JPA – Set Mapping
Last Updated :
08 Apr, 2024
In Java, JPA is defined as Java Persistence API. The mapping association between the entities is an important aspect of designing database-driven applications. One such mapping is the Set mapping. It establishes the one-to-many relationship between the entities.
Set mapping in JPA is used to represent the collection of related entities where each entity instance can occur at the most in the collection.
Steps to Implement Set Mapping in JPA
Here are the steps to implement the Set mapping.
Step 1: Define the entities
We can define the entities and it establish the one-to-many relationship using the @OneToMany annotation of the application.
@Entity
public class Parent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(mappedBy = "parent", cascade = CascadeType.ALL)
private Set<Child> children;
// Getters and setters
}
Step 2: We can use the @JoinColumn annotation to the specify the foreign key column in the child entity of the application.
@Entity
public class Child {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "parent_id")
private Parent parent;
// Getters and setters
}
Step 3: We can access and manipulate the associated entities through the Set collection in the parent entity of the application.
Project Implementation to demonstrate JPA Set Mapping
Below is the implementation with a suitable example to demonstrate Set Mapping in JPA.
Step 1: Create a new JPA project using Intellj Idea named jpa-set-mapping-demo.
Once the project creation done, the folder structure will look like below image:
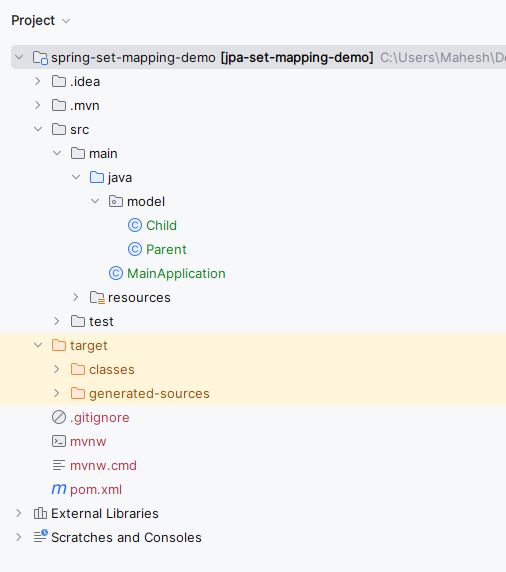
Step 2: Open the pom.xml and add the below dependencies into the project.
Dependency:
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
Step 3: Open the persistence.xml and paste the below code into the project to configure the project database.
XML
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<persistence xmlns="https://jakarta.ee/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/persistence https://jakarta.ee/xml/ns/persistence/persistence_3_0.xsd"
version="3.0">
<persistence-unit name="example-unit">
<class>model.Parent</class>
<class>model.Child</class>
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/example"/>
<property name="javax.persistence.jdbc.user" value="root"/>
<property name="javax.persistence.jdbc.password" value=""/>
<property name="javax.persistence.jdbc.driver" value="com.mysql.jdbc.Driver"/>
<property name="hibernate.dialect" value="org.hibernate.dialect.MySQL5Dialect"/>
<property name="hibernate.hbm2ddl.auto" value="update"/>
</properties>
</persistence-unit>
</persistence>
Step 4: Create a new Java package named model. Inside that package, create a new Entity Java class named Parent.
Go to src > main > java > model > Parent and put the below code.
Java
package model;
import jakarta.persistence.*;
import java.util.Set;
@Entity
public class Parent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(mappedBy = "parent", cascade = CascadeType.ALL)
private Set<Child> children;
// Getters and setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Set<Child> getChildren() {
return children;
}
public void setChildren(Set<Child> children) {
this.children = children;
}
}
Step 5: Create a new Entity Java class named Child.
Go to src > main > java > model > Child and put the below code.
Java
package model;
import jakarta.persistence.*;
@Entity
public class Child {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "parent_id")
private Parent parent;
// Getters and setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Parent getParent() {
return parent;
}
public void setParent(Parent parent) {
this.parent = parent;
}
}
Step 6: Create a new Java class named MainApplication.
Go to src > main > java > MainApplication and write the below provided code there.
