JPA – Cascade Remove
Last Updated :
09 Apr, 2024
JPA in Java is referred to as Java Persistence API(JPA). Cascade Operations can provide a way to manage the persistence state of the related entities. One such cascade operation is “CascadeType.REMOVE” which can propagate the remove operation from the parent entity to its associated with the child entities of the JPA application.
Understanding of the Cascade Remove in JPA
Cascade Remove is the feature in JPA that can automate the removal of the associated child entities when the parent entity is deleted. It can simplify the management of the entity relationships by propagating the removal operation from the parent entity to its associated child entities of the JPA application.
Steps to Implement
1. Define the Entity classes
We can create Java classes that can represent the entities and it can annotate the fields and methods of the entity classes with the JPA annotations to specify the mapping to the database tables and establish the relationships.
Parent Entity:
@Entity
public class Parent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(mappedBy = "parent", cascade = CascadeType.REMOVE)
private List<Child> children;
// Other fields and methods
}
Child Entity
@Entity
public class Child {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
private Parent parent;
// Other fields and methods
}
2. Create the Parent and Child objects
We can create the instances of the Parent and Child entities and it can establish the parent child relationships of the entities.
Parent parent = new Parent();
Child child1 = new Child();
Child child2 = new Child();
child1.setParent(parent);
child2.setParent(parent);
parent.setChildren(Arrays.asList(child1, child2));
3. Remove the Parent Entity
When the parent entity is removed and the Cascade Remove can ensures that the associated with the child entities are also deleted automatically.
EntityManager entityManager = entityManagerFactory.createEntityManager();
entityManager.getTransaction().begin();
Parent managedParent = entityManager.find(Parent.class, parentId);
entityManager.remove(managedParent);
entityManager.getTransaction().commit();
entityManager.close();
Project Implementation of the Cascade Remove in JPA
Step 1: First, we will create a JPA project using Intellij Idea IDE. The project named as jpa-cascade-remove-demo.
Step 2: Now, we will add the following dependencies into the created JPA project.
Dependencies:
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
Now the project structure will look like below image:
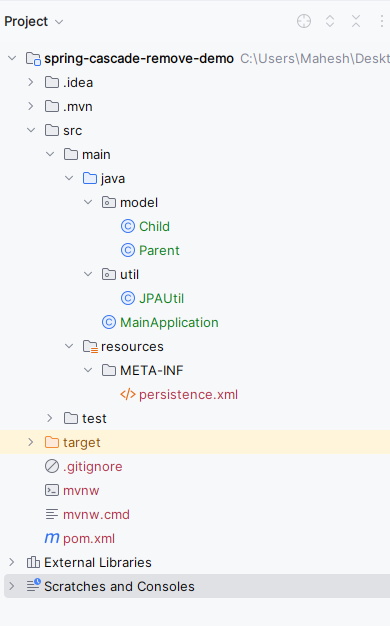
Step 3: Now, open the persistance.xml file and write the below code for MYSQL database configuration of the database.
XML
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<persistence xmlns="https://jakarta.ee/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/persistence https://jakarta.ee/xml/ns/persistence/persistence_3_0.xsd"
version="3.0">
<persistence-unit name="example-persistence-unit">
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/example"/>
<property name="javax.persistence.jdbc.user" value="root"/>
<property name="javax.persistence.jdbc.password" value=""/>
<property name="javax.persistence.jdbc.driver" value="com.mysql.jdbc.Driver"/>
<property name="hibernate.dialect" value="org.hibernate.dialect.MySQL5Dialect"/>
<property name="hibernate.hbm2ddl.auto" value="update"/>
</properties>
</persistence-unit>
</persistence>
Step 4: After all the above steps, now create a package named model and create an entity class inside that package named as Parent. Go to src > main > java > model > Parent and write the below code file..
Java
package model;
import jakarta.persistence.*;
import java.util.ArrayList;
import java.util.List;
@Entity
public class Parent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(mappedBy = "parent", cascade = CascadeType.ALL, orphanRemoval = true)
private List<Child> children = new ArrayList<>();
// Getters and setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public List<Child> getChildren() {
return children;
}
public void setChildren(List<Child> children) {
this.children = children;
}
}
In the Parent Entity class:
- @OneToMany annotation can defines the one-to-many relationship with the Child entity mappedBy = “parent” and it can indicates that the parent field in Child class maintains the relationship.
- cascade = CascadeType.ALL can specifies that all the persistence operations like persist, remove, merge, refresh should be the cascaded to associated with the child entities.
- orphanRemoval=True can ensures that if the child entity is removed from the parent collection and it will be the deleted from the database.
Step 5: After all the above steps, now create a package named model and create an entity class inside that package named as Child. Go to src > main > java > model > Child and write the below code file.
