Difference between NextJS Link vs useRouter in Navigating
Last Updated :
30 Apr, 2024
NextJS is a React framework that is used to build full-stack web applications. It is used both for front-end as well as back-end. It comes with a powerful set of features to simplify the development of React applications. One of its features is navigation. Navigation is crucial for providing users with seamless browsing experiences.
Next.js provides two primary methods Link component and the useRouter hook for navigation. In this article, we will learn about the difference between the Link component and useRouter hook.
Link Component
Next.js provides a Link component. It is used to create navigation links between pages in a Next.js application. It allows for client-side navigation, which means that when a user clicks on a link created by the Link component, the content of the target page is fetched and rendered without a full page reload.
Syntax:
import Link from 'next/link';
<Link href="/target-page">
<a>Go to Target Page</a>
</Link>
Steps to Create Next.js Application
Step 1: Create a NextJS application using the following command.
npx create-next-app@latest gfg
Step 2: It will ask you some questions, so choose the following.
√ Would you like to use TypeScript? ... No
√ Would you like to use ESLint? ... Yes
√ Would you like to use Tailwind CSS? ... No
√ Would you like to use `src/` directory? ... Yes
√ Would you like to use App Router? ... Yes
√ Would you like to customize the default import alias (@/*)? ... No
Step 3: After creating your project folder i.e. gfg, move to it using the following command.
cd gfg
Example:
JavaScript
//File path : ./src/app/page.js
import Link from "next/link";
export default function Home() {
return (
<>
<h1>Home Page</h1>
<Link href='/about'>About Page</Link>
</>
);
}
JavaScript
//File path : ./src/app/about/page.js
import Link from "next/link";
export default function Page() {
return (
<>
<h1>About page</h1>
<Link href='/'>Home Page</Link>
</>
)
}
Output:
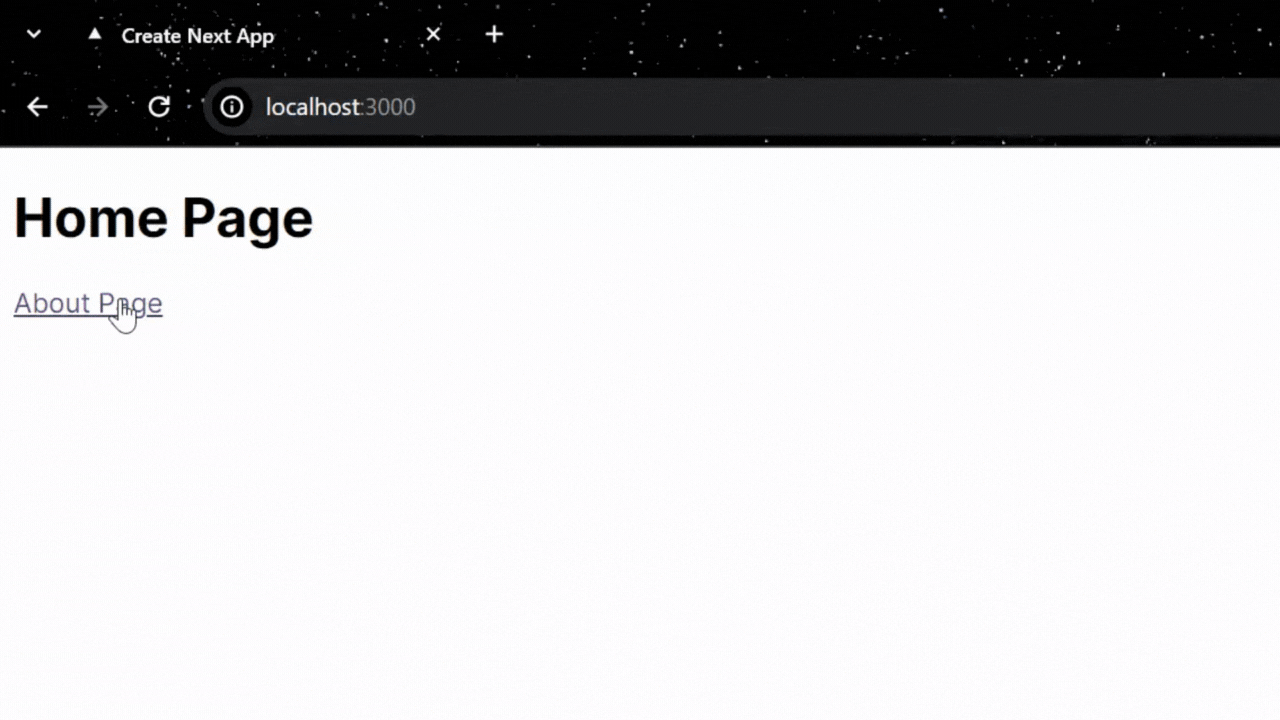
useRouter hook
Next.js provides a useRouter hook to access the router object and provides various methods to perform a operations. If you have selected Pages Router, while creating app then you have to import useRouter hook from next/router and you can access the router object and use it’s methods.
