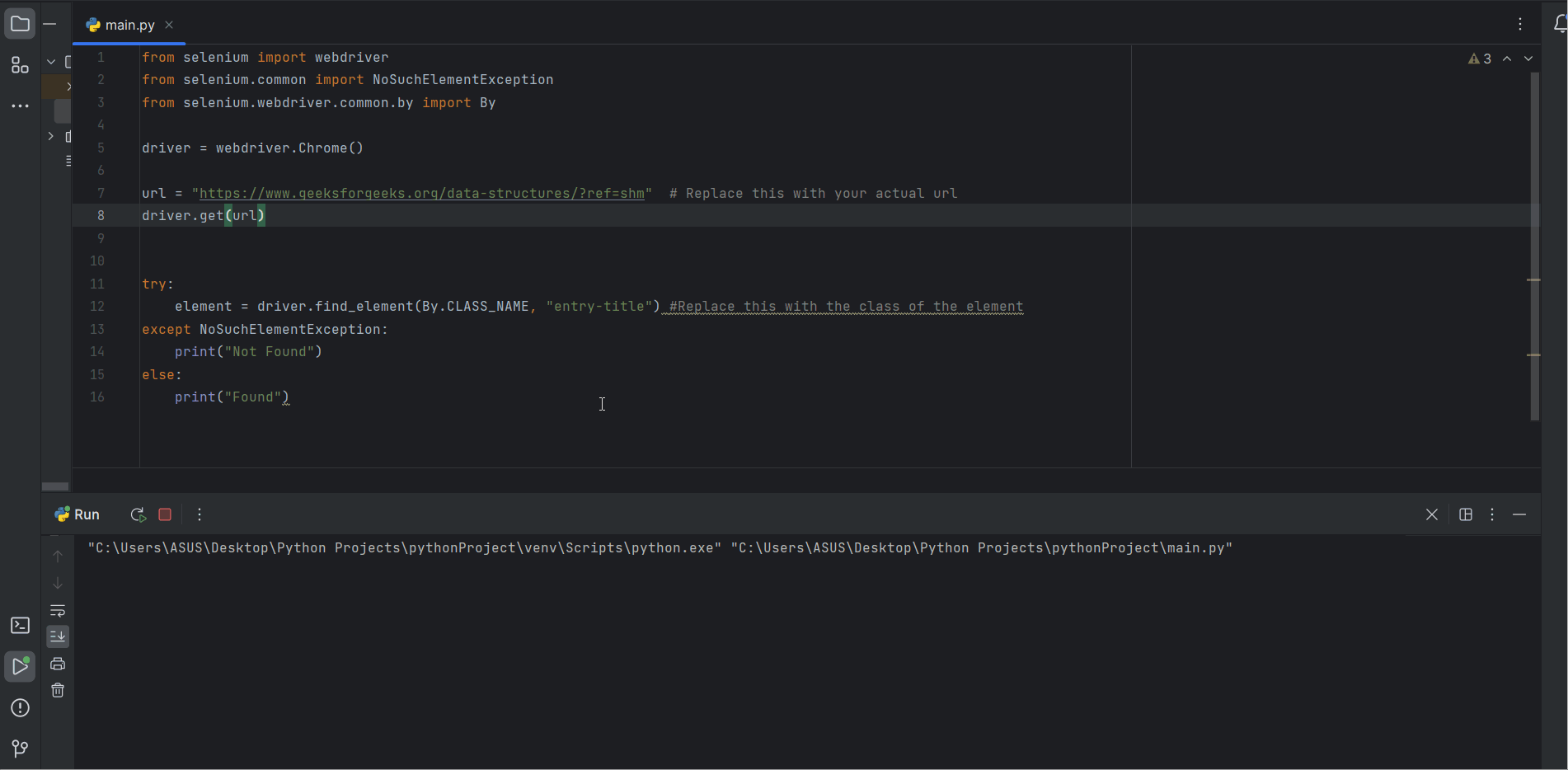
Selenium Locating Strategies
Explanation:
driver.find_element() return a element of type selenium.webDriver when it successfully find the elements otherwise it throws an NoSuchElementException exception.
