How to make Sound only Play Once in HTML5 Audio ?
Last Updated :
20 Mar, 2024
In HTML5, we can validate the audio playing by using the proper event handling and control manipulation. In this article, we will explore two different approaches to create sound that only plays once in HTML5 Audio.
Using JavaScript Event Listeners
In this approach, we are using JavaScript event listeners to handle the ‘play‘ event for the HTML5 audio element. The code makes sure that the audio plays only once by pausing the playback and displaying an alert if it has already been played, and resets the play status when the audio has ended.
Syntax:
element.addEventListener(event, listener, useCapture);
Example: The below example uses the JavaScript Event Listeners to create Sound, which only plays once in HTML5 Audio.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
</head>
<body>
<h1 style="color: green;">
GeeksforGeeks
</h1>
<h3>Using JavaScript Event Listeners</h3>
<audio id="audioPlayer" controls>
<source
src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240228225306/twirling-intime-lenovo-k8-note-alarm-tone-41440.mp3"
type="audio/mp3">
Your browser does not support the audio element.
</audio>
<script>
const audioPlayer =
document.getElementById('audioPlayer');
let hasPlayed = false;
audioPlayer.addEventListener('play', () => {
if (hasPlayed) {
audioPlayer.pause();
alert('Audio can only be played once.');
} else {
hasPlayed = true;
}
});
audioPlayer.addEventListener('ended', () => {
hasPlayed = true;
});
</script>
</body>
</html>
Output:

Using onended Attribute
In this approach, we are using the onended attribute within the <audio> element to specify a JavaScript function, in this case, the disableControls() function, that will be executed when the audio playback reaches its end.
Syntax:
<element onended="myScript">
Example: The below example uses the onended Attribute to create Sound only plays once in HTML5 Audio.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
</head>
<body>
<h1 style="color: green;">
GeeksforGeeks
</h1>
<h3>Using onended Attribute</h3>
<audio id="myAudio"
controls autoplay preload="auto"
onended="disableControls()">
<source src="
https://media.geeksforgeeks.org/wp-content/uploads/20240228225306/twirling-intime-lenovo-k8-note-alarm-tone-41440.mp3"
type="audio/mp3">
Your browser does not support the audio tag.
</audio>
<p id="message"
style="display: none;">
Audio can be played only once.
</p>
<script>
let audioPlayed = false;
function disableControls() {
let audio =
document.getElementById('myAudio');
let message =
document.getElementById('message');
if (!audioPlayed) {
audio.controls = false;
audioPlayed = true;
message.style.display = 'block';
}
audio.removeEventListener(
'ended', disableControls
);
}
</script>
</body>
</html>
Output:
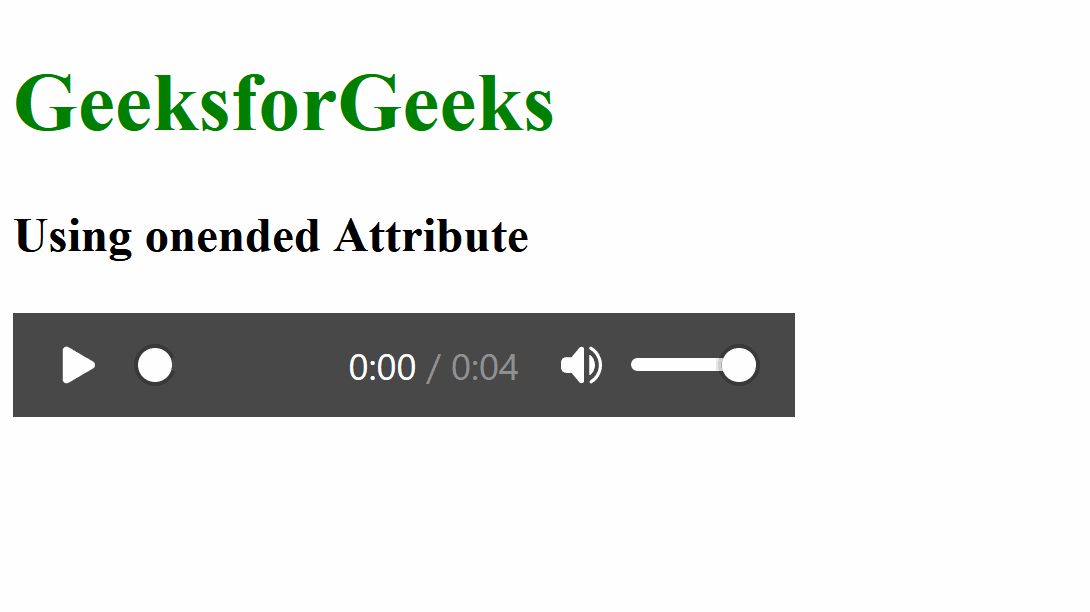
Share your thoughts in the comments
Please Login to comment...