How to Make a Loading Bar for an HTML5 Audio Element ?
Last Updated :
20 Mar, 2024
A Loading bar provide a visual representation of audio loading progress in the application. It tells how much of the audio file is covered and how much of it is left to be listened to.
Below are the approaches to make a loading bar for an HTML audio element:
Using audio tag
In HTML5, we have built-in media support, using the audio tag we can embed media straight into any HTML document with limited code. It comes with built-in browser controls as well if you specify. To implement an audio progress bar in HTML5, you can use the <audio> element.
Syntax:
<audio controls>
<source src="audio_file.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
Example: This example shows the implementation of audio tag in HTML.
HTML
<!DOCTYPE html>
<html>
<head>
<title>GFG</title>
</head>
<body>
<h1 style="color:green; text-align: center">
GeeksforGeeks
</h1>
<audio controls style="display: flex; margin: auto;">
<source src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240308211308/town-10169.mp3"
type="audio/mpeg" />
Your browser does not support the audio element.
</audio>
</body>
</html>
Output:
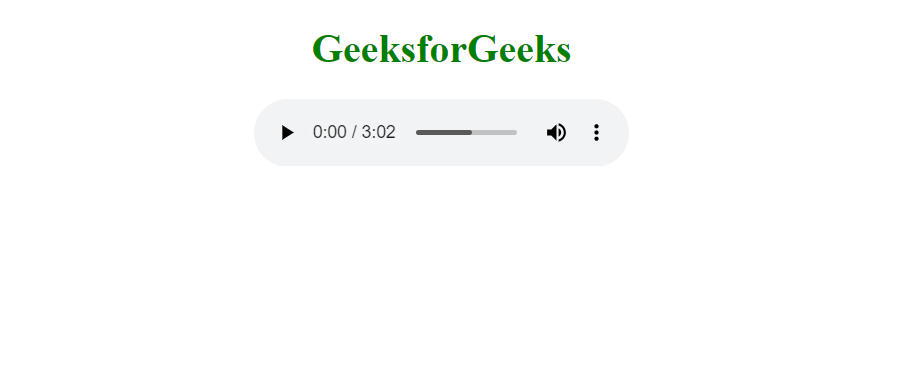
Using custom CSS and JavaScript
Also, we can create a custom loading bar for an HTML5 audio element by integrating JavaScript with HTML and CSS to provide a visual representation of audio loading progress. This progress bar is styled using CSS to ensure it aligns with the design of the web page.
Example: This example shows the implementation of custom CSS & JavaScript to create a Loading Bar.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Custom Audio Player with Loading Bar and Volume Control</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1 style="color:green; text-align: center">
GeeksforGeeks
</h1>
<div class="audio-container">
<audio id="myAudio">
<source src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240308211308/town-10169.mp3"
type="audio/mpeg" />
Your browser does not support the audio element.
</audio>
<div class="controls">
<button id="playPauseBtn" class="play">
Play
</button>
<div class="progress-container">
<div class="progress-bar" id="progressBar"></div>
</div>
</div>
<div class="volume-container">
<svg class="w-6 h-6 text-gray-800 dark:text-white"
aria-hidden="true" xmlns="http://www.w3.org/2000/svg"
fill="currentColor" viewBox="0 0 24 24">
<path
d="M13 6a2 2 0 0 0-3.3-1.5l-4 3.4H4a2 2
0 0 0-2 2V14c0 1.2.9 2 2 2h1.6l4.1
3.5A2 2 0 0 0 13 18V6Z" />
<path fill-rule="evenodd"
d="M14.8 7.7a1 1 0 0 1 1.4 0 6.1 6.1 0
0 1 0 8.6 1 1 0 0 1-1.3 0 1 1 0 0 1
0-1.5 4 4 0 0 0-.1-5.7 1 1 0 0 1 0-1.4Z"
clip-rule="evenodd" />
<path fill-rule="evenodd"
d="M17.7 4.8a1 1 0 0 1 1.4 0 10.2 10.2
0 0 1 0 14.4 1 1 0 0 1-1.4 0 1 1 0 0
1 0-1.4 8.2 8.2 0 0 0 0-11.6 1 1 0 0 1 0-1.4Z"
clip-rule="evenodd" />
</svg>
<input type="range" min="0" max="1"
step="0.1" value="1" id="volumeControl" />
</div>
</div>
<script src="script.js"></script>
</body>
</html>
CSS
.audio-container {
position: relative;
margin-top: 20px;
}
.controls {
display: flex;
align-items: center;
}
.progress-container {
height: 7px;
margin: 0 10px;
width: 80%;
background-color: #A8A8A8;
}
.volume-container {
/* flex: 1; */
display: flex;
height: 7px;
margin-top: 15px;
width: 10%;
align-items: center;
}
.volume-container>svg {
height: 2rem;
}
.progress-bar,
input[type="range"] {
width: 100%;
height: 100%;
}
.progress-bar {
background-color: #4caf50;
}
button {
padding: 8px 12px;
font-size: 16px;
cursor: pointer;
}
Javascript
const audio =
document.getElementById('myAudio');
const playPauseBtn =
document.getElementById('playPauseBtn');
const progressBar =
document.getElementById('progressBar');
const volumeControl =
document.getElementById('volumeControl');
let isPlaying = false;
playPauseBtn.addEventListener(
'click', togglePlay
);
volumeControl.addEventListener(
'input', adjustVolume
);
audio.addEventListener(
'timeupdate', updateProgress
);
function togglePlay() {
if (isPlaying) {
audio.pause();
playPauseBtn.textContent = 'Play';
} else {
audio.play();
playPauseBtn.textContent = 'Pause';
}
isPlaying = !isPlaying;
}
function adjustVolume() {
audio.volume = volumeControl.value;
}
function updateProgress() {
const duration = audio.duration;
const currentTime = audio.currentTime;
const progress =
(currentTime / duration) * 100;
progressBar.style.width = progress + '%';
}
Output:
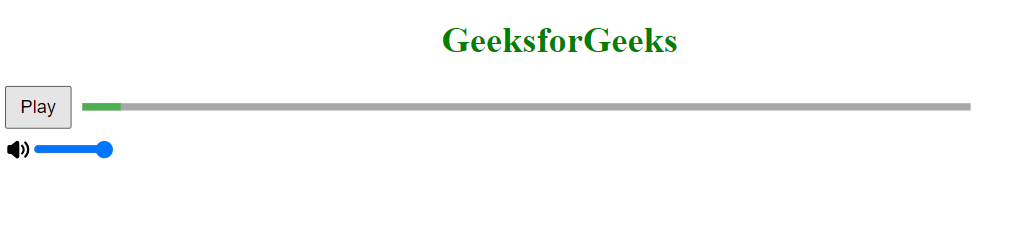
Share your thoughts in the comments
Please Login to comment...