Java Program For Decimal to Octal Conversion
Last Updated :
08 Sep, 2022
Given a decimal number N, convert N into an equivalent octal number i.e convert the number with base value 10 to base value 8. The decimal number system uses 10 digits from 0-9 and the octal number system uses 8 digits from 0-7 to represent any numeric value.
Illustration:
Input : 33
Output: 41
Input : 10
Output: 12
Approach 1:
- Store the remainder when the number is divided by 8 in an array.
- Divide the number by 8 now
- Repeat the above two steps until the number is not equal to 0.
- Print the array in reverse order now.
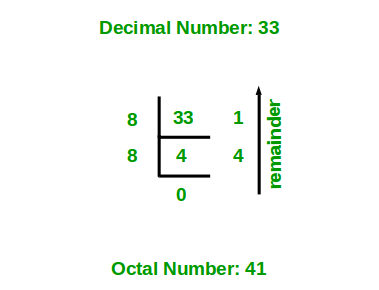
Example of converting the decimal number 33 to an equivalent octal number.
Example:
Java
import java.io.*;
class GFG {
static void decToOctal( int n)
{
int [] octalNum = new int [ 100 ];
int i = 0 ;
while (n != 0 ) {
octalNum[i] = n % 8 ;
n = n / 8 ;
i++;
}
for ( int j = i - 1 ; j >= 0 ; j--)
System.out.print(octalNum[j]);
}
public static void main(String[] args)
{
int n = 33 ;
decToOctal(n);
}
}
|
Time Complexity: O(log N)
Auxiliary Space: O(1) It is using constant space for variable and array OctalNum
Approach 2:
- Initialize ocatalNum with 0 and countVal with 1 and the decimal number as n
- Calculate the remainder when decimal number divided by 8
- Update octal number with octalNum + (remainder * countval)
- Increment the countval by countval*10
- Divide the decimal number by 8
- Repeat from step 2 until the decimal number is zero
Example:
Java
import java.io.*;
class GFG {
static void decimaltooctal( int deciNum)
{
int octalNum = 0 , countval = 1 ;
int dNo = deciNum;
while (deciNum != 0 ) {
int remainder = deciNum % 8 ;
octalNum += remainder * countval;
countval = countval * 10 ;
deciNum /= 8 ;
}
System.out.println(octalNum);
}
public static void main(String[] args)
{
int n = 33 ;
decimaltooctal(n);
}
}
|
Time Complexity: O(log N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...