3. Locating By Name
The name attribute of an HTML element is an identifier like a label . Unlike id ,class the name attribute doesn’t necessarily unique within a web page. They are often used for naming form elements and is used in HTML forms to handle form controls such as input fields , radio button etc. The name attribute is essential for from submission and used to associate input data to specific name. This makes name a useful locator strategy especially when dealing with form submissions.
- To locate an element by Name we use By.NAME locator provided by the By class.
- By is a class that provides a set of locating strategies to locate web elements on a web page.
Syntax:
element=driver.find_element(By.NAME, “element_name”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.NAME: This specifies that you’re locating the element by its name.
element_name: Replace this with the actual name of the element you want to locate.
Below is the Python program to implement Locating by name:
Python
# Python program to implement Locating by name
import time
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace this with your actual url
url = "https://www.geeksforgeeks.org/about/contact-us/"
driver.get(url)
# Replace ID_NAME with the Id of your element
try:
# Replace this with the name of the element
element = driver.find_element(By.NAME, "feedback_area")
except NoSuchElementException:
print("Not Found")
else:
print("Found")
Output:
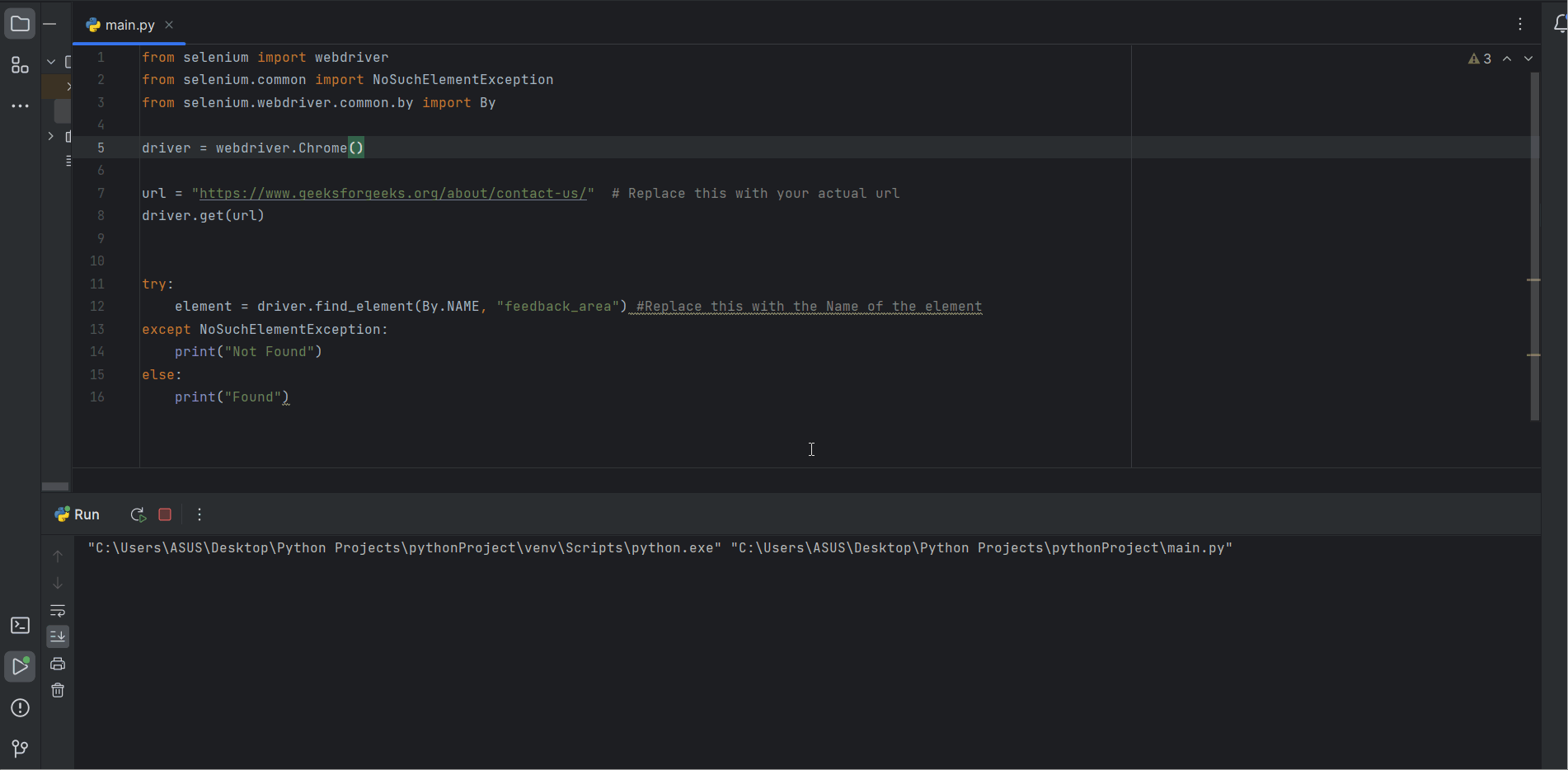
Selenium Locating Strategies
Explanation:
driver.find_element() return a element of type selenium.webDriver when it successfully find the elements otherwise it throws an NoSuchElementException exception.
