Implementing Authentication Flows with React Hooks and Auth0
Last Updated :
20 Feb, 2024
Authentication is a crucial aspect of many web applications, ensuring that users have access to the appropriate resources while maintaining security. In this article, we’ll explore how to implement authentication flows in a React application using React Hooks and Auth0.
Prerequisites:
React Authentication Made Easy with Auth0 and Hooks:
Auth0 provides a simple way to add authentication to your React applications. With React Hooks, managing the authentication state becomes more straightforward and efficient. This guide will cover setting up Auth0, integrating it with a React application using Hooks, and handling authentication-related tasks.
Approach:
To implement authentication with React Hooks and Auth0, follow these steps:
- Create a React Application.
- Set up Auth0: Create an Auth0 account and set up an application. Note down the domain and client ID.
- Install Dependencies: Install the necessary packages using npm or yarn.
Steps to Create React Application And Installing Module:
Step 1:Â Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3:Â Install the necessary package in your application using the following command.
npm install @auth0/auth0-react
Step 4:Â After setting up react environment on your system, we can start by creating an App.js file and create a directory by the name of components in which we will write our desired function.
Project Structure:
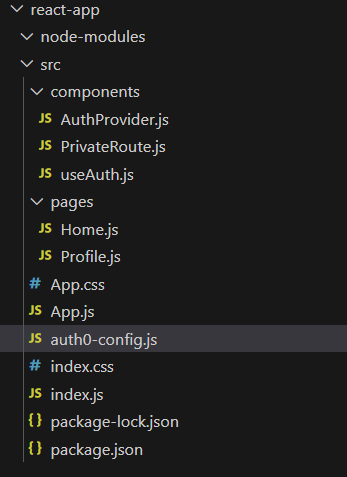
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@auth0/auth0-react": "^2.2.4",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: Write the following code in respective files to implement the approach
- Create Auth0Provider: Create a context provider to wrap your React application with Auth0 authentication.
- Use Auth0 Hooks: Utilize Auth0 hooks such as useAuth0 to access authentication state and functions in your components. for example Create a custom hook for handling authentication logic.
- Implement Authentication Flows: Implement login, logout, and authentication callback functions in your application.Use the custom hook in your components.
Javascript
import { Auth0Provider } from '@auth0/auth0-react' ;
import App from './App' ;
ReactDOM.render(
<Auth0Provider
domain= "your-auth0-domain"
clientId= "your-auth0-client-id"
redirectUri={window.location.origin}
>
<App />
</Auth0Provider>,
document.getElementById( 'root' )
);
|
Javascript
import { useAuth0 } from '@auth0/auth0-react' ;
export const useAuth = () => {
const { loginWithRedirect, logout, user, isAuthenticated } = useAuth0();
return {
login: () => loginWithRedirect(),
logout,
user,
isAuthenticated,
};
};
|
Javascript
import React from 'react' ;
import { useAuth } from './useAuth' ;
import './App.css' ;
const Profile = () => {
const { user, isAuthenticated, login, logout } = useAuth();
return (
<div className= "profile-container" >
{isAuthenticated ? (
<>
<p>Hello, {user.name}!</p>
<button className= "logout-button"
onClick={logout}>Logout</button>
</>
) : (
<button className= "login-button"
onClick={login}>Login</button>
)}
</div>
);
};
export default Profile;
|
Javascript
import React from 'react' ;
import Profile from './components/Profile' ;
const App = () => {
return (
<div >
<Profile />
</div>
)
}
export default App
|
CSS
.profile-container {
margin : 20px ;
display : flex;
flex- direction : column;
align-items: center ;
text-align : center ;
}
.login-button, .logout-button {
margin-top : 20px ;
padding : 8px 16px ;
font-size : 16px ;
border-radius: 4px ;
cursor : pointer ;
border : none ;
color : #fff ;
}
.login-button {
background-color : #6495ed ;
}
.logout-button {
background-color : #ff6347 ;
}
|
Start your application using the following command.
npm start
Output:
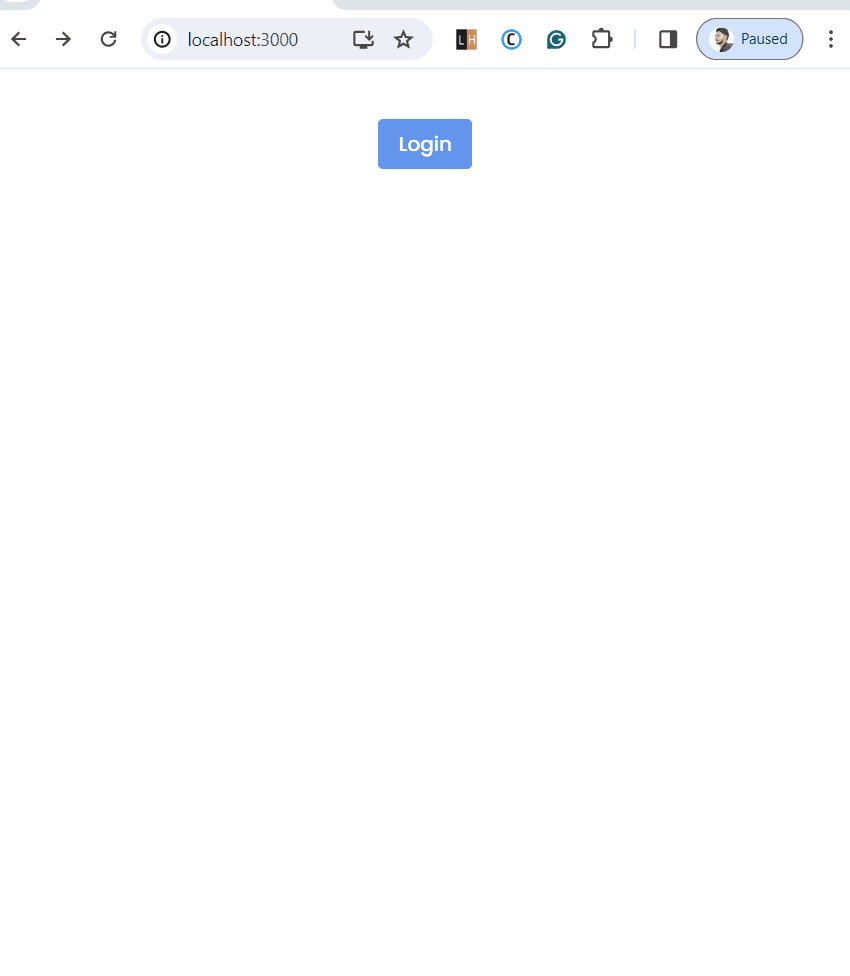
Output
Share your thoughts in the comments
Please Login to comment...