JPA – Criteria WHERE Clause
Last Updated :
05 Apr, 2024
JPA in Java is defined as the Java Persistence API and the Criteria API provides a structured and type-safe way to build dynamic queries at runtime. The WHERE clause is an integral part of any SQL query and allows us to modify records based on specified conditions. Similarly, the WHERE clause in the JPA Criteria API is used to specify filtering criteria for retrieving entities from the database.
Implementation Steps:
The basic syntax for using the WHERE clause in the JPA Criteria API involves the following steps:
- First, we obtain the CriteriaBuilder instance from the EntityManager.
- Then create the CriteriaQuery for the desired entity type.
- After that, we will define the root entity from the clause using the from() method.
- Then we will use the CriteriaBuilder to construct predicates to filter the conditions.
- Now we will add the predicates to the CriteriaQuery using the where() method.
- Finally, it will execute the query using the EntityManager to obtain the result set.
Key Terminologies:
- CriteriaBuilder: It is the instance that can be obtained from the EntityManager which is used to construct the various parts of the CriteriaQuery. It includes the predicates for the WHERE clause.
- CriteriaQuery: It can represent the query object that encapsulates the information needed to the expressions, ordering and filtering the criteria.
- Root Entity: The root entity can be used to represent the main entity from which the query starts, and it is specified using from() method of the CriteriaQuery.
- Predicate: Used to represent the condition of the filtering added to the WHERE clause of the query and these are generated using CriteriaBuilder provided methods such as equal(), geaterThan() and lessThan().
- Where Clause: The where() method of CriteriaQuery can be used to specify filtering criteria and can join multiple predicates together using conjunctions(AND) or disjunctions(OR).
Example:
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<Employee> cq = cb.createQuery(Employee.class);
Root<Employee> root = cq.from(Employee.class);
Predicate condition = cb.equal(root.get("department"), "IT");
cq.where(condition);
List<Employee> resultList = entityManager.createQuery(cq).getResultList();
Step-by-Step Implementation of JPA Criteria WHERE Clause
We can develop the simple JPA application that can demonstrate the WHERE clause of the application.
Step 1: Create the new JPA project using the Intelj Idea named jpa-where-clause-demo. After creating the project, the file structure looks like the below image.
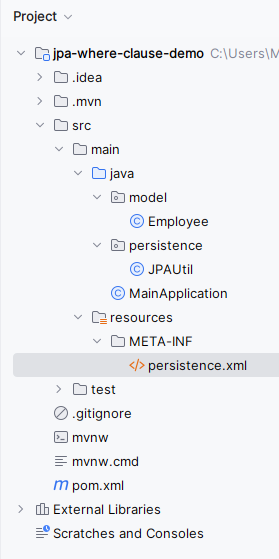
Step 2: Open the open.xml and add the below dependencies into the project.
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.27</version> <!-- Use the appropriate version -->
</dependency>
Step 3: Open the persistence.xml and put the below code into the project and it can configure the database of the project.
XML
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<persistence xmlns="https://jakarta.ee/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/persistence https://jakarta.ee/xml/ns/persistence/persistence_3_0.xsd"
version="3.0">
<persistence-unit name="persistenceUnitName">
<class>model.Employee</class>
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/example"/>
<property name="javax.persistence.jdbc.user" value="root"/>
<property name="javax.persistence.jdbc.password" value=""/>
<property name="javax.persistence.jdbc.driver" value="com.mysql.jdbc.Driver"/>
<property name="hibernate.dialect" value="org.hibernate.dialect.MySQL5Dialect"/>
<property name="hibernate.hbm2ddl.auto" value="update"/>
</properties>
</persistence-unit>
</persistence>
Step 4: Create the new Java package named as model. In that package, create the new Entity Java class named as Employee.
Go to src > main > java > model > Employee and put the below code.
Java
package model;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
/**
* Represents an Employee entity.
*/
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String department;
// Getters and Setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
}
Step 5: Create the new Java package named as persistence. In that package, create the new Entity java class named as JPAUtil.
Go to src > main > java > persistence > JPAUtil and put the below code.
Java
package persistence;
import jakarta.persistence.EntityManager;
import jakarta.persistence.EntityManagerFactory;
import jakarta.persistence.Persistence;
/**
* Utility class for managing the EntityManager and EntityManagerFactory.
