How to Strike Through Text When Checking a Checkbox in ReactJS ?
Last Updated :
26 Oct, 2023
In this article, we are going to learn about strike-through text when checking a checkbox. Strike through text when checking a checkbox in React-Bootstrap refers to the functionality of applying a strikethrough style to text when a corresponding checkbox is checked. It is commonly used to visually indicate completed or selected items in lists or tasks within a React-Bootstrap-based user interface.
We will explore all the above methods along with their basic implementation with the help of examples.
Steps to Create React Application And Installing Module:
Creating React Application And Installing Module:
Step 1: Create a React application using the following command
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command.
cd foldername
Step 3: After creating the ReactJS application, Install the required modules using the following command.
npm install --save mdbreact react-chartjs-2
Step 4: Add Bootstrap CSS and fontawesome CSS to index.js.
import '@fortawesome/fontawesome-free/css/all.min.css';
import 'bootstrap-css-only/css/bootstrap.min.css';
import 'mdbreact/dist/css/mdb.css';
Project structure:
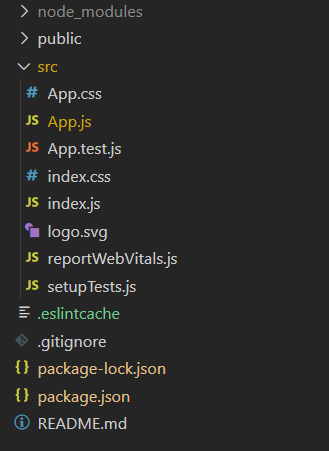
Approach 1 : Using React State and CSS
In this approach,We’ll manage the strike-through state using React’s state management, apply conditional rendering based on the checkbox state, and use CSS text decoration style to toggle the strike-through effect based on the checkbox’s state.
Example 1: In this example, we use React Bootstrap components. It features a gray background, a green <h2> heading, and a checkbox. When the checkbox is clicked, the text below toggles between having a strikethrough effect and normal style.
Javascript
import { useState } from "react" ;
import Form from "react-bootstrap/Form" ;
function App() {
const [strikeThroughCSS, setStrikeThroughCSS] =
useState( false );
const pageStyle = {
backgroundColor: "gray" ,
padding: "40px" ,
};
return (
<div style={pageStyle}>
<Form>
<h2 style={{ color: "green" }}>
GeeksforGeeks
</h2>
<Form.Check
type={ "checkbox" }
onClick={() =>
setStrikeThroughCSS((prev) => !prev)}
/>
<p style={
{
textDecoration: strikeThroughCSS ?
"line-through" : "none"
}}>
Using CSS text decoration style
Strikethrough text effect
</p>
</Form>
</div>
);
}
export default App;
|
Steps to Run the Application:
To run the application, type the following command:
npm run dev
Open your browser and go to http://localhost:5173/
Output:
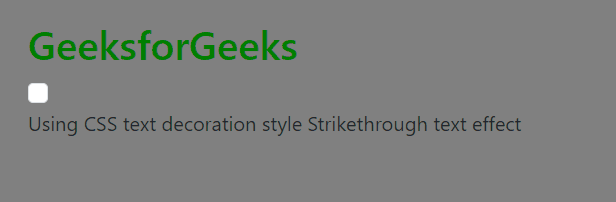
Approach 2 Using <del> HTML tag and map():
In this approach, we use the <del> tag and the map function to create a checkbox-controlled strikethrough effect for a list of text items. Checked checkboxes apply the <del> tag, striking through the text.
Example 1: In this example, we use the <del> tag and the map function to create a checkbox-controlled strikethrough effect for a list of text items. Checked checkboxes apply strikethrough styling.
Javascript
import { useState } from "react" ;
import Form from "react-bootstrap/Form" ;
function App() {
const [textItems, setTextItems] = useState([
{ text: "JavaScript" , checked: false },
{ text: "React.Js" , checked: false },
{ text: "Node.Js" , checked: false },
{ text: "HTML" , checked: false },
{ text: "CSS" , checked: false },
]);
const handleCheckboxChange = (index) => {
const updatedTextItems = [...textItems];
updatedTextItems[index].checked =
!updatedTextItems[index].checked;
setTextItems(updatedTextItems);
};
return (
<div style={{
backgroundColor: "black" ,
color: "white" ,
padding: "40px"
}}>
<Form>
<h2 style={{ color: "green" }}>
GeeksforGeeks
</h2>
{textItems.map((item, index) => (
<div key={index} style={{
display: "flex" ,
alignItems: "center"
}}>
<Form.Check
type= "checkbox"
checked={item.checked}
onChange={() => handleCheckboxChange(index)}
/>
<span style={{ marginLeft: "10px" }}>
{item.checked ?
<del>{item.text}</del>
: item.text}
</span>
</div>
))}
</Form>
</div>
);
}
export default App;
|
Steps to Run the Application:
To run the application, type the following command:
npm run dev
Open your browser and go to http://localhost:5173/
Output:
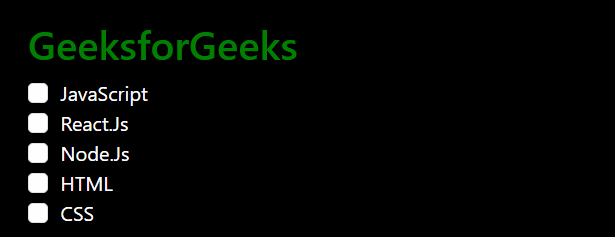
Share your thoughts in the comments
Please Login to comment...