How to use Native JavaScript Promises in Postman ?
Last Updated :
13 Mar, 2024
Postman is a popular tool used by developers for testing APIs. While Postman primarily offers a graphical user interface (GUI) for making HTTP requests, it also supports scripting capabilities using JavaScript. One common use case for scripting in Postman is making asynchronous requests and handling responses using promises.
We will discuss the different approaches to using native JavaScript promises in Postman.
Steps to Create an Application:
To use the approaches mentioned above in a Postman script, follow these steps:
- Open your Postman application.
- Create a new request or open an existing one.
- Go to the “Tests” tab.
- Write your script using one of the approaches mentioned above.
- Save your request.
Using pm.sendRequest and Promise constructor:
This approach involves creating a custom function to make asynchronous requests using the pm.sendRequest function within a Promise constructor. This method allows for greater control over error handling and response resolution.
Syntax:
function makeRequest(url) {
return new Promise((resolve, reject) => {
pm.sendRequest(url, (err, res) => {
if (err) {
reject(err);
} else {
resolve(res);
}
});
});
}
Example: This example implements the above mentioned approach.
Javascript
// Define a function to make a request using
// pm.sendRequest within a Promise
function makeRequest(url) {
return new Promise((resolve, reject) => {
pm.sendRequest(url, (err, res) => {
if (err) {
reject(err);
} else {
resolve(res);
}
});
});
}
// Example usage
makeRequest('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log('Response:', response.json());
})
.catch(error => {
console.error('Error:', error);
});
Output:
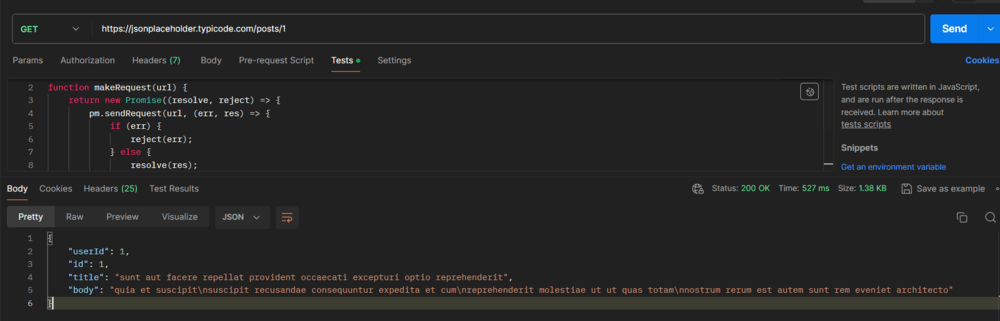
Using axios Library:
axios is a popular Promise-based HTTP client for making requests in both browsers and Node.js environments. This approach involves utilizing the axios library to simplify the process of making asynchronous requests with promises.
Syntax:
const axios = require('axios');
function makeRequest(url) {
return axios.get(url);
}
Example: This example implements the above mentioned approach
Javascript
// Import the axios library
const axios = require('axios');
// Define a function to make a request using axios
function makeRequest(url) {
return axios.get(url);
}
// Example usage
makeRequest('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log('Response:', response.data);
})
.catch(error => {
console.error('Error:', error);
});
Output:
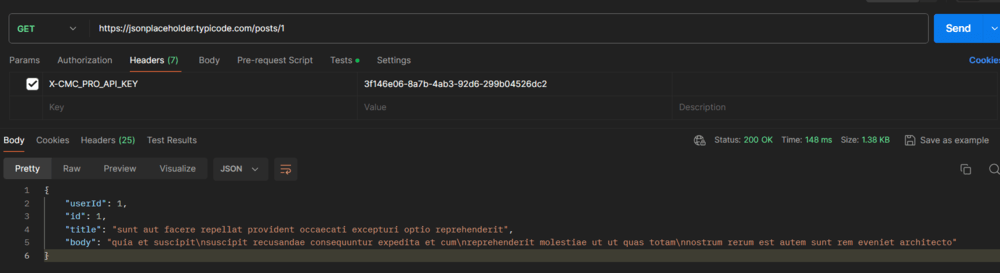
Using fetch API:
The fetch API provides a native browser API for making HTTP requests. This approach involves using the fetch API to make asynchronous requests and handle responses with promises.
Syntax:
function makeRequest(url) {
return fetch(url).then(response => response.json());
}
Example: This example implements the above mentioned approach
Javascript
// Define a function to make a request using the fetch API
function makeRequest(url) {
return fetch(url).then(response => response.json());
}
// Example usage
makeRequest('https://jsonplaceholder.typicode.com/posts/1')
.then(data => {
console.log('Data:', data);
})
.catch(error => {
console.error('Error:', error);
});
Output:
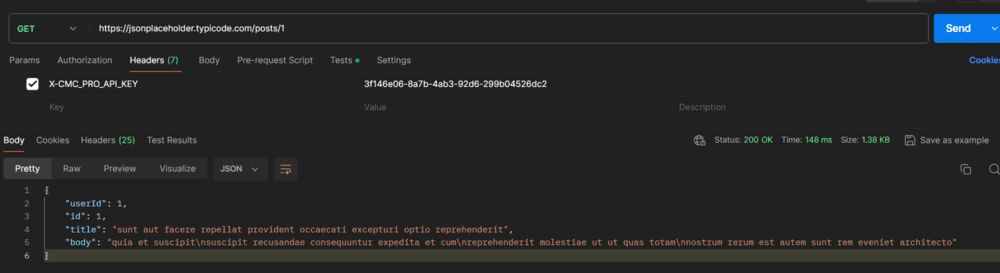
By following these steps and approaches, you can effectively use native JavaScript promises in Postman to make asynchronous requests and handle responses in your API testing workflows.
Share your thoughts in the comments
Please Login to comment...