JavaScript Program to Add Two Promises
Last Updated :
26 Mar, 2024
JavaScript Promises provide a powerful tool for asynchronous programming, allowing developers to work with values that may not be immediately available.
We’ll discuss multiple approaches to solve this problem, examining their syntax and providing examples for each approach.
Chaining
In this approach, we chain promises together using .then() to access the resolved values and perform the addition operation.
Syntax:
promise1.then((value1) => {
return promise2.then((value2) => {
return value1 + value2;
});
}).then((result) => {
// Handle the result
}).catch((error) => {
// Handle any errors
});
Example: In this example, adding the results of two promises in JavaScript using the chaining method with the help of .then().
JavaScript
const promise1 = new Promise((resolve) => {
setTimeout(() => {
resolve(5);
}, 1000);
});
const promise2 = new Promise((resolve) => {
setTimeout(() => {
resolve(10);
}, 2000);
});
promise1.then((value1) => {
return promise2.then((value2) => {
return value1 + value2;
});
}).then((result) => {
console.log(`The sum of two number is:${result.toString()}`);
}).catch((error) => {
console.error(error);
});
Output:
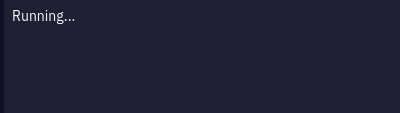
The sum of two numbers using Promises chaining in JavaScript.
Promise.all()
This approach involves using Promise.all() to wait for both promises to resolve and then perform the addition operation.
Syntax:
Promise.all([promise1, promise2]).then((values) => {
const result = values[0] + values[1];
// Handle the result
}).catch((error) => {
// Handle any errors
});
Example: In this example, adding the results of two promises in JavaScript using the Promise.all() method.
JavaScript
const promise1 = new Promise((resolve) => {
setTimeout(() => {
resolve(5);
}, 1000);
});
const promise2 = new Promise((resolve) => {
setTimeout(() => {
resolve(10);
}, 2000);
});
Promise.all([promise1, promise2]).then((values) => {
const result = values[0] + values[1];
console.log(`The sum of two number is:${result}`);
}).catch((error) => {
console.error(error);
});
Output:
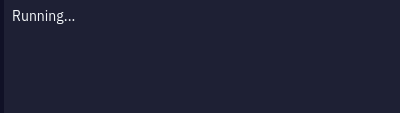
The sum of two numbers using Promise.All() method in JavaScript
Async/Await
This approach utilizes the async and await keywords to write asynchronous code in a synchronous style.
Syntax:
async function addPromises(promise1, promise2) {
try {
const value1 = await promise1;
const value2 = await promise2;
return value1 + value2;
} catch (error) {
// Handle any errors
}
}
// Usage
addPromises(promise1, promise2)
.then((result) => {
// Handle the result
})
.catch((error) => {
console.error(error);
});
Example: In this example, adding the results of two promises in JavaScript using the Async/Await method.
JavaScript
const promise1 = new Promise((resolve) => {
setTimeout(() => {
resolve(5);
}, 1000);
});
const promise2 = new Promise((resolve) => {
setTimeout(() => {
resolve(10);
}, 2000);
});
async function addPromises(promise1, promise2) {
try {
const value1 = await promise1;
const value2 = await promise2;
return value1 + value2;
} catch (error) {
console.error(error);
}
}
addPromises(promise1, promise2)
.then((result) => {
console.log(` The sum of two number is:${result}`);
})
.catch((error) => {
console.error(error);
});
Output:
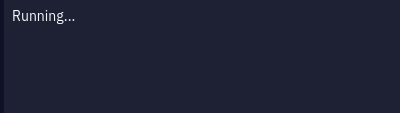
The sum of two number using Async and Await in JavaScript.
Share your thoughts in the comments
Please Login to comment...