How to display play pause and mute buttons in HTML5 ?
Last Updated :
12 Mar, 2024
To display play, pause, and mute buttons in an audio file through HTML5, we can use JavaScript methods such as “play()“, “pause()” and “.muted“. With this feature of audio display with only play, pause, and mute buttons, we’re essentially giving the user control over the playback of the audio file according to their preference.
Syntax to display Audio Buttons:
play() - It is used to start the current playing audio
pause() - It is used to pause the current playing audio
.muted - It mutes the current playing audio. To unmute, use it's negation.
Approach to Display Play, Pause, and Mute Button
- Use an audio tag to specify the path and give it an ID (such as “music” in the below example).
- After that, create 3 buttons for play, pause, and mute the audio and also specify “onClick” eventListener to each button.
- Now, take the instance of the audio file in the script through “.getElementById” and store it in a variable.
- Create three functions for different actions (play, pause, and mute) as described below, and apply “play()“, “pause()“, and “.muted” on that variable that has the audio file’s instance.
- Call these functions from individual “onClick” events of the respective buttons (play, pause, and mute).
Example: Illustration of Audio display only plays pause and mute buttons in HTML5.
HTML<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>How to Audio display only play pause
and mute buttons in HTML5
</title>
<link rel="stylesheet" href=
"https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.5.1/css/all.min.css">
<style>
button {
border: 3px solid #b1b3b4;
background-color: #287bd3;
color: white;
padding: 10px 20px;
margin: 20px;
transition: all 0.3s ease;
cursor: pointer;
outline: none;
border-radius: 5px;
}
button:hover {
background-color: #0056b3;
border-color: #afcff2;
}
button:active {
transform: scale(0.95);
}
i {
margin-left: 7px;
}
</style>
</head>
<body>
<h2>Audio display only play pause and mute buttons </h2>
<!-- audio file -->
<audio id="music">
<source src="sample.mp3" type="audio/mp3">
</audio>
<!-- button to play -->
<button onclick="playAudio()" type="button">
Play
<i class="fa-solid fa-play"></i>
</button>
<!-- button to pause -->
<button onclick="pauseAudio()" type="button">
Pause
<i class="fa-solid fa-pause"></i>
</button>
<!-- button to mute -->
<button onclick="muteAudio()" type="button">
Mute
<i class="fa-solid fa-volume-xmark"></i>
</button>
<!-- javascript code -->
<script>
// get element from html
var audioT = document.getElementById("music");
// use play() method to play music
function playAudio() {
audioT.play();
}
// use pause() method to play music
function pauseAudio() {
audioT.pause();
}
// use muted to pause music
function muteAudio() {
audioT.muted = !audioT.muted;
}
</script>
</body>
</html>
Output:
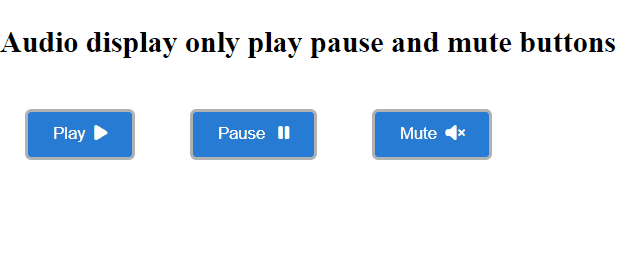
Output
Share your thoughts in the comments
Please Login to comment...