If you have selected App Router, while creating app then you have to import useRouter hook from next/navigation and to access different information such as pathname, query then you have to use usePathname, useSearchParams to get those values.
Here, we are using App Router.
Syntax:
'use client'
import { useRouter } from 'next/navigation'
const router = useRouter()
router.push('/path')
Example:
JavaScript
//File path : ./src/app/page.js
'use client'
import { useRouter } from "next/navigation";
export default function Home() {
const router = useRouter()
return (
<>
<h1>Home Page</h1>
<li onClick={()=>router.push('/about')}>About Page</li>
</>
);
}
JavaScript
//File path : ./src/app/about/page.js
'use client';
import { useRouter } from "next/navigation";
export default function Page() {
const router = useRouter();
return (
<>
<h1>About page</h1>
<li onClick={() => router.push('/')}>Home Page</li>
</>
)
}
Output:
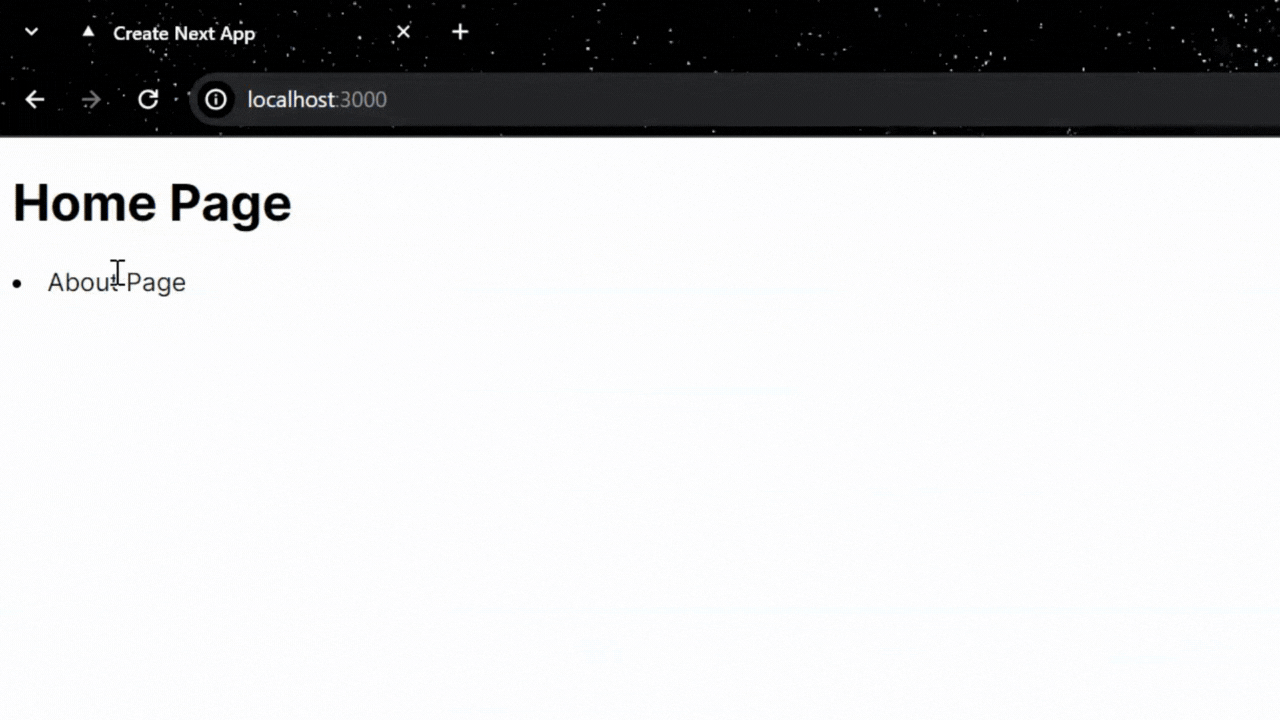
Differences between Link and useRouter
Link Component
| useRouter Hook
|
---|
It is component based.
| It is hook based.
|
We can directly import the component and use it within JSX.
| We have to import the hook and by accessing it’s method, we can perform a navigation.
|
We can’t access the Route object Information.
| We can access Route object information and use all it’s method.
|
It supports dynamic routing and query parameters.
| It also supports dynamic routing and query parameters.
|
It is used for basic routing.
| It is used for complex navigation logic and conditional navigation.
|
Share your thoughts in the comments
Please Login to comment...