How to Get The Current URL Domain in Next.js ?
Last Updated :
17 Apr, 2024
In Next.js, accessing the current URL domain can be achieved using various methods. In this article, we’ll explore different approaches and best practices for obtaining the current URL domain in Next.js applications.
Why Accessing the Current URL Domain is Important ?
Obtaining the current URL domain is useful for various purposes in web development, such as:
- Dynamic Content: Displaying content based on the current domain or subdomain.
- Analytics and Tracking: Tracking user behavior or performing analytics based on the domain.
- Localization: Determining the user’s location or language preference based on the domain.
- SEO (Search Engine Optimization): Customizing meta tags or content based on the domain for better search engine visibility.
Steps to Create the Next.js Application
Step 1: Set up React Project using the Command:
npx create-next-app url-domain
Step 2: Navigate to the Project folder using:
cd url-domain
Project Structure:
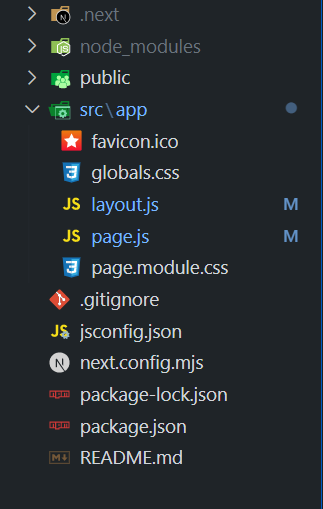
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.2.1"
}
Approach 1: Using window.location
In this approach, we are using the useState hook in a Next.js page component (HomePage) to manage the current URL domain state. When the button is clicked, the handleGetDomain function updates the state with window.location.origin, dynamically displaying the current URL domain in the component.
Syntax:
window.location.origin
Example: The below example uses window.location to Get The Current URL Domain in NextJS.
JavaScript
//src/app/page.js
"use client";
import { useState } from 'react';
const HomePage = () => {
const [currentDomain, setCurrentDomain] = useState('');
const handleGetDomain = () => {
setCurrentDomain(window.location.origin);
};
return (
<div>
<h1>Using window.location</h1>
<button onClick={handleGetDomain}>Get Current Domain</button>
{currentDomain && <h2>Current URL Domain: {currentDomain}</h2>}
</div>
);
};
export default HomePage;
Step to run the application: Now run the application with the below command:
npm run dev
Output:
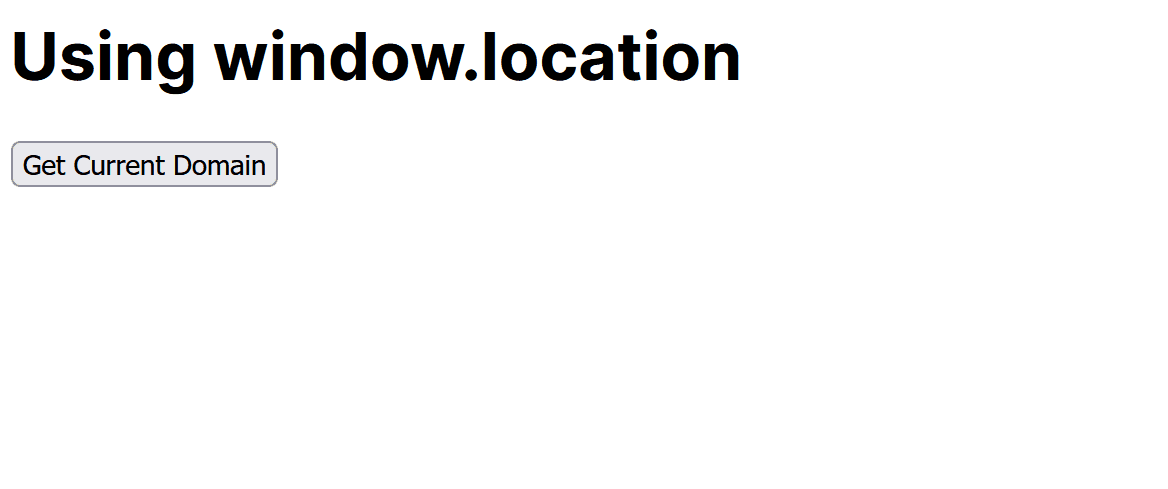
Approach 2: Using document.location
In this approach, we are using the useState hook in a Next.js page component (HomePage) to manage states for the current URL’s hostname, protocol, port, and the full URL itself (currHostName, currProtocol, currPort, and currURL). By invoking document.location and window.location properties within the handleGetURLDetails function, we dynamically fetch and display the corresponding URL details when the “Get URL Details” button is clicked.
Syntax:
window.location.href
Example: The below example uses document.location to Get The Current URL Domain in NextJS.
JavaScript
//src/app/page.js
"use client";
import React, { useState } from 'react';
const HomePage = () => {
const [currHostName, setCurrentHostname] = useState('');
const [currProtocol, setCurrentProtocol] = useState('');
const [currPort, setCurrentPort] = useState('');
const [currURL, setCurrentURL] = useState('');
const handleGetURLDetails = () => {
setCurrentHostname(document.location.hostname);
setCurrentProtocol(document.location.protocol);
setCurrentPort(document.location.port || 'Default');
setCurrentURL(window.location.href);
};
return (
<div>
<h1>Using document.location</h1>
<button onClick={handleGetURLDetails}>Get URL Details</button>
<div>
<p>Current URL: {currURL}</p>
<p>Hostname: {currHostName}</p>
<p>Protocol: {currProtocol}</p>
<p>Port: {currPort}</p>
</div>
</div>
);
};
export default HomePage;
Step to run the application: Now run the application with the below command:
npm run dev
Output:
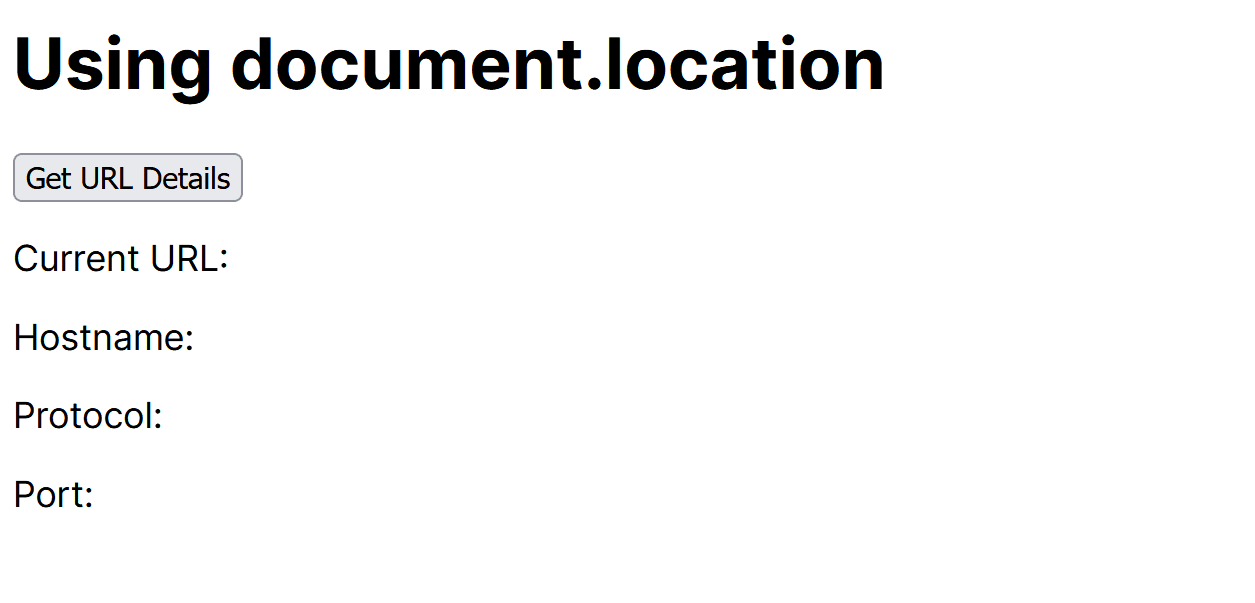
Share your thoughts in the comments
Please Login to comment...