How to open a link in a new Tab in NextJS?
Last Updated :
17 Apr, 2024
Opening a link in a new tab in Next.js consists of using either the target=”_blank” attribute in an anchor (<a>) tag or using Next.js’s Link component with the passHref prop to ensure proper handling of routing while opening the link in a new tab. In this article, we will explore both these approaches with complete implementation.
Link Behavior in Next.js
Next.js provides a versatile <Link>
component for client-side navigation within your application. By default, when a user clicks on a link rendered using <Link>
, Next.js handles the navigation without triggering a full page reload. This behavior is optimized for single-page applications (SPAs) and enhances performance by efficiently updating the DOM without reloading the entire page.
Steps to Create the Next App
Step 1: Set up React Project using the Command:
npx create-next-app new-tab
Step 2: Navigate to the Project folder using:
cd new-tab
Project Structure:
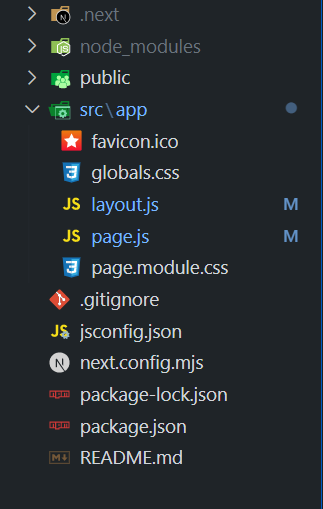
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.2.1"
}
Approach 1: Using Anchor Tag with target=”_blank”
In this approach, we’re using an anchor (<a>) tag with the target=”_blank” attribute in Next.js. This combination ensures that when the link is clicked, the destination page opens in a new browser tab, providing a seamless user experience while browsing.
Example: The below example uses Anchor Tag with target=”_blank” to open a link in a new Tab in NextJS.
JavaScript
// src/app/page.js
"use client";
import React from 'react';
const NewTabLink = () => (
<>
<h1>Using Anchor Tag with target="_blank"</h1>
<a href="https://geeksforgeeks.org"
target="_blank" rel="noopener noreferrer">
Open in New Tab
</a>
</>
);
export default NewTabLink;
Step to run the application: Now run the application with the below command:
npm run dev
Output:
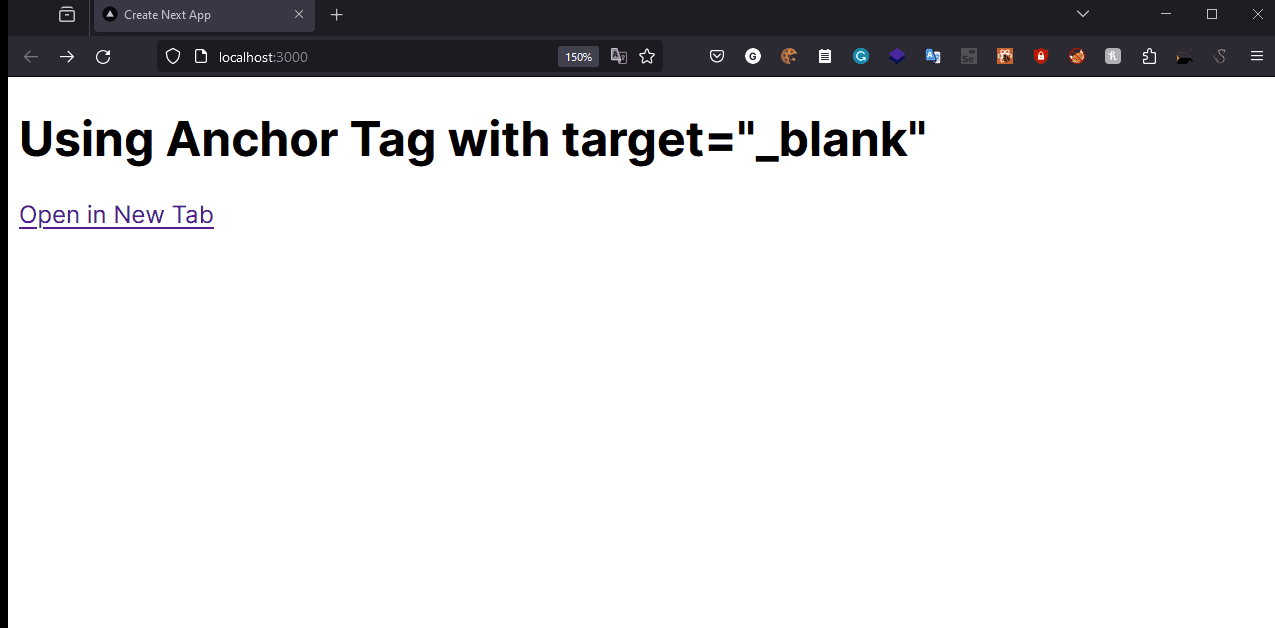
Approach 2: Using Link Component with passHref Prop
In this approach, we are using Next.js’s Link component with the passHref prop and legacyBehavior to generate an anchor tag (<a>) that opens the link in a new tab when clicked. This method ensures proper handling of routing within Next.js while opening the link in a new tab.
