How to Use MaxLengthValidator in Django
Last Updated :
11 Oct, 2023
Django, an excessive degree Python web framework, affords a plethora of gear and capabilities to make net development simpler and extra green. One such feature is the MaxLengthValidator, a validation tool that lets you enforce man or woman limits on entering fields for your Django fashions. In this newsletter, we will discover a way to use MaxLengthValidator in Django to make sure that your information stays inside detailed duration constraints.
MaxLengthValidator in Django
Install Django and follow these steps:
Creating the Project Files
To start the project use this command
django-admin startproject microblog
cd microblog
To start the app use this command
python manage.py startapp app
Now add this app to the ‘settings.py’ in the installed app.
Setting up the Files
app/model.py: In this model, we have a content field with a maximum length of 14 characters, and we’ve added the ‘MaxLengthValidator’ to enforce this limit.
Python3
from django.db import models
from django.core.validators import MaxLengthValidator
class Message(models.Model):
content = models.CharField(
max_length = 140 ,
validators = [MaxLengthValidator(limit_value = 14 ,
message = "Message is too long" )]
)
created_at = models.DateTimeField(auto_now_add = True )
def __str__( self ):
return self .content
|
app/form.py: It is used to create a form for adding new messages in Django forms
Python3
from django import forms
from .models import Message
class MessageForm(forms.ModelForm):
class Meta:
model = Message
fields = [ 'content' ]
|
app/views.py: we define two views:
- home: Displays existing messages in descending order of creation.
- add_message: Handles adding new messages and enforces the character limit.
Python3
from django.shortcuts import render, redirect
from .models import Message
from .forms import MessageForm
def index(request):
messages = Message.objects. all ().order_by( '-created_at' )
return render(request, 'myapp/index.html' , { 'messages' : messages})
def add_message(request):
if request.method = = 'POST' :
form = MessageForm(request.POST)
if form.is_valid():
form.save()
return redirect( 'home' )
else :
form = MessageForm()
return render(request, 'myapp/index2.html' , { 'form' : form})
|
Creating GUI for the Project
Create a templates folder and, create 2 files, Here we named it as index.html and index2.html.
index.html: We create HTML templates for displaying messages (index.html) and adding messages (index2.html) in the templates folder.
HTML
<-- index.html -->
<!DOCTYPE html>
< html >
< head >
< title >Microblog</ title >
</ head >
< body >
< h1 >Microblog</ h1 >
< ul >
{% for message in messages %}
< li >{{ message.content }}</ li >
{% endfor %}
</ ul >
< a href = "{% url 'add_message' %}" >Add Message</ a >
</ body >
</ html >
|
index2.html: This HTML page is used to collect the Information from the user.
HTML
<-- index2.html -->
<!DOCTYPE html>
< html >
< head >
< title >Add Message</ title >
</ head >
< body >
< h1 >Add Message</ h1 >
< p >The word limit is 13</ p >
< form method = "post" >
{% csrf_token %}
{{ form.as_p }}
< input type = "submit" value = "Submit" >
</ form >
< a href = "{% url 'home' %}" >Back to Home</ a >
</ body >
</ html >
|
app/urls.py: URLs for the app are defined in app/urls.py, including the homepage and the add message page.
Python3
from django.urls import path
from . import views
urlpatterns = [
path(' ', views.index, name=' home'),
path( 'add/' , views.add_message, name = 'add_message' ),
]
|
urls.py: We include the app’s URLs in the project’s urls.py.
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' app.urls')),
]
|
Deployement of the Project
Migrate your files to the database:
python manage.py makemigrations
python manage.py migrate
Deploy your project
python manage.py runserver
Output:
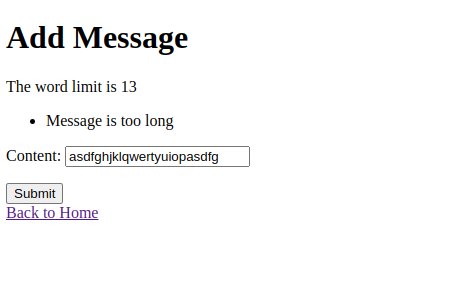
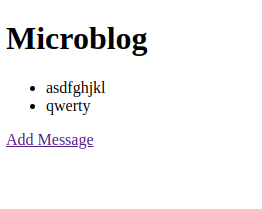
Share your thoughts in the comments
Please Login to comment...