*/
public class JPAUtil {
private static final String PERSISTENCE_UNIT_NAME = "persistenceUnitName";
private static EntityManagerFactory entityManagerFactory;
/**
* Initializes the EntityManagerFactory and retrieves the EntityManager instance.
*/
public static EntityManager getEntityManager() {
if (entityManagerFactory == null) {
entityManagerFactory = Persistence.createEntityManagerFactory(PERSISTENCE_UNIT_NAME);
}
return entityManagerFactory.createEntityManager();
}
/**
* Closes the EntityManagerFactory.
* This method should be called when the application shuts down.
*/
public static void closeEntityManagerFactory() {
if (entityManagerFactory != null && entityManagerFactory.isOpen()) {
entityManagerFactory.close();
}
}
}
Step 6: Create the new Java main class named as the MainApplication.
Go to src > main > java > MainApplication and put the below code.
Java
import jakarta.persistence.EntityManager;
import jakarta.persistence.criteria.CriteriaBuilder;
import jakarta.persistence.criteria.CriteriaQuery;
import jakarta.persistence.criteria.Predicate;
import jakarta.persistence.criteria.Root;
import model.Employee;
import persistence.JPAUtil;
import java.util.List;
public class MainApplication {
public static void main(String[] args) {
EntityManager entityManager = JPAUtil.getEntityManager();
// Add 5 entities
persistEmployees(entityManager);
// Criteria query to retrieve employees from the "IT" department
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<Employee> cq = cb.createQuery(Employee.class);
Root<Employee> root = cq.from(Employee.class);
Predicate condition = cb.equal(root.get("department"), "IT");
cq.where(condition);
List<Employee> resultList = entityManager.createQuery(cq).getResultList();
for (Employee employee : resultList) {
System.out.println(employee.getName() + " - " + employee.getDepartment());
}
entityManager.close();
JPAUtil.closeEntityManagerFactory();
}
private static void persistEmployees(EntityManager entityManager) {
entityManager.getTransaction().begin();
for (int i = 1; i <= 5; i++) {
Employee employee = new Employee();
employee.setName("Employee " + i);
employee.setDepartment(i % 2 == 0 ? "IT" : "HR"); // Alternating departments
entityManager.persist(employee);
}
entityManager.getTransaction().commit();
}
}
pom.xml:
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>jpa-where-clause-demo</artifactId>
<version>1.0-SNAPSHOT</version>
<name>jpa-where-clause-demo</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.target>11</maven.compiler.target>
<maven.compiler.source>11</maven.compiler.source>
<junit.version>5.9.2</junit.version>
</properties>
<dependencies>
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.27</version> <!-- Use the appropriate version -->
</dependency>
</dependencies>
<build>
<plugins>
</plugins>
</build>
</project>
Step 7: Once the project is completed, run the application. It will then show the Employee name and department name of the IT department in output. Refer the below output image for the better understanding of the concept.
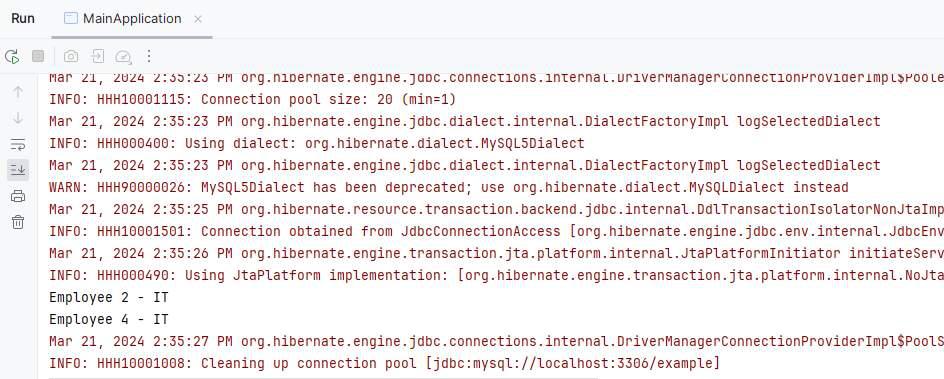
By the following the above steps of the article, developers can gain the solid understanding of the how to effectively use the WHERE clause in their JPA applications.
Share your thoughts in the comments
Please Login to comment...