4. Locating By Tag Name
Every element in HTML document is identified by a TAG NAME. Tag Name are used to define the purpose and type of an element in a webpage. Tag Name lets us locate an element on a web page using the Tag Name like button , useful when we are looking for a specific element in our web page. Common HTML tag names are “div”, “p”, “input”, “button” and many more.
- Locating an element with tag name is useful specially when you want to interact with a group of elements that share a common tag name.
- For example if we are testing a web application with multiple input fields , we can locate all the input fields by their “input” tag name ,this makes it easier to perform actions like filling out forms or validating inputs.
- To locate an element by TAG NAME we use By.TAG_NAME locator provided by the By class.
- By is a class that provides a set of locating strategies to locate web elements on a web page.
Syntax:
element=driver.find_element(By.TAG_NAME, “element_tag_name”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.TAG_NAME: This specifies that you’re locating the element by its tag name.
element_tag_name: Replace this with the actual tag_name of the element you want to locate.
Below is the Python program to implement Locating by tag name:
Python
# Python program to implement Locating by tag name
import time
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace this with your actual url
url = "https://www.geeksforgeeks.org/about/contact-us/"
driver.get(url)
try:
# Replace this with the Tag Name of the element
element = driver.find_element(By.TAG_NAME, "select")
except NoSuchElementException:
print("Not Found")
else:
print("Found")
Output:
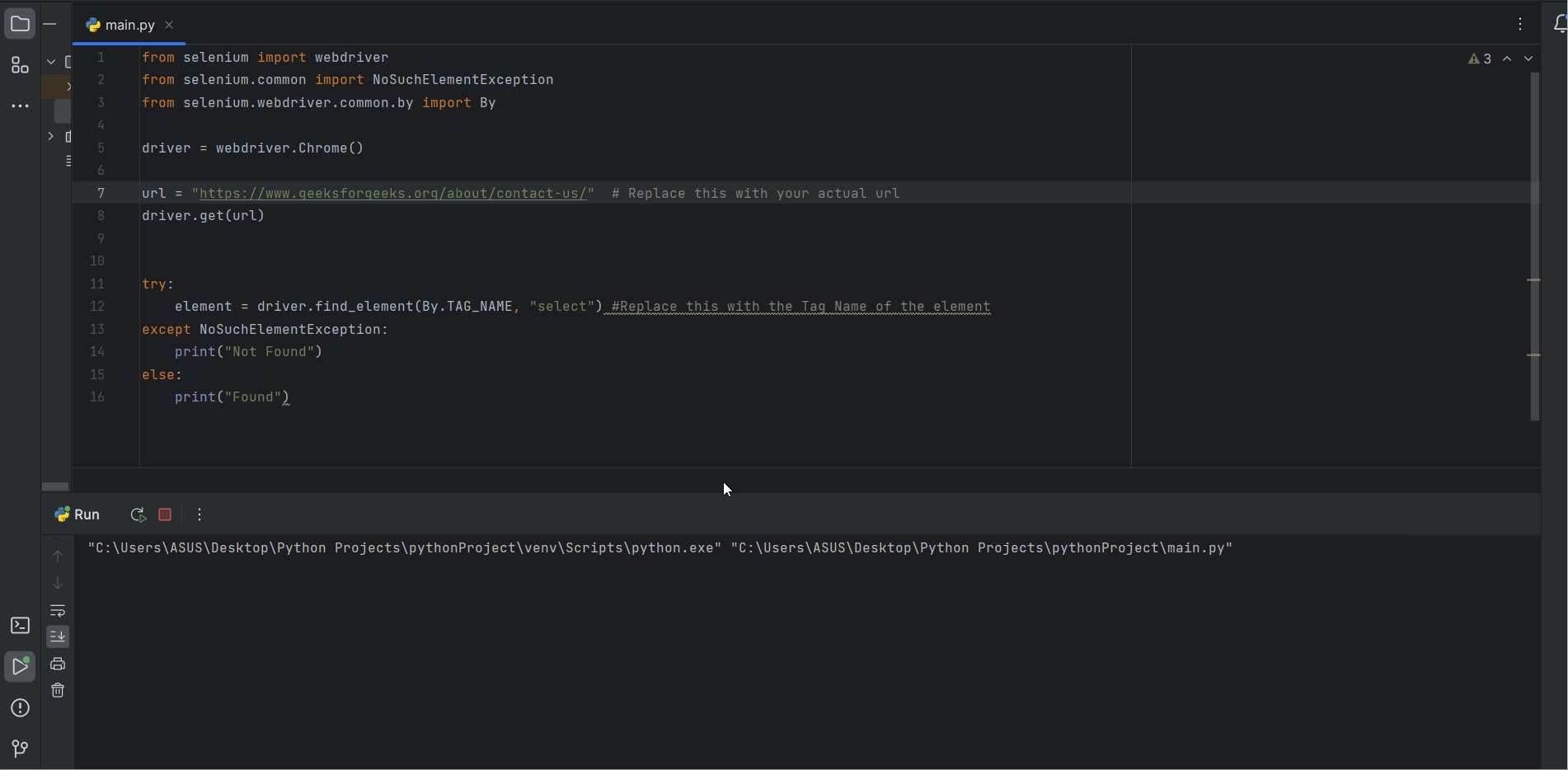
Selenium Locating Strategies
Explanation:
driver.find_element() return a element of type selenium.webDriver when it successfully find the elements otherwise it throws an NoSuchElementException exception.
5. Locating By CSS Selectors
CSS Selectors are the patterns that used to select and style HTML elements in web devleopment. CSS selectors allows us to target elements in a web page by their attributes ,position and hierarchy on a web page.
Some common CSS selectors are:
- Element Selectors: Selects an element based on their HTML tag name. For example ‘p’ selects all <p> elements.
- Class Selectors: Selects an element with a specific class attribute. For example ‘.btn’ selects all elemets with ‘class=”btn”‘.
- ID Selector: Selects an element with specific id attribute . For example “#header” will select all the element with ‘id=”header”‘.
- Descendent Selector: Selects an element that is a descendant of another element. For example, ‘ul li’ selects all ‘<li>’ element within a ‘<ul>’ element
To locate an element by CSS Selector we use By.CSS_SELECTOR locator provided by the By class. By is a class that provides a set of locating strategies to locate web elements on a web page.
Syntax:
driver.find_element(By.CSS_SELECTOR, “element_css_selector”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.CSS_SELECTOR: This specifies that you’re locating the element by its CSS_SELECTOR.
element_css_selector: Replace this with the actual css selector of the element you want to locate.
