JPA – CRUD
Last Updated :
02 Apr, 2024
JPA in Java can be called Java Persistence API (JPA) which can simplify the process of working with the database by providing object relationship mapping structure CRUD operations (Create, Read, Update, Delete) are actions especially if it contains database abuse.
JPA CRUD operation
JPA is a Java specification for Object-Relational Mapping (ORM). It provides a standard way to move Java objects to a related database table. CRUD operations are basic operations used to interact with data in a database. In JPA, these operations are performed on entities that are Java objects that represent data stored in the database.
Create Operation:
The Create operation involves inserting new records into the database. In JPA, this is achieved by the persisting new entity instances.
Steps to implement the create operation:
- Create a new instance of the entity class
- Set the values for its attributes.
- Use the EntityManagers persist() method to make entity managed and persistent.
Example:
EntityManager em = entityManagerFactory.createEntityManager();
EntityTransaction transaction = em.getTransaction();
transaction.begin();
//create the instance of the entity
Employee employee = new Employee();
//set the attributes
employee.setName("John");
employee.setSalary(50000);
em.persist(employee);
transaction.commit();
Read Operation:
The read operation involves the retrieving the existing records from the database. In JPA, this is the typically done using primary keys of the entity.
Steps to implement :
- Use the EntityManagers find() method to the retrieve the entity by its primary key.
Example:
Employee employee = em.find(Employee.class, 1L);
Update Operation:
The update operation involves modifying the existing records in the database. In JPA, this is the accomplished by the updating managed by the entity instances.
Steps to implement:
- Retrieve the entity using Entitymanagers find() method or the query.
- Modify the arrtibutes of the entity.
- Use the EntityManagers merge() method to the update the entity in database.
Example:
Employee employee = em.find(Employee.class, 1L);
employee.setSalary(55000);
em.merge(employee);
Delete Operation:
The delete operation involves removing records from the database. In JPA, this can be done by removing the managed entity instances.
Steps to implement:
- Retrieve the entity using the EntityManagers find() method or the query.
- Use the EntityManagers remove() method to the delete entity from the database.
Example:
Employee employee = em.find(Employee.class, 1L);
em.remove(employee);
In the JPA, these CRUD operations are typically performed within the transaction to the ensure data consistency and integrity. Transaction Management can be handled by the EntityManager which begins, commit or the rolls back transactions as necessary.
Step-by-step Implementation to perform CRUD Operation in JPA
We can develop a simple JPA application by performing the CRUD operation using MySQL database.
Step 1: First, we will create the JPA project using the Intellij Idea named as jpa-crud of the project.
Step 2: Now, we will add the below dependencies into the JPA project.
Dependencies:
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
Once the project creation completed, then the file structure will look like the below image.
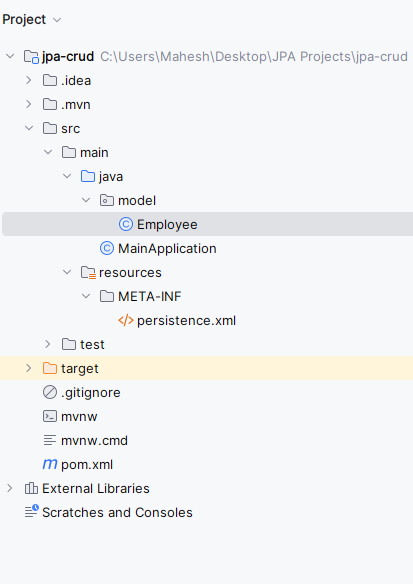
Step 3: Open persistance.xml and put the below code for the MYSQL database configuration of the database.
XML
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<persistence xmlns="https://jakarta.ee/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/persistence https://jakarta.ee/xml/ns/persistence/persistence_3_0.xsd"
version="3.0">
<persistence-unit name="persistenceUnitName">
<class>model.Employee</class>
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/example"/>
<property name="javax.persistence.jdbc.user" value="root"/>
<property name="javax.persistence.jdbc.password" value=""/>
<property name="javax.persistence.jdbc.driver" value="com.mysql.jdbc.Driver"/>
<property name="hibernate.dialect" value="org.hibernate.dialect.MySQL5Dialect"/>
<property name="hibernate.hbm2ddl.auto" value="update"/>
</properties>
</persistence-unit>
</persistence>
Step 4: Create the new Java package named as model in that package, create the new Entity Java class for the creation of the entity and it named as Employee.
Go to src > main > java > model > Employee and put the below code.
Java
package model;
import jakarta.persistence.*;
@Entity // Specifies that this class is an entity, mapped to a database table
@Table(name = "employees") // Specifies the table name in the database
public class Employee {
@Id // Specifies the primary key of the entity
@GeneratedValue(strategy = GenerationType.IDENTITY) // Specifies the generation strategy for the primary key
private Long id; // Primary key field
private String name; // Field to store employee name
private double salary; // Field to store employee salary
// Default constructor
public Employee() {
}
// Parameterized constructor
public Employee(Long id, String name, double salary) {
this.id = id;
this.name = name;
this.salary = salary;
}
// Getter and setter methods for id, name, and salary fields
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
}
- This class represents the entity Employee in the database.