Example: The below example uses Link Component with passHref Prop to open a link in a new Tab in NextJS.
JavaScript
//src/app/page.js
"use client";
import React from 'react';
import Link from 'next/link';
const NewTabLink = () => (
<>
<h1>Using Link Component with passHref Prop</h1>
<Link href="https://geeksforgeeks.org" passHref legacyBehavior>
<a target="_blank">
Open in New Tab
</a>
</Link>
</>
);
export default NewTabLink;
Step to run the application: Now run the application with the below command:
npm run dev
Output:
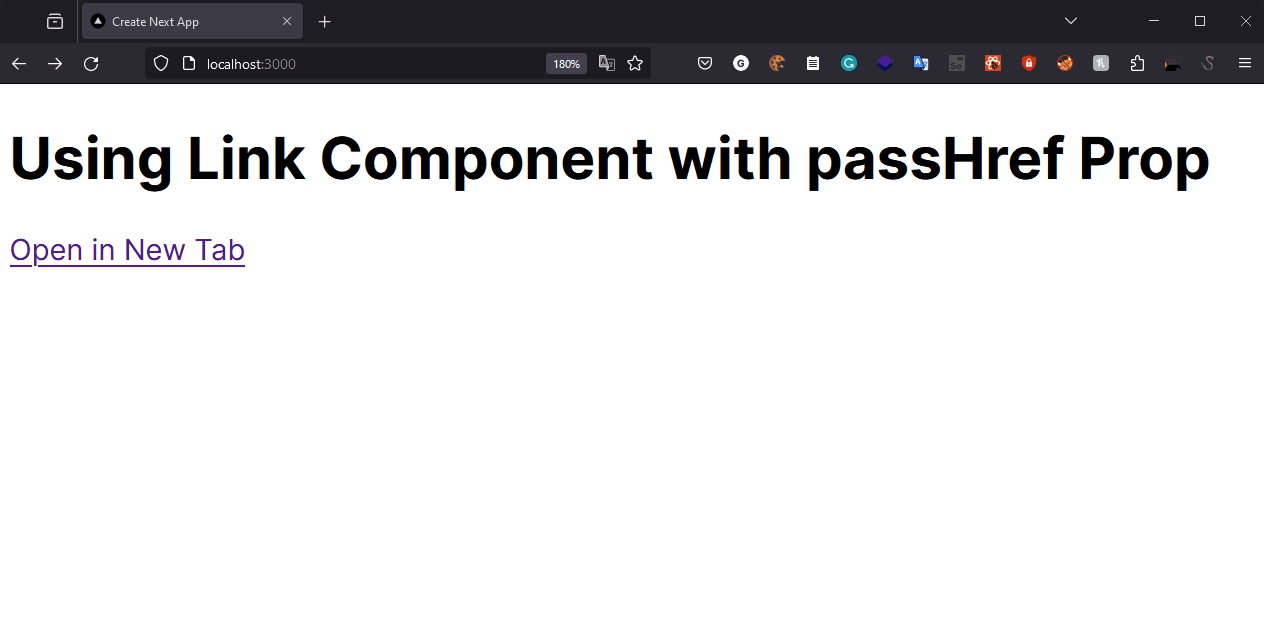
Share your thoughts in the comments
Please Login to comment...