How to wait for multiple Promises in JavaScript ?
Last Updated :
09 Nov, 2023
In this article, we will discuss how to wait for multiple promises in JavaScript.
A Promise is basically an object that represents the completion (or failure) of an asynchronous operation along with its result. A promise has 3 types of states and based upon these states, the promise executes the results.
- Pending: This state represents that the promise is still in progress and hasn’t been fulfilled or rejected.
- Fulfilled: This state represents that the asynchronous operation is successfully completed.
- Rejected: This state represents that the asynchronous operation is rejected.
Promise. all( )
Promise. all() in JavaScript handles multiple promises concurrently, resolving when all promises in an array resolve, or rejecting if any of them fail, streamlining asynchronous operations.
Syntax
Promise.all([
// Array of promises
promise1,
promise2,
])
.then((results) => {
// Handle the resolved results
})
.catch((error) => {
// Handle errors on promise rejection.
});
Approach
- Create an array of promises that you want to wait for, e.g. promise1, promise2, and so on.
- Use Promise.all with the array of promises as its argument to wait for all of them to resolve. This method returns a new promise.
- In the .then callback, you can access the resolved values from all the promises as an array called results and handle them as needed.
- In the .catch callback, you can handle errors if at least one of the promises in the array is rejected.
Example: This example illustrates how to wait for multiple promises by using Promise.all( ) function in JavaScript.
Javascript
const promises = [
new Promise((resolve) =>
setTimeout(() => resolve( "first" ), 2000)),
new Promise((resolve) =>
setTimeout(() => resolve( "second" ), 2500)),
new Promise((resolve) =>
setTimeout(() => resolve( "third" ), 1000)),
];
Promise.all(promises)
.then((result) => {
console.log( "All promises have been resolved " , result);
})
. catch ((error) => {
console.error(
"At least any one promise is rejected:" , error);
});
|
Output:
All promises have been resolved [ 'first', 'second', 'third' ]
Async/await
Async/await is a JavaScript feature used to simplify asynchronous programming by allowing code to pause and resume using the await keyword within functions marked as async, enhancing readability and maintainability.
Syntax
async function fetchData() {
const [res1, res2] = await Promise.all([
fetch(' '),
fetch(' '),
]);
// Perform operations on result received
}
fetchData();
Approach
- Start by defining an async function, and create a function to handle your code after all promises are done.
- Inside the async function, create an array of promises, and collect promises for each asynchronous operation you need to await.
- Utilize the Promise.all() method to await all promises.
- After all promises have been resolved, we’ll receive an array of results: Once everything is done, you’ll get an array of results.
Example: This is the code showing how to wait for multiple promises by using async await in JavaScript.
Javascript
async function fetchData() {
const [title1, title2] =
await Promise.all([
.json(),
.json(),
]);
alert(`First title is
${title1.title} and Second title is
${title2.title}`);
}
fetchData();
|
Output:
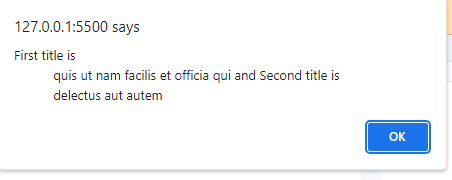
Output
Note: For this example, you may use any random Public API for the desired output.
Share your thoughts in the comments
Please Login to comment...