How to handle Route Parameters in Express ?
Last Updated :
05 Jan, 2024
Express makes it easy to create strong and scalable web applications. One cool thing it can do is handle route parameters, which lets developers build dynamic routes that respond to specific values. In this article, we’ll see different ways to work with route parameters in Express.
Prerequisites:
Understanding Route Parameters:
In a web address, route parameters are like special slots that grab important values for the server. We mark these slots with a colon and give them a name in the route.
For example, consider the following route:
app.get('/users/:userId', (req, res) => {
const userId = req.params.userId;
// Rest of the logic to handle the user with the specified userId
});
In this route, `:userId` is a route parameter that can capture any value in the URL. Express automatically parses these parameters and makes them available in the `req.params` object.
Approach 1: Basic Route Parameter Handling
The simplest way to handle route parameters is by accessing them directly from `req.params`. You can extract the value of the parameter and use it in your route handler logic.
app.get('/users/:userId', (req, res) => {
const userId = req.params.userId;
// Rest of the logic to handle the user with the specified userId
});
Approach 2: Optional Route Parameters
Express allows you to define optional parameters in your routes by adding a question mark `?` to the parameter name. This means that the parameter is not required for the route to match.
app.get('/products/:productId?', (req, res) => {
const productId = req.params.productId || 'default';
// Logic to handle the product with the specified productId or a default value
});
Approach 3: Multiple Route Parameters
You can define routes with multiple parameters by adding additional colon-prefixed parameters in the route definition.
app.get('/posts/:category/:postId', (req, res) => {
const category = req.params.category;
const postId = req.params.postId;
// Logic to handle the post with the specified category and postId
});
Steps to setup Express server:
Step 1: Create a new directory for your project
mkdir express-routing-example
cd express-routing-example
Step 2: Initialize a new Node.js project
npm init -y
Step 3: Install the required dependencies.
npm install express
Folder Structure:
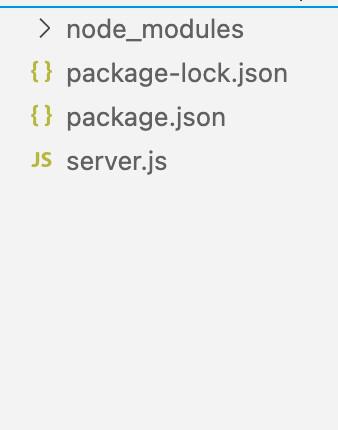
Folder structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Example: Create a file named server.js in your project directory and write the provided code into it.
Javascript
const express = require( "express" );
const app = express();
const PORT = 3000;
app.get( "/users/:userId" , (req, res) => {
const userId = req.params.userId;
res.send(`Result of BASIC ROUTE PARAMETER HANDLING: User ID: ${userId}`);
});
app.get( "/products/:productId?" , (req, res) => {
const productId = req.params.productId || "default" ;
res.send(
`Result of OPTIONAL ROUTE PARAMETER HANDLING: Product ID: ${productId}`
);
});
app.get( "/posts/:category/:postId" , (req, res) => {
const category = req.params.category;
const postId = req.params.postId;
res.send(
`Result of MULTIPLE ROUTE PARAMETER HANDLING:
Category: ${category}, Post ID: ${postId}`
);
});
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
To run the server: Execute the following command to start the server:
node server.js
This will start the server on http://localhost:3000 (or the port you specified in PORT).
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...