- A JPA annotation is included to define its mapping to the corresponding database table.
- It contains fields for employee ID, name, and salary, and getter and setter methods for these fields.
Step 5: Create the new Java class and named as the MainApplication.
Go to src > main > java > MainApplication and put the below code.
Java
import jakarta.persistence.EntityManager;
import jakarta.persistence.EntityManagerFactory;
import jakarta.persistence.EntityTransaction;
import jakarta.persistence.Persistence;
import model.Employee;
import java.util.List;
public class MainApplication {
public static void main(String[] args) {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("persistenceUnitName");
EntityManager em = emf.createEntityManager();
EntityTransaction transaction = em.getTransaction();
try {
transaction.begin();
for (int i = 1; i <= 5; i++) {
Employee emp = new Employee();
emp.setName("Employee " + i); // Setting unique names for each employee
emp.setSalary(50000 + (i * 1000)); // Incrementing salary for each employee
em.persist(emp);
}
transaction.commit();
// Read Operation: Print all employees
transaction.begin(); // Begin a new transaction
List<Employee> allEmployees = em.createQuery("SELECT e FROM Employee e", Employee.class)
.getResultList();
System.out.println("All Employees:");
for (Employee employee : allEmployees) {
System.out.println(employee.getName() + " - " + employee.getSalary());
}
transaction.commit(); // Commit the transaction after reading
// Update Operation: Update two employees
transaction.begin(); // Begin a new transaction
Employee employeeToUpdate1 = em.find(Employee.class, 1L); // Assuming ID 1 and 2 for updating
employeeToUpdate1.setSalary(employeeToUpdate1.getSalary() + 5000); // Increasing salary
Employee employeeToUpdate2 = em.find(Employee.class, 2L);
employeeToUpdate2.setSalary(employeeToUpdate2.getSalary() + 5000); // Increasing salary
em.merge(employeeToUpdate1);
em.merge(employeeToUpdate2);
transaction.commit(); // Commit the transaction after updating
// Read Operation: Print updated employees
transaction.begin(); // Begin a new transaction
List<Employee> updatedEmployees = em.createQuery("SELECT e FROM Employee e WHERE e.id IN (1, 2)", Employee.class)
.getResultList();
System.out.println("\nUpdated Employees:");
for (Employee employee : updatedEmployees) {
System.out.println(employee.getName() + " - " + employee.getSalary());
}
transaction.commit(); // Commit the transaction after reading
// Delete Operation
transaction.begin(); // Begin a new transaction
for (Employee employee : updatedEmployees) {
em.remove(employee);
}
transaction.commit(); // Commit the transaction after deletion
} finally {
em.close();
emf.close();
}
}
}
The above class file describes a brief description of each step in the main application, including CRUD operations (Create, Read, Update, Delete) using JPA.
pom.xml:
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>jpa-crud</artifactId>
<version>1.0-SNAPSHOT</version>
<name>jpa-crud</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.target>11</maven.compiler.target>
<maven.compiler.source>11</maven.compiler.source>
<junit.version>5.9.2</junit.version>
</properties>
<dependencies>
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
</dependencies>
<build>
<plugins>
</plugins>
</build>
</project>
Step 6: Once the project is done, run the application and we can get the output like the below image.
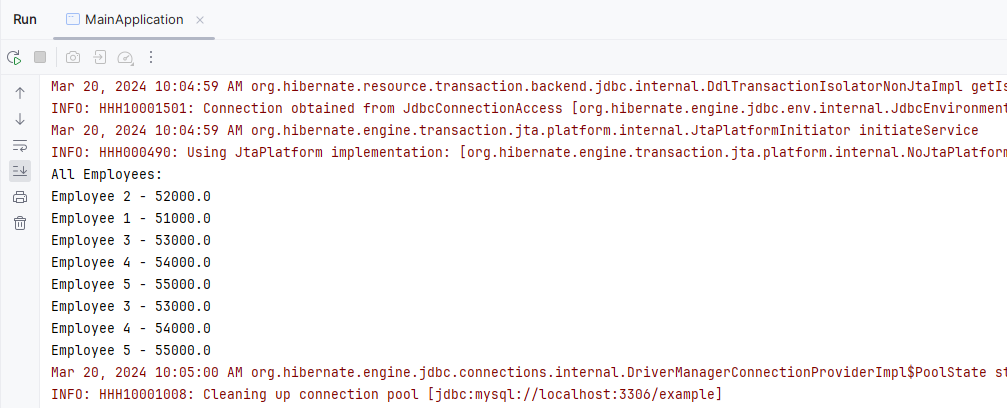
If we follow the above steps, then we can successfully demonstrate the CRUD application of the JPA.
JPA can simplifies the database operations in Java applications through its the ORM capabilities. Understanding the CRUD operations in the JPA is crucial for efficient data managements.
Share your thoughts in the comments
Please Login to comment...