How to Play a Small Clip from a Long Video in HTML5?
Last Updated :
21 Feb, 2024
Playing a specific clip from a longer video in HTML can enhance user experience and streamline content delivery. Sometimes, you may have a long video but only want to showcase a specific portion of it on your website. In such cases, playing a small clip from a longer video can be useful. HTML5 offers several approaches to achieve this seamlessly. Media fragments let you choose a specific part of a video by adding something extra to its web address The other approach used JavaScript to set the start and end times of the clip and control video playback accordingly.
Syntax
<video controls>
<source src="longvideo.mp4#t=START_TIME_IN_SECONDS,END_TIME_IN_SECONDS"
type="video/mp4">
Your browser does not support the video tag.
</video>
To play a small clip from a long video in HTML5 we can use media fragments. The #t=10,25 shows it will play from 10 seconds to 25 seconds. The width and height set the size of the video player. You just add #t= and the start and end times to the video’s web address. The times are written like hours, minutes, and seconds. If you only give one time, it means the start, and it plays until the end.
Example: Illustration of playing a small clip from a long video in HTML5 using Media Fragment.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Play Small Clip from Long Video</ title >
< style >
h1,
h3 {
color: green;
text-align: center;
}
.videobox {
display: flex;
justify-content: center;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >Playing a small clip from a
long video using Media Fragments
</ h3 >
< div class = "videobox" >
< video controls width = "640"
height = "360" >
< source src = "gfgvideo.mp4#t=10,25"
type = "video/mp4" >
Your browser does not support the video tag.
</ video >
</ div >
</ body >
</ html >
|
Output:
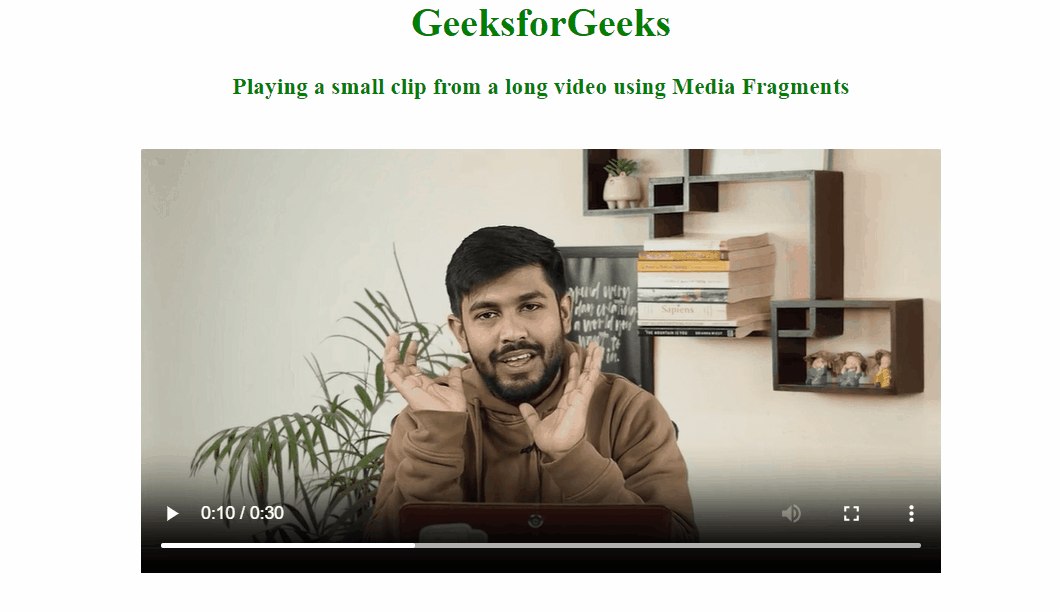
Using JavaScript
In JavaScript, we first get a reference to the video element using document.getElementById. Define clipStartTime and clipEndTime in seconds to specify the start and end times of the clip. The playClip() function checks if the start time is before the end time and if both are within the video duration. If not, it displays an error message using alert() and exits the function. We call playClip() to start playback when the page loads. We add an event listener to the video element to automatically pause the video when it reaches the end time of the clip.
Example: Illustration of playing a small clip from a long video in HTML5 using JavaScript.
Javascript
const video = document.getElementById( 'myVideo' );
const clipStartTime = 10;
const clipEndTime = 20;
function playClip() {
if (clipStartTime >= clipEndTime) {
alert( 'Error: Clip start time cannot be after end time.' );
return ;
}
if (clipStartTime < 0 || clipEndTime > video.duration) {
alert( 'Error: Clip times must be within video duration.' );
return ;
}
video.currentTime = clipStartTime;
video.play();
}
playClip();
video.addEventListener( 'timeupdate' , function () {
if (video.currentTime >= clipEndTime) {
video.pause();
}
});
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >Video Clip Player</ title >
< style >
h1,
h3 {
color: green;
text-align: center;
}
.videobox {
display: flex;
justify-content: center;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >Play a small clip from a long video
in HTML5 using JavaScript
</ h3 >
< div class = "videobox" >
< video id = "myVideo" controls
height = "300"
width = "400" >
< source src = "Video1.mp4"
type = "video/mp4" >
Your browser does not support the video tag.
</ video >
</ div >
< script src = "index.js" ></ script >
</ body >
</ html >
|
Output:
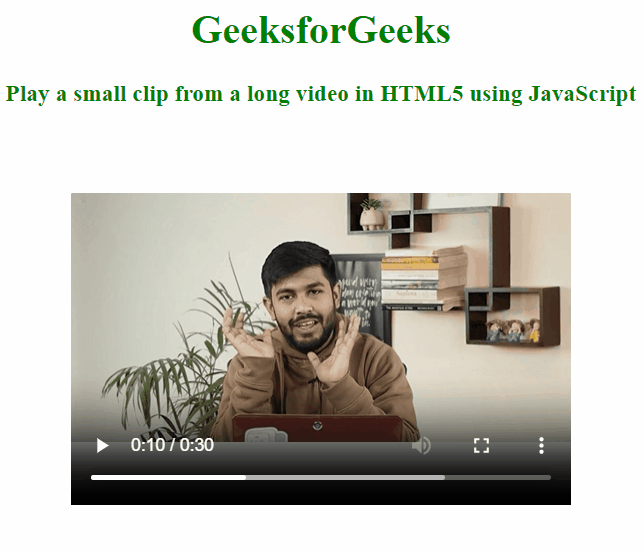
Share your thoughts in the comments
Please Login to comment...