Authentication and Authorization with React Hooks
Last Updated :
04 Mar, 2024
Authentication and Authorization are fundamental aspects of web development, ensuring that users have secure access to resources within an application.
With the introduction of React Hooks, managing authentication and authorization in React applications has become more streamlined and efficient. In this article, we’ll explore how React Hooks can simplify the implementation of authentication and authorization mechanisms in your applications.
What is Authentication ?
The process of verifying the identity of a user, typically through credentials such as username and password. It commonly involves a username and password but can include additional factors like biometrics, multi-factor authentication, and token-based methods for enhanced security.
What is Authorization ?
Once authenticated, determine what actions and resources a user is allowed to access within the application. Authorization involves defining and enforcing permissions and privileges based on the user’s identity and role.
What are React Hooks ?
Introduced in React 16.8, Hooks are functions that enable developers to use state and other React features in functional components. They make handling state and other React things in your components a whole lot simpler, meaning your code looks good and is easier to understand.
Authentication with React Hooks:
Authentication in React applications typically involves managing user sessions, storing tokens, and handling login/logout functionality. React Hooks make it easier to manage authentication-related state and actions within functional components. Additionally, context and custom hooks enhances code reusability, providing a scalable and maintainable solution for authentication across various parts of a React application.
Example: Below is an example of Authentication with React Hooks.
Javascript
import React, { useState } from 'react' ;
const Login = () => {
const [isLoggedIn, setIsLoggedIn] = useState( false );
const handleLogin = () => {
setIsLoggedIn( true );
};
return (
<div>
{isLoggedIn ? (
<p>Welcome, User!</p>
) : (
<button onClick={handleLogin}>
Login
</button>
)}
</div>
);
};
export default Login;
|
Output:
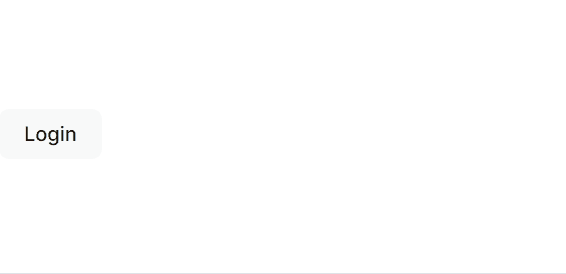
output
Authorization with React Hooks:
Authorization involves controlling access to different parts of the application based on user roles or permissions. React Hooks can be used to manage authorization logic within components. Additionally, combining hooks with context APIs facilitates centralized authorization management, streamlining the implementation of role-based access control across the application.
Example: Below is an example of authorization with React Hooks:
Javascript
import React, { useState } from 'react' ;
const Dashboard = () => {
const [isAdmin, setIsAdmin] = useState( true );
return (
<div>
{isAdmin ? (
<p>Welcome, Admin!</p>
) : (
<p>Unauthorized Access</p>
)}
</div>
);
};
export default Dashboard;
|
Output:
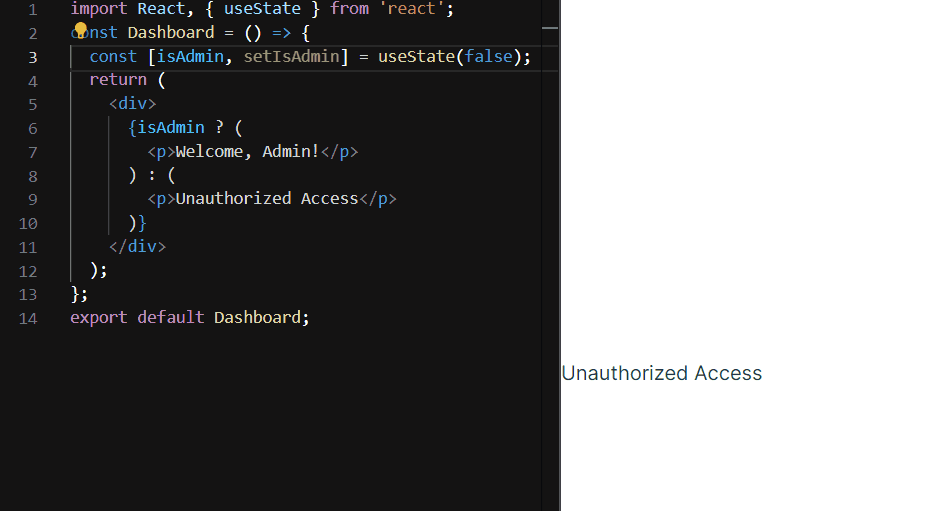
Output
Conclusion
React Hooks offer a powerful way to manage authentication and authorization logic within React applications. By using useState and other Hooks, users can easily handle authentication state, user sessions, and access control in functional components. This leads to cleaner, more maintainable code and a better developer experience overall. As you continue to explore React Hooks, leverage their capabilities to enhance the security and usability of your applications.
Share your thoughts in the comments
Please Login to comment...