Below is the Python program to implement Locating by CSS Selector:
Python
# Python program to implement Locating by CSS Selector
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace this with your actual url
url = "https://www.geeksforgeeks.org/about/contact-us/"
driver.get(url)
try:
# Replace this with the css selector of the element
element = driver.find_element(By.CSS_SELECTOR, "div.title")
except NoSuchElementException:
print("Not Found")
else:
print("Found")
Output:
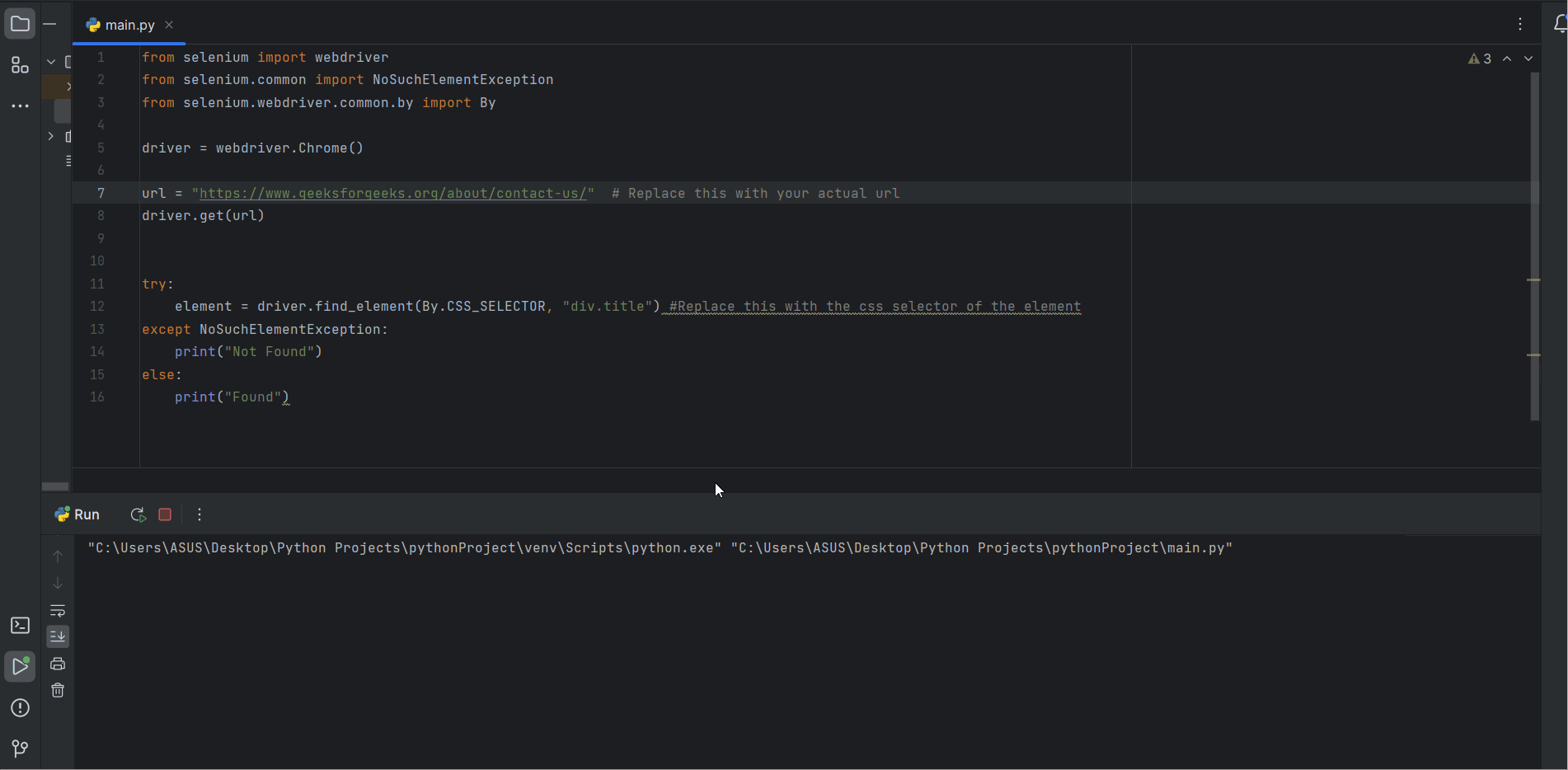
Selenium Locating Strategies
Explanation:
driver.find_element() return a element of type selenium.webDriver when it successfully find the elements otherwise it throws an NoSuchElementException exception.