Java
import jakarta.persistence.EntityManager;
import jakarta.persistence.EntityManagerFactory;
import jakarta.persistence.Persistence;
import model.Child;
import model.Parent;
import java.util.HashSet;
import java.util.Set;
public class MainApplication {
public static void main(String[] args) {
EntityManagerFactory entityManagerFactory = Persistence.createEntityManagerFactory("example-unit");
EntityManager entityManager = entityManagerFactory.createEntityManager();
// Create parent entity
Parent parent = new Parent();
Set<Child> children = new HashSet<>();
// Create child entities
Child child1 = new Child();
child1.setParent(parent);
Child child2 = new Child();
child2.setParent(parent);
// Add children to parent
children.add(child1);
children.add(child2);
parent.setChildren(children);
// Begin transaction
entityManager.getTransaction().begin();
// Persist parent and children
entityManager.persist(parent);
entityManager.persist(child1);
entityManager.persist(child2);
// Commit transaction
entityManager.getTransaction().commit();
// Display relationships
System.out.println("Parent ID: " + parent.getId());
for (Child child : parent.getChildren()) {
System.out.println("Child ID: " + child.getId() + ", Parent ID: " + child.getParent().getId());
}
// Close EntityManager and EntityManagerFactory
entityManager.close();
entityManagerFactory.close();
}
}
- This MainApplication demonstrates the management of parent-child relationships using JPA.
- It creates a Parent entity and two Child entities.
- It establishes the relationship between them, persists them into the database, and displays their IDs along with the parent-child associations.
- Finally, it closes the EntityManager and EntityManagerFactory to release resources.
pom.xml:
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>jpa-set-mapping-demo</artifactId>
<version>1.0-SNAPSHOT</version>
<name>spring-set-mapping-demo</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.target>11</maven.compiler.target>
<maven.compiler.source>11</maven.compiler.source>
<junit.version>5.9.2</junit.version>
</properties>
<dependencies>
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
</plugins>
</build>
</project>
Step 7: Once the project is completed, run the application. It will then show the Parent and Child along with their ID’s as an output. Refer the below console output image for better understanding.
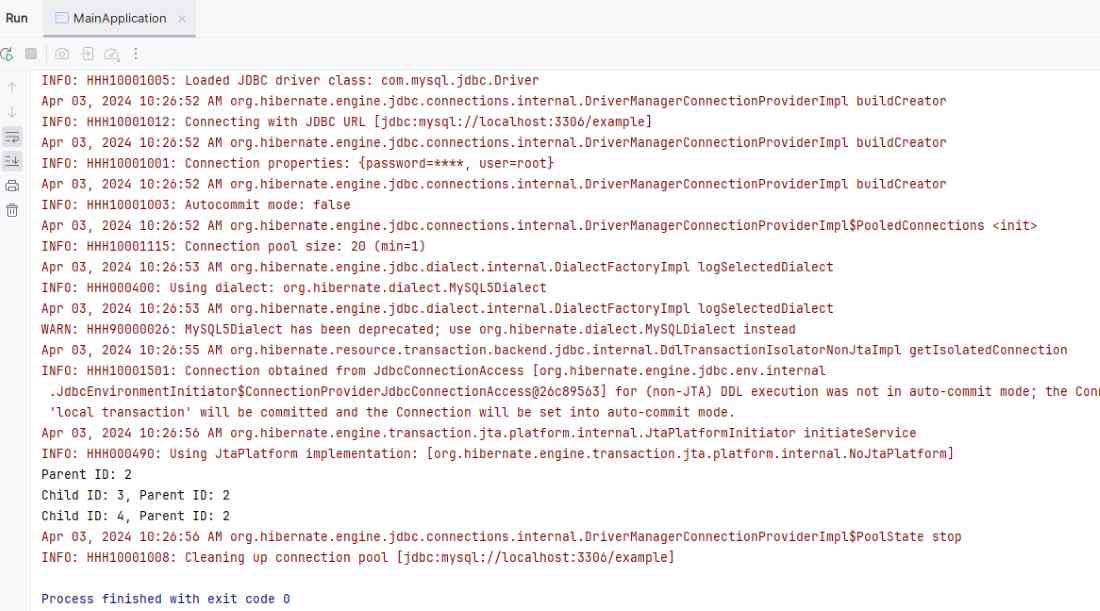
By the understanding the concept and implementation steps, developers can effectively the model complex data structures and relationships in their database driven applications.
Share your thoughts in the comments
Please Login to comment...