How to strike through text when checking a Checkbox using HTML CSS and JavaScript ?
Last Updated :
20 Nov, 2023
In this article, we will learn how to apply strikethrough to a paragraph text when a checkbox is selected using HTML, CSS, and JavaScript. This is an interactive user-friendly effect and can be used on any web page. Here, we have included a code that simply creates a card with a checkbox, when you click on the checkbox, your text will immediately be strike-through.
Preview
Approach
- Create a card using HTML tags and by using the <input> element of the type checkbox to display the icon and for writing text inside the card.
- Add style through internal CSS inside <head> of the HTML file like various flex properties, box-shadow, color, etc. that give beautiful effects.
- Get the element from the id using the getElementById() method and store it in a variable similarly get the element paragraph and store it in another variable. Then use an addEventListener of type change and specify the function that is executed.
- Using the if condition check if the checkbox is checked then simply strike through the paragraph text and if the checkbox is unchecked then simply remove the strikethrough.
Example: This example describes the basic implementation of the striking through a particular paragraph text with a checkbox.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Strike through Text</ title >
< style >
#checkbox:checked,
p#textToStrike {
text-decoration: line-through;
}
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
flex-direction: column;
}
#heading {
color: green;
}
.main {
border: 2px solid green;
padding: 40px;
box-shadow: 0px 4.5px 5.5px rgba(0, 0, 0, 0.15);
border-radius: 15px;
text-align: center;
}
</ style >
</ head >
< body >
< h2 id = heading >
GeeksforGeeks
</ h2 >
< div class = "main" >
< label for = "checkbox" >
< input type = "checkbox"
id = "checkbox" >
Scratch Text
</ label >
< p id = "TextToStrike" >
Let's strike this text for fun.
</ p >
</ div >
< script >
const checkbox =
document.getElementById("checkbox");
const textToCross =
document.getElementById("TextToStrike");
checkbox.addEventListener("change",
function () {
// If checked, strike through
// the paragraph text
if (this.checked) {
textToCross.style.textDecoration =
"line-through";
}
// If unchecked, remove the strikethrough
else {
textToCross.style.textDecoration = "none";
}
});
</ script >
</ body >
</ html >
|
Output:
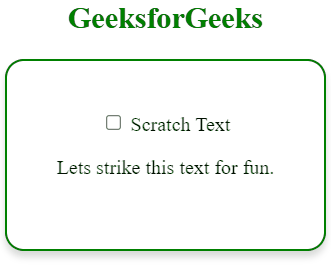
Share your thoughts in the comments
Please Login to comment...