6. Locating By XPath
XPath (XML Path Language) is a powerful and one of the best locating strategy in Selenium for identifying elements on a web page. XPath is a language used for navigating and querying XML documents but its widely used in web scrapping and automation.
- In selenium XPath is a valuable tool for selecting elements based on their attributes , position and relationship within the HTML structure of a web page.
- XPath is a powerful strategy but its slower than other locators. However its a good choice when other locating strategy don’t work or specially when the elements don’t have reliable ID’s, classes, or names.
- While using XPath in Selenium we’ll often inspect the webpage’s HTML source code to identify the attributes that uniquely identify the element we want to interact.
- These attributes can be id, class, or name.
- XPath provides a powerful way to combine these attributes to pinpoint elements accurately.
Syntax-
element=driver.find_element(By.XPATH, “element_xpath”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.XPATH: This specifies that you’re locating the element by its XPATH.
element_xpath: Replace this with the actual xpath of the element you want to locate.
Below is the Python program of Locating by XPath:
Python
# Python program of Locating by XPath
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace this with your actual url
url = "https://www.geeksforgeeks.org/about/contact-us/"
driver.get(url)
try:
# Replace this with the XPATH selector of the element
element = driver.find_element(By.XPATH,
"/html/body/div[3]/div[2]/div[1]/div/div")
except NoSuchElementException:
print("Not Found")
else:
print("Found")
Output:
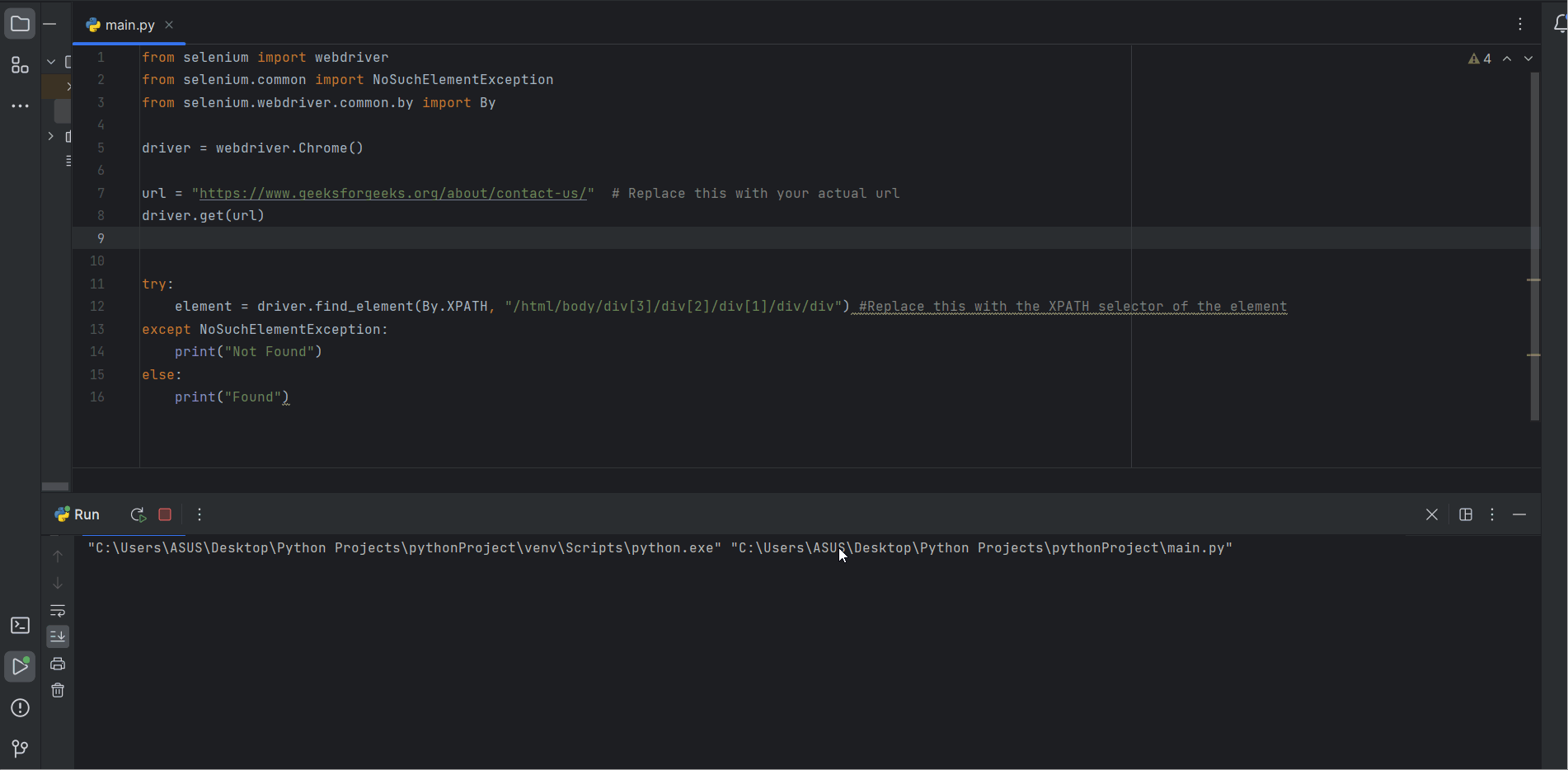
Selenium Locating Strategies
Explanation:
driver.find_element() return a element of type selenium.webDriver when it successfully find the elements otherwise it throws an NoSuchElementException exception.
7. Locating using Link Texts and Partial Link Texts
Link Texts as the name suggests are primarily used to locate anchor tags ‘<a>’ elements on a webpage . Anchor tags are primarily used for hyperlinks (those which navigate us to different page or resource on a website).
- To locate an element by its link text we provide the exact text that contained within the anchor elements. Selenium then searches the web page for an anchor element that matches with the text you provided.
- Partial link texts are similar to link texts but it allows us to search for elements by providing only a part of the link text instead of the full text.
- This can be particularly used when we want to locate element with dynamic or changing texts.
- By using By.LINK_TEXT we can find the elements with exact link text, while By.PARTIAL_LINK_TEXT we can find elements with partial match of link texts.
Syntax:
element = driver.find_element(By.LINK_TEXT, “element_link_text”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.LINK_TEXT: This specifies that you’re locating the element by its link_text.
element_link_text: Replace this with the actual link text of the element you want to locate.
Syntax:
element = driver.find_element(By.PARTIAL_LINK_TEXT, “element_partial_link_text”)
Here,
driver: This is the selenium web driver instance.
find_element(): This method is used to locate element in Selenium.
By.PARTIAL_LINK_TEXT: This specifies that you’re locating the element by its partial link text.
element_partial_link_text: Replace this with the actual partial link text of the element you want to locate.
Below is the Python program to implement Locating using Link Texts and Partial Link Texts:
Python
# Python program to implement Locating
# using Link Texts and Partial Link Texts
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace this with your url
url = "https://www.example.com"
driver.get(url)
# Replace Learn more with the Link Text of the element
element1 = driver.find_element(By.LINK_TEXT,
"Learn more");
# Replace Click with the Link Text of the element
element2 = driver.find_element(By.PARTIAL_LINK_TEXT,
"Click");
Output:
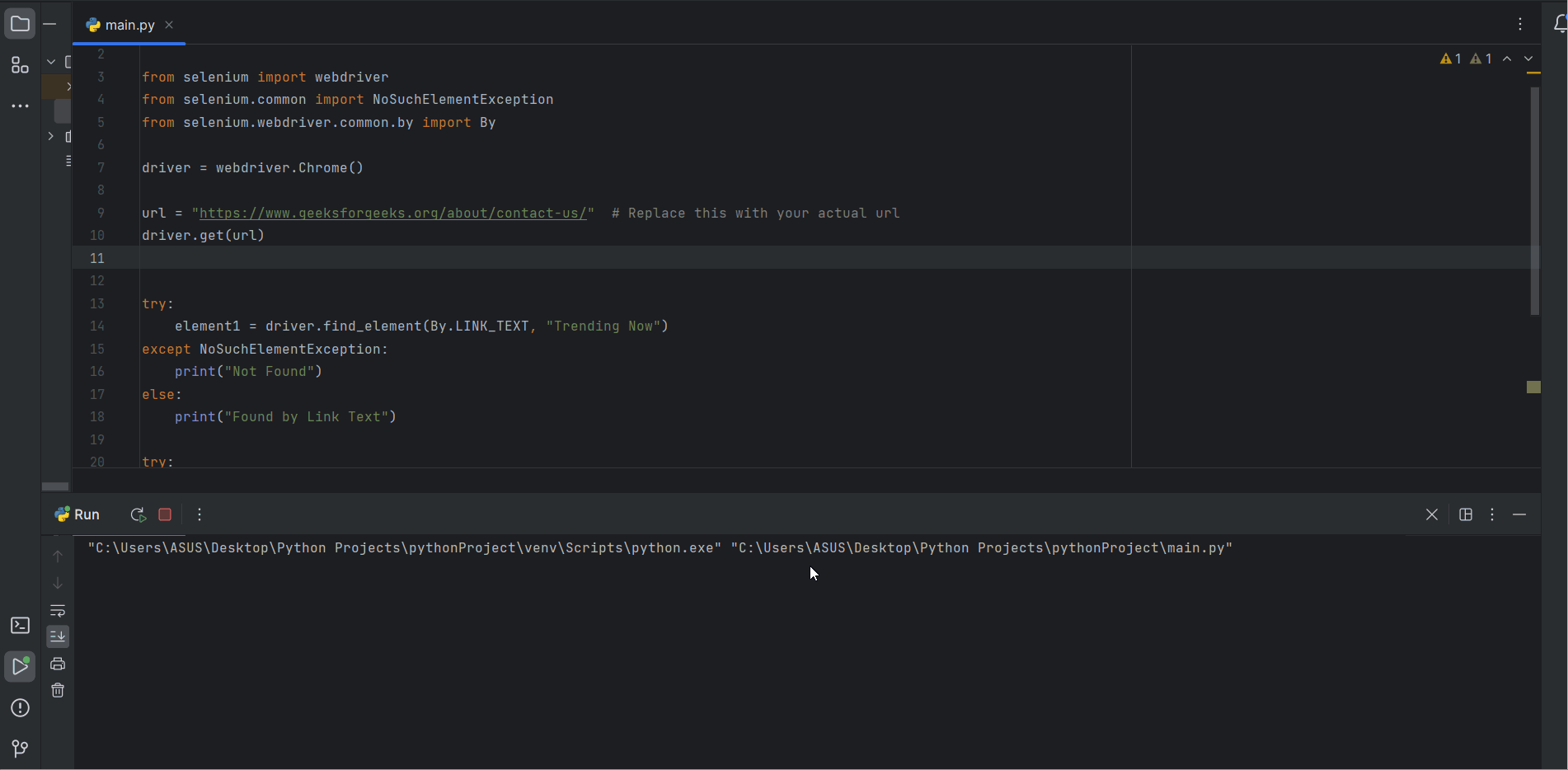
Selenium Locating Strategies
Explanation:
driver.find_element() return a element of type selenium.webDriver when it successfully find the elements otherwise it throws an NoSuchElementFound exception.
Share your thoughts in the comments
Please Login to comment...