Java
package model;
import jakarta.persistence.*;
@Entity
public class Child {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
private Parent parent;
// Getters and setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Parent getParent() {
return parent;
}
public void setParent(Parent parent) {
this.parent = parent;
}
}
In the Child Entity class:
- @ManyToOne: This annotation can establishes the many-to-one relationship with Parent entity and it can indicates the each child belongs to the single parent.
Step 6: Create a new Java package named util in that package, create the new Entity Java class for the creation of the entity and it named as JPAUtil. Go to src > main > java > util > JPAUtil and put the below code.
Java
package util;
import jakarta.persistence.EntityManager;
import jakarta.persistence.EntityManagerFactory;
import jakarta.persistence.Persistence;
public class JPAUtil {
private static final EntityManagerFactory entityManagerFactory;
static {
entityManagerFactory = Persistence.createEntityManagerFactory("example-persistence-unit");
}
public static EntityManager getEntityManager() {
return entityManagerFactory.createEntityManager();
}
public static void close() {
entityManagerFactory.close();
}
}
- getEntityManager(): This method can returns the EntityManager instances that can be used to the interact with the persistence context of the application.
Step 7: Create a new Java class and named as the MainApplication. Go to src > main > java > MainApplication and put the below code.
Java
import jakarta.persistence.EntityManager;
import model.Child;
import model.Parent;
import util.JPAUtil;
import java.util.ArrayList;
import java.util.Arrays;
public class MainApplication {
public static void main(String[] args) {
EntityManager entityManager = JPAUtil.getEntityManager();
entityManager.getTransaction().begin();
try {
// Create Parent and Child entities
Parent parent = new Parent();
Child child1 = new Child();
Child child2 = new Child();
// Establish parent-child relationship
child1.setParent(parent);
child2.setParent(parent);
parent.setChildren(Arrays.asList(child1, child2));
// Persist parent along with children
entityManager.persist(parent);
entityManager.getTransaction().commit();
// Print IDs of parent and children
System.out.println("Parent ID: " + parent.getId());
for (Child child : parent.getChildren()) {
System.out.println("Child ID: " + child.getId());
}
// Remove parent entity (Cascade Remove will delete associated children)
entityManager.getTransaction().begin();
entityManager.remove(parent);
// Clear parent's reference to children
parent.setChildren(new ArrayList<>());
entityManager.getTransaction().commit();
// Print after removal (should show no children)
System.out.println("After removal:");
for (Child child : parent.getChildren()) {
System.out.println("Child ID: " + child.getId());
}
} catch (Exception e) {
e.printStackTrace();
entityManager.getTransaction().rollback();
} finally {
entityManager.close();
JPAUtil.close();
}
}
}
pom.xml:
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>spring-cascade-remove-demo</artifactId>
<version>1.0-SNAPSHOT</version>
<name>spring-cascade-remove-demo</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.target>11</maven.compiler.target>
<maven.compiler.source>11</maven.compiler.source>
<junit.version>5.9.2</junit.version>
</properties>
<dependencies>
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.0.2.Final</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>3.0.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
</plugins>
</build>
</project>
Step 8: Once the project is done, run the application and we will get the output like the below image.
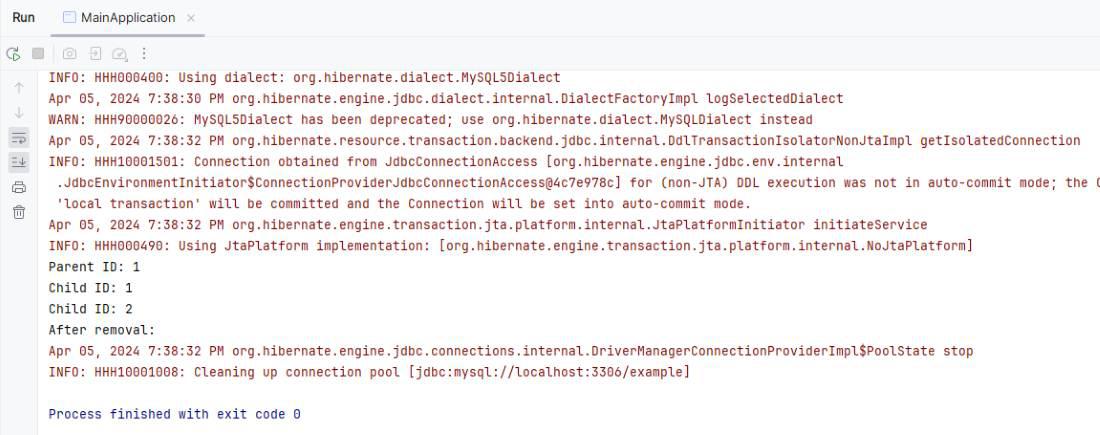
output
The above example can demonstrates the simple java application using JPA for the Cascade Remove and it can incudes the entity class for the Parent and Child along with the JPA utility class for managing the EntityManager instances.
Share your thoughts in the comments
Please Login to comment...