How to use ‘validate_email’ in Django?
Last Updated :
09 Oct, 2023
One crucial aspect of web development is managing user data, and email validation is a common task in this regard. The validate_email function in Django is a handy tool that helps developers ensure the validity of email addresses submitted by users. In this article, we will explore how to use validate_email in Django.
How to use ‘validate_email’ in Django?
validate_email is a function provided by Django’s validators module that allows you to check if a given string is a valid email address. It’s part of Django’s built-in form and model field validation system, making it easy to incorporate email validation into your application.
Setting Up the Project
Use the below command to start your project:
django-admin startproject <project_name>
cd <project_name>
python manage.py startapp user
user/model.py: Here we have created a User table with name, and email field in the table.
Python3
from django.db import models
from django.core.validators import validate_email
class User(models.Model):
name = models.CharField(max_length = 100 )
email = models.EmailField(validators = [validate_email])
def __str__( self ):
return self .name
|
user/form.py: Here we created a form to register a user request.
Python3
from django import forms
from .models import User
class UserForm(forms.ModelForm):
class Meta:
model = User
fields = [ 'name' , 'email' ]
|
user/views.py: This file uses the form and renders a template to collect user data an dverify ‘.
Python3
from django.shortcuts import render
from .forms import UserForm
def create_user(request):
if request.method = = 'POST' :
form = UserForm(request.POST)
if form.is_valid():
form.save()
else :
form = UserForm()
return render(request, 'index.html' , { 'form' : form})
def home(request):
return HttpResponse( 'Hello, World!' )
|
templates/index.html: A HTML form for a user to validate it’s user id email.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >User Registration</ title >
</ head >
< body >
< h1 >User Registration</ h1 >
< form method = "post" >
{% csrf_token %}
{{ form.as_p }}
< input type = "submit" value = "Register" >
</ form >
</ body >
</ html >
|
user/urls.py: Define the URL patterns in the urls.py file of the user app to map views to URLs.
Python3
from django.urls import path
from . import views
urlpatterns = [
path( '/home' , views.home, name = 'home' ),
path(' ', views.create_user, name=' create_user'),
]
|
urls.py: Add the necessary URL patterns in your project’s urls.py.
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' user.urls')),
]
|
Deploy the Project
Migrate the data into the database.
python manage.py makemigrations
python manage.py migrate
Deploy the project
python manage.py runserver
Output
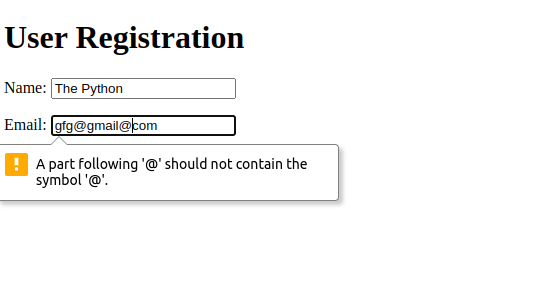
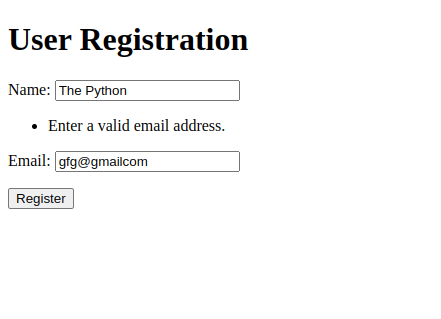
Share your thoughts in the comments
